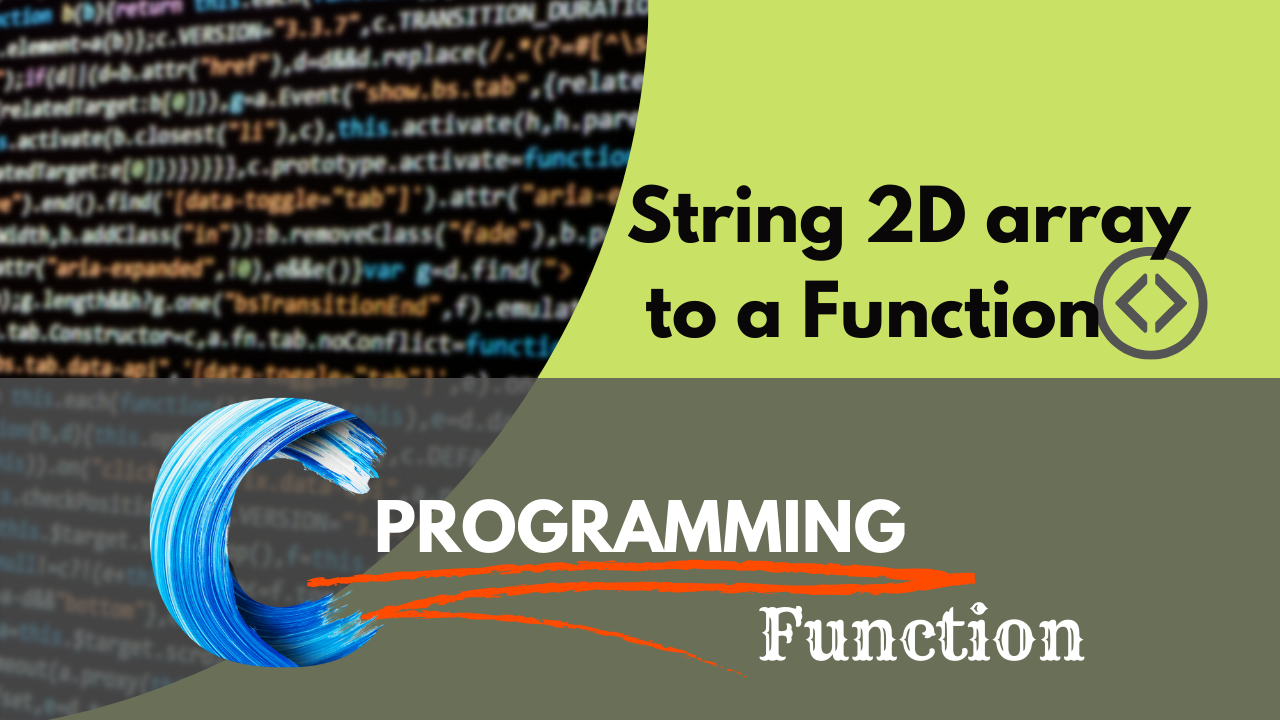
String 2D array to a Function in c
what does mean String 2D array to a Function in c
Passing a String 2D array
to a function in C means that you are sending a 2-dimensional array of strings as an argument to a function in your code. A 2D string array is an array of arrays where each element in the array is a string itself.
When passing a 2D string array to a function, you are essentially passing a pointer to the first element of the array, which is also an array of characters representing a string. The pointer points to the memory address of the first character of the first string.
In order for the receiving function to correctly handle the 2D string array, it needs to know the size of the array (i.e. the number of rows and columns), so that it can correctly access each element of the array. Additionally, if the strings in the array are not of a fixed size, you need to specify the maximum length of the strings, so that the function knows how many characters to read for each string.
When working with strings in C, remember that strings are represented as arrays of characters, terminated with a null character ('\0').
When passing string arrays to functions, you should use functions like strcpy()
or strncpy()
to safely copy string values, and printf()
with the %s format specifier to print out strings.print out each string in the array.
To pass a string 2D array to a function in C using the second method, you need to do the following:
- Declare the function parameter to be an array of pointers to strings.
- Get the address of each row of the 2D array.
- Pass the array of pointers to the function as an argument.
write a C program to Passing a 2D String Array to a Function in C
#include<stdio.h>
void print_array(char *array[10]) {
for (int i = 0; i < 10; i++) {
printf("%s\n", array[i]);
}
}
int main() {
char array[10][10] = {
"Hello",
"World",
"!"
};
// Get the address of each row of the array
char *array_ptrs[10];
for (int i = 0; i < 10; i++) {
array_ptrs[i] = array[i];
}
print_array(array_ptrs);
return 0;
}
Output:
Hello
World
!
Note that the second method is more efficient than the first method, but it is also more complex. This is because you need to get the address of each row of the 2D array before you can pass it to the function.
Further Reading:
2D Dimensional Array in c programming
Write a C Program to reverse 2D array Elements
Integer 2D array Passing to function in c
String 2D array to a Function in c
Write a C program to short 2D array using bubble sort
c program to allocate dynamic memory for 2D array integers
Using Arrays of Pointers to Represent Two-Dimensional Arrays
How to Calculate of sub element of 2D array and How to print it in c
Write a C Program to scan 5 Elements and print on the screen using 2D array
Assingement of 2D array in c
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [[email protected]].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!