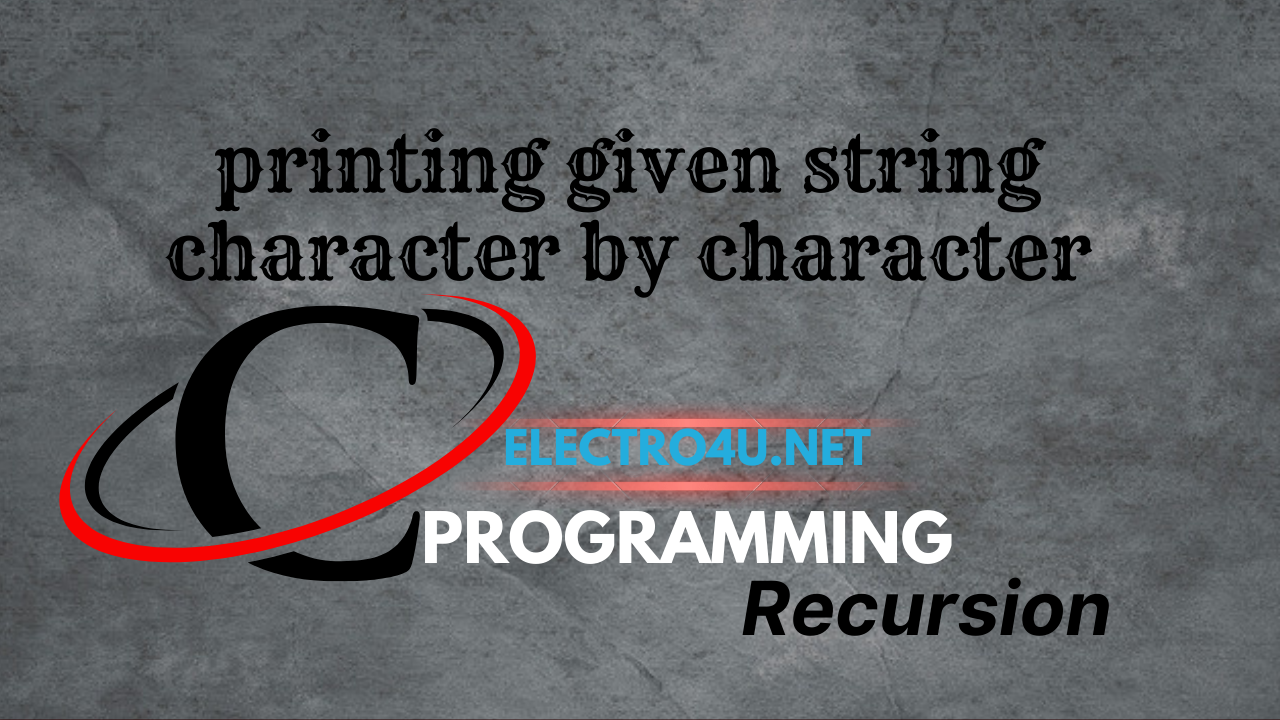
Design a recursive function for printing given string character by character
what does mean Design a recursive function for printing a given string character by character
Design a recursive function for printing a given string character by character" means to create a function that takes a string as input and recursively prints each character of the string one by one to the console in the C programming language.
The function should take a string as an argument, and recursively print each character of the string until a base case is reached. The base case is typically when the function reaches the end of the string (i.e. the null terminator character '\0'), at which point the recursion should stop.
The recursive function should print the current character to the console, and then make a recursive call to the same function with the next character of the string as an argument. The design of the function should ensure that it works for any string of characters that is within the range of representable strings in C
#include <stdio.h>
void printString(char str[]) {
if (*str == '\0') { // base case: end of string reached
return;
}
printf("%c", *str); // print current character
printString(str + 1); // recursive call with the next character
}
int main() {
char str[] = "Hello, World!";
printString(str);
return 0;
}
In this example, the printString function takes a character array str as an argument and prints each character of the string using recursion.
The base case is when the current character is the null terminator character '\0', which indicates the end of the string. In this case, the function simply returns without doing anything.
The recursive case is when the current character is not the null terminator character. The function prints the current character using printf, and then makes a recursive call to printString with the next character of the string (str + 1) as the argument.
Finally, in the main function, a test string is declared and passed to the printString function. This causes each character of the string to be printed to the console.
second method