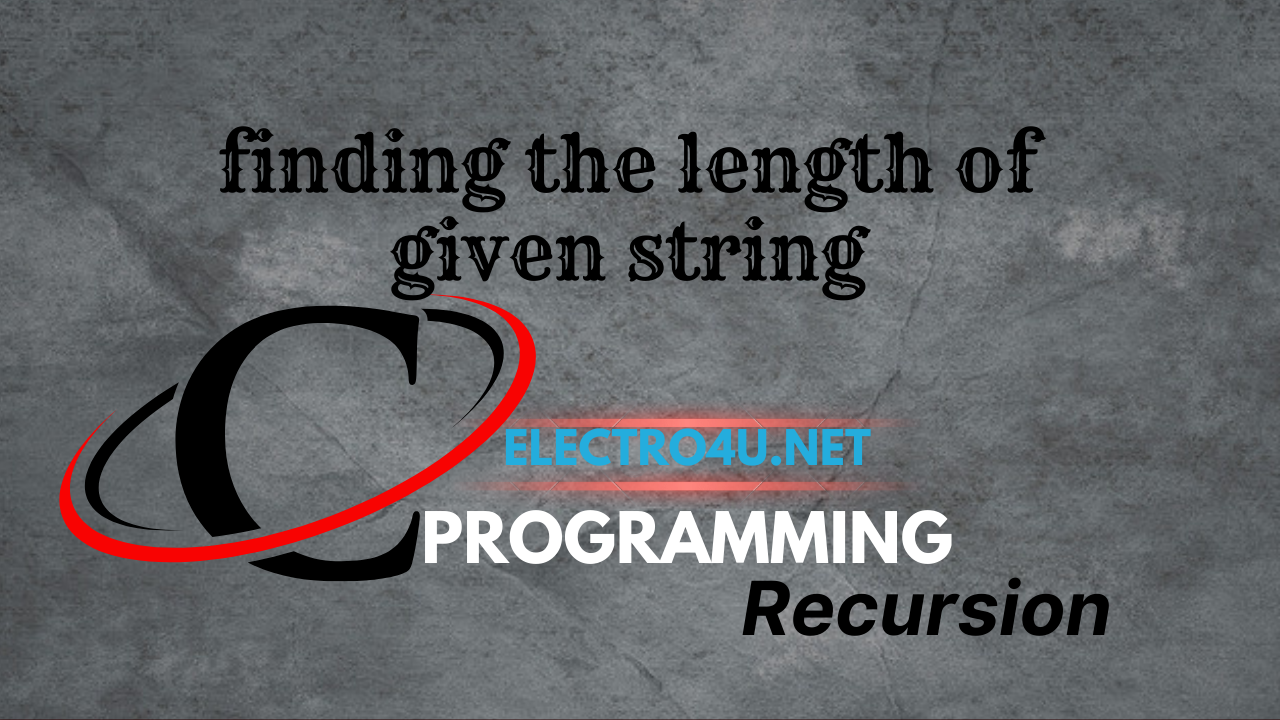
design a recursive function for finding the length of given string
Design a recursive function for finding the length of a given string" means to create a function that takes a string as input and recursively calculates the number of characters in the string in the C programming language.
The function should take a string as an argument, and recursively count each character of the string until a base case is reached. The base case is typically when the function reaches the end of the string (i.e. the null terminator character '\0'), at which point the recursion should stop.
The recursive function should add 1 to the count of characters for each recursive call until the base case is reached. The design of the function should ensure that it works for any string of characters that is within the range of representable strings in C.
Once the function has calculated the length of the string using recursion, it should return the length to the calling function or print it to the console as required.
#include <stdio.h>
int stringLength(char str[]) {
if (*str == '\0') { // base case: end of string reached
return 0;
}
return 1 + stringLength(str + 1); // recursive call with the next character
}
int main() {
char str[] = "Hello, World!";
int length = stringLength(str);
printf("Length of the string is %d\n", length);
return 0;
}
In this example, the stringLength function takes a character array str as an argument and returns the length of the string using recursion.
The base case is when the current character is the null terminator character '\0', which indicates the end of the string. In this case, the function returns 0, indicating that the length of the string is 0.
The recursive case is when the current character is not the null terminator character. The function makes a recursive call to stringLength with the next character of the string (str + 1) as the argument, and adds 1 to the result. This recursively counts the number of characters in the string until the end of the string is reached.
Finally, in the main function, a test string is declared and passed to the stringLength function. This causes the length of the string to be calculated and printed to the console.
Second method
#include<stdio.h>
int my_strlen(const char*);
void main()
{
char s[]="abcd";
int r;
r=my_strlen(s);
printf("\n r=%d\n",r);
}
int my_strlen(const char *p)
{
if(*p)
{
return 1+my_strlen(p+1);
}
else
return 0;
}