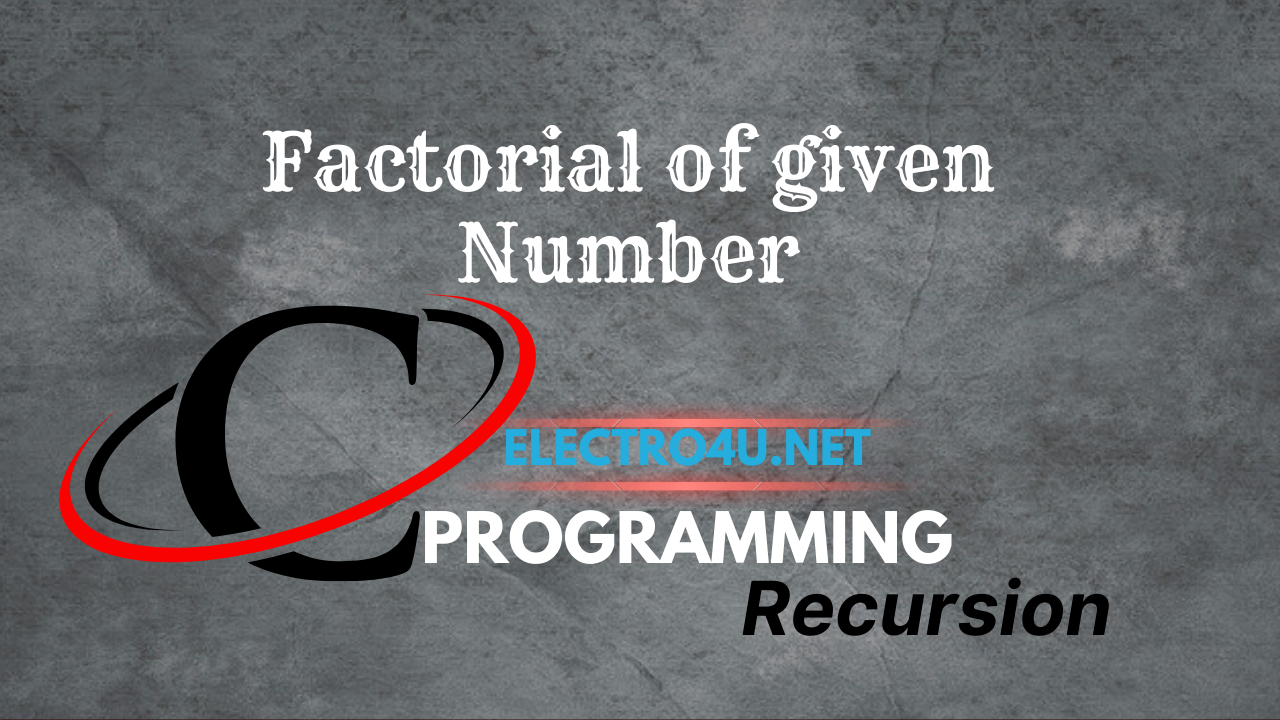
Design a recursive Function For Finding The Factorial of given Number in c
Recursive Factorial Function in C
In this session how to Design a recursive function for finding the factorial of a given number in C, means creating a function that calculates the factorial of a given number using recursion in the C programming language.
The function should take an integer as an argument, and recursively calculate the factorial of that integer until a base case is reached. The base case is typically when the integer is 0 or 1, and the factorial value is 1.
The recursive function should return the factorial value of the integer, which is the product of all the positive integers up to and including the integer. The design of the function should ensure that it works for any positive integer that is within the range of representable integers in C.
Recursive function for finding the factorial of a given number in C:
long factorial(int n) {
if (n == 0) {
return 1;
} else {
return n * factorial(n - 1);
}
}
This function works by recursively calling itself to calculate the factorial of the given number. The base case is when the given number is 0, in which case the factorial is 1. Otherwise, the factorial is calculated by multiplying the given number by the factorial of the given number minus 1.
how to use the factorial() function:
int main() {
int n;
long factorialResult;
printf("Enter a number to find the factorial of: ");
scanf("%d", &n);
factorialResult = factorial(n);
printf("The factorial of %d is %ld.\n", n, factorialResult);
return 0;
}
Output:
Enter a number to find the factorial of: 5
The factorial of 5 is 120.
Further Reading:
Calculating Factorials: A Program to Find Factorial of a Number
Design a function to finding the factorial of a given number in c
Design a recursive Function For Finding The Factorial of given Number in c
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [[email protected]].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!