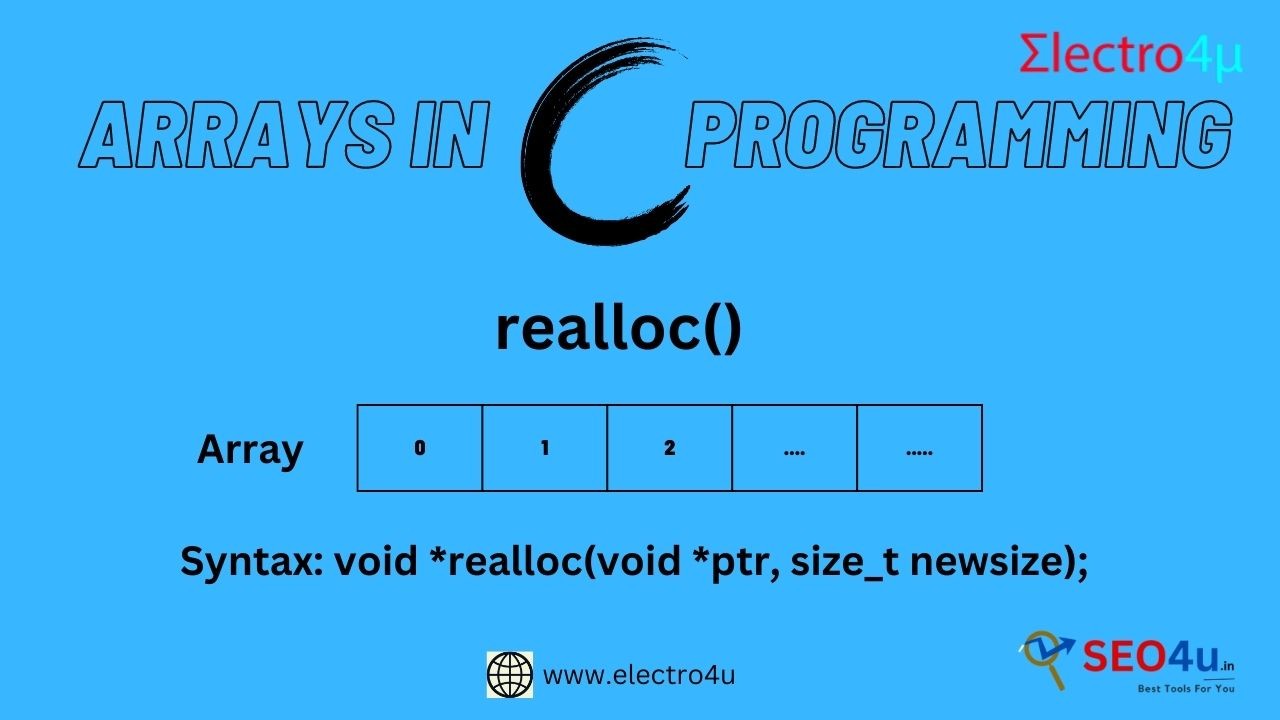
realloc() to dynamically increase size of an already allocated array
Using realloc() Function to Dynamically Increase the Size of an Already Allocated Array in C Programming Language
In C programming language, the realloc() function can be used to dynamically increase the size of an already allocated array. Here's how to use realloc() function:
-
Declare a pointer variable to the array:
int *arr;
-
Allocate memory to the array using malloc() or calloc() function:
arr = (int *)malloc(sizeof(int) * n);
where n is the initial size of the array.
-
Use the array as needed.
-
If you need to increase the size of the array, use realloc() function:
arr = (int *)realloc(arr, sizeof(int) * (n + m));
where m is the additional number of elements to be added to the array.
-
Use the expanded array as needed.
-
When finished using the array, free the memory using free() function:
free(arr);
Note that realloc() function may move the allocated memory block to a new location, so it's important to update the pointer variable accordingly.
In conclusion, realloc() function in C programming language can be used to dynamically increase the size of an already allocated array, making it a useful tool for managing memory efficiently. Understanding the syntax and practical applications of this function can help programmers write more flexible and efficient code.