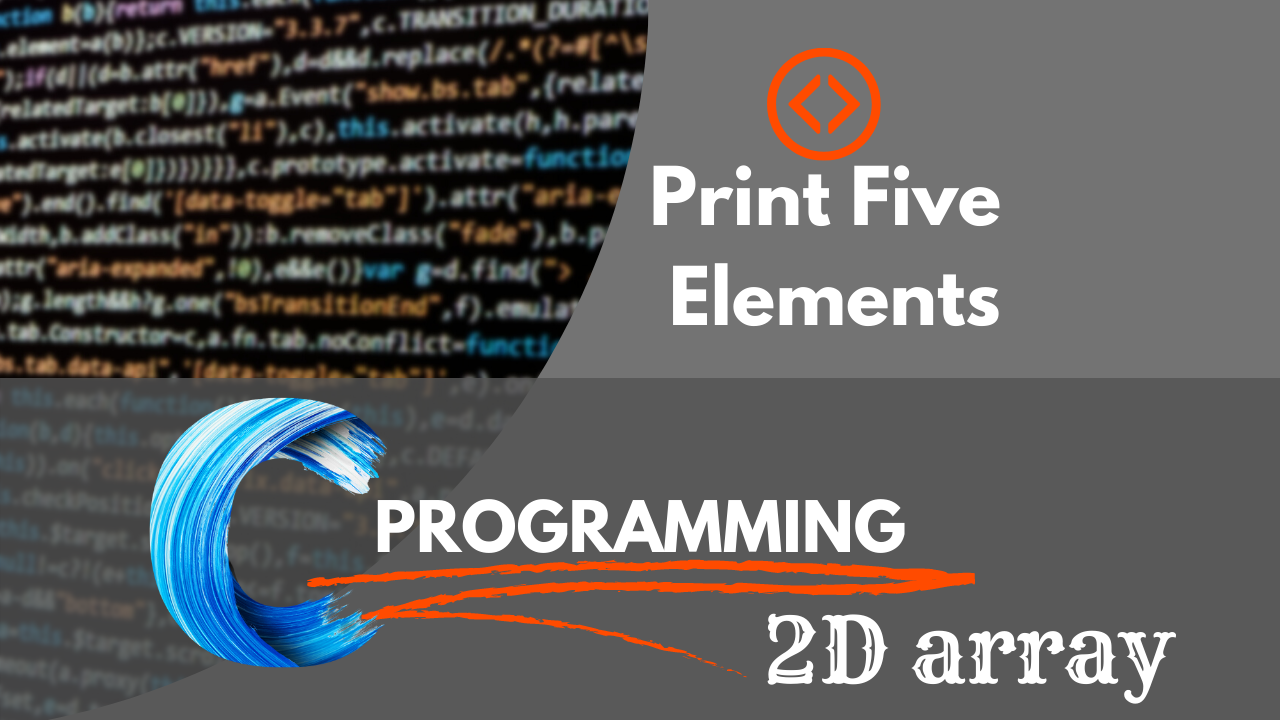
Write a program to scan 5 Elements and print on the screen using 2D array in c
2D Array Program in C: Scanning and Printing 5 Elements
To write a program to scan 5
elements and print them on the screen using a 2D
array in C, you can use the following steps:
- Declare a
2D
array with1
row and5
columns. - Use a for loop to scan the
5
elements from the user and store them in the2D
array. - Use another for loop to print the elements of the
2D
array on the screen.
Here is an example of a complete program:
C programming
#include<stdio.h>
int main() {
// Declare a 2D array with 1 row and 5 columns
int array[1][5];
// Scan the 5 elements from the user and store them in the 2D array
for (int i = 0; i < 5; i++) {
printf("Enter element %d: ", i + 1);
scanf("%d", &array[0][i]);
}
// Print the elements of the 2D array on the screen
for (int i = 0; i < 5; i++) {
printf("%d ", array[0][i]);
}
printf("\n");
return 0;
}
Output:
Enter element 1: 1
Enter element 2: 2
Enter element 3: 3
Enter element 4: 4
Enter element 5: 5
Further Reading:
2D Dimensional Array in c programming
Write a C Program to reverse 2D array Elements
Integer 2D array Passing to function in c
String 2D array to a Function in c
Write a C program to short 2D array using bubble sort
c program to allocate dynamic memory for 2D array integers
Using Arrays of Pointers to Represent Two-Dimensional Arrays
How to Calculate of sub element of 2D array and How to print it in c
Write a C Program to scan 5 Elements and print on the screen using 2D array
Assingement of 2D array in c
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!