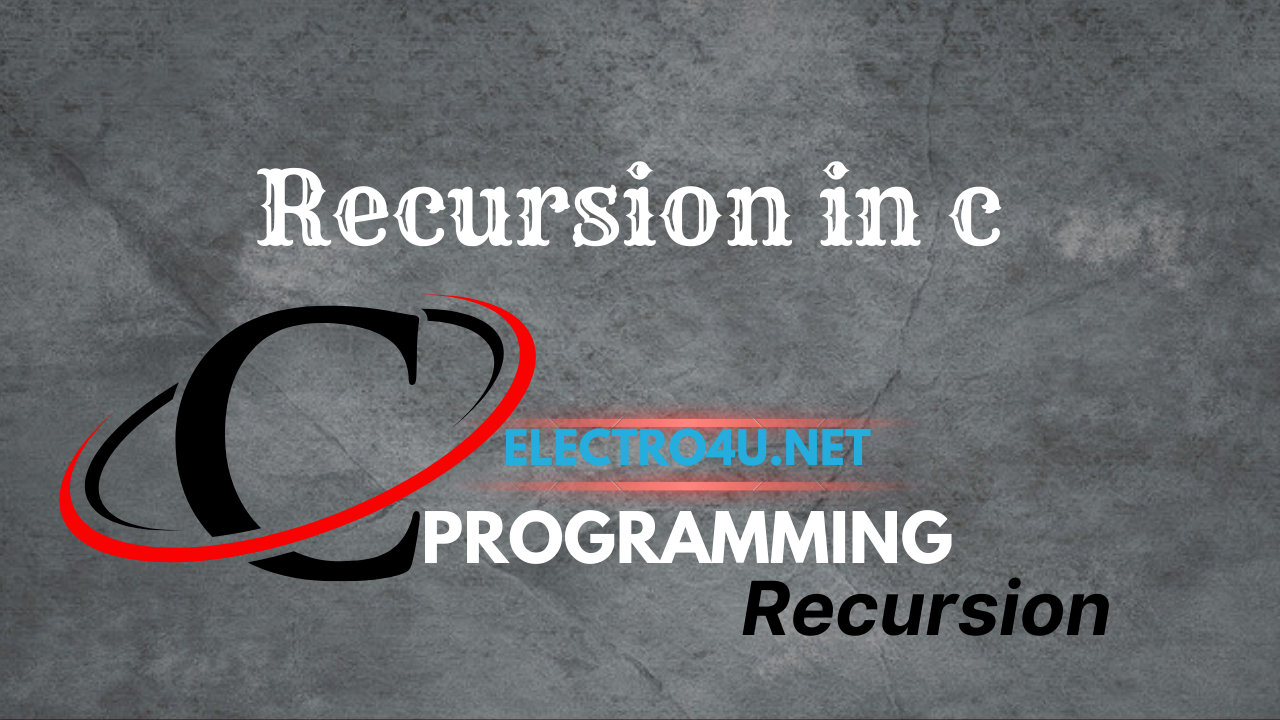
Recursion in c
what is a recursion function?
Recursion is a programming technique in which a function calls itself repeatedly until a certain condition is met. In C programming language, recursion is implemented using a function that calls itself with different arguments until a base condition is reached.
Recursion in one function definition, if the same function gets called such function is called a recursive function.
Recursion Function Implementation: For a problem to be written in recursive, two conditions must be satisfied.
- it should be possible to express the problem in a recursive form
- the problem statement must include a stopping condition
Things to be taken care of while writing a recursive function
- The recursive function acts as a loop, so avoid loops in recursive functions.
- when writing recursive functions are must have a conditional statement, such as an if somewhere to force the function to return without the recursive call being executed. if you don't the function will never return once you call it omitting the conditional statement is a standard error when writing a recursive function.
- if the recursive function returns a value, then the proper return statement should be there for both the if and else parts.
- when we are designing the recursive function maximum write the code in the if and else parts.
Here is an example of a recursive function that calculates the factorial of a number
#include <stdio.h>
int factorial(int n)
{
if(n == 0) // base condition
return 1;
else
return n * factorial(n-1); // recursive call
}
int main()
{
int n = 5;
printf("Factorial of %d is %d", n, factorial(n));
return 0;
}
Attention: In this example, the function factorial() calculates the factorial of a number n using recursion. The base condition is n == 0, which means that when the value of n reaches 0, the function stops calling itself and returns 1. Otherwise, the function calls itself with n-1 as the argument and multiplies the result with n.
Recursion can be a powerful tool in programming, but it should be used with care to avoid stack overflow and other issues that can arise from excessive recursion.