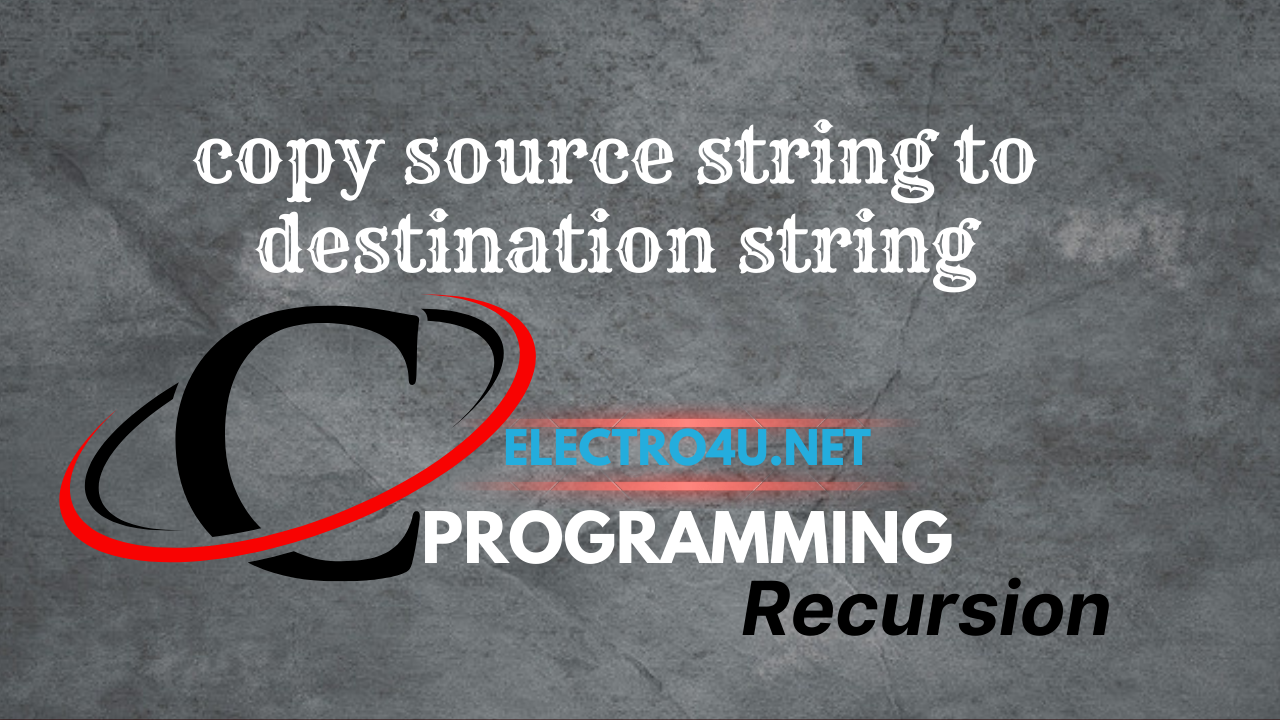
Design a recursive function to copy the data source string to destination string
what does mean Design a recursive function to copy the data source string to the destination string
"Design a recursive function to copy the data source string to destination string" means creating a function that takes two character arrays (strings) as input and recursively copies the contents of the first array (the source string) to the second array (the destination string) in the C programming language.
The function should take two character arrays as arguments: the source string and the destination string. The function should recursively copy each character of the source string to the corresponding position in the destination string until a base case is reached.
The base case is typically when the function reaches the end of the source string (i.e. the null terminator character '\0'), at which point the recursion should stop.
The recursive function should copy each character from the source string to the corresponding position in the destination string using assignment (=) or a string function such as strcpy(). The design of the function should ensure that it works for any string of characters that is within the range of representable strings in C.
Once the function has copied the contents of the source string to the destination string using recursion, it should return the destination string or print it to the console as required.
Example 01 of a recursive function in C that copies the contents of the source string to the destination string using recursion:
#include
void my_strcpy(char *p,char *q);
int main()
{
char s1[10],s2[10];
printf("Enter the 1st string:");
scanf("%[^\n]",&s1);
my_strcpy(s1,s2);
printf("string s1=%s and string s2=%s\n",s1,s2);
printf("print sucessfully.....\n");
}
void my_strcpy(char *p,char *q)
{
if(*p)
{
*q=*p;
my_strcpy(p+1,q+1);
}
else
*q=*p;
}
Example 02 of a recursive function in C that copies the contents of the source string to the destination string using recursion
#include <stdio.h>
void stringCopy(char dest[], char src[], int i) {
if (src[i] == '\0') { // base case: end of source string reached
dest[i] = '\0'; // terminate the destination string
return;
}
dest[i] = src[i]; // copy current character to destination
stringCopy(dest, src, i + 1); // recursive call with the next character
}
int main() {
char source[] = "Hello, World!";
char destination[50];
stringCopy(destination, source, 0);
printf("Source string: %s\n", source);
printf("Destination string: %s\n", destination);
return 0;
}
In this example, the stringCopy function takes two character arrays dest and src as arguments, as well as an integer i to keep track of the current index in the strings.
The base case is when the current character in the source string is the null terminator character '\0', which indicates the end of the string. In this case, the function sets the corresponding position in the destination string to the null terminator character as well, effectively terminating the destination string. Then it returns to the previous recursive call.
The recursive case is when the current character in the source string is not the null terminator character. The function copies the current character from the source string to the corresponding position in the destination string using assignment (=). Then it makes a recursive call to stringCopy with the next index in the strings (i + 1) as the argument.
Finally, in the main function, a test source string is declared and a destination string of sufficient length is created. The stringCopy function is called with these arguments, causing the contents of the source string to be copied to the destination string using recursion. The source and destination strings are then printed to the console to verify the result.