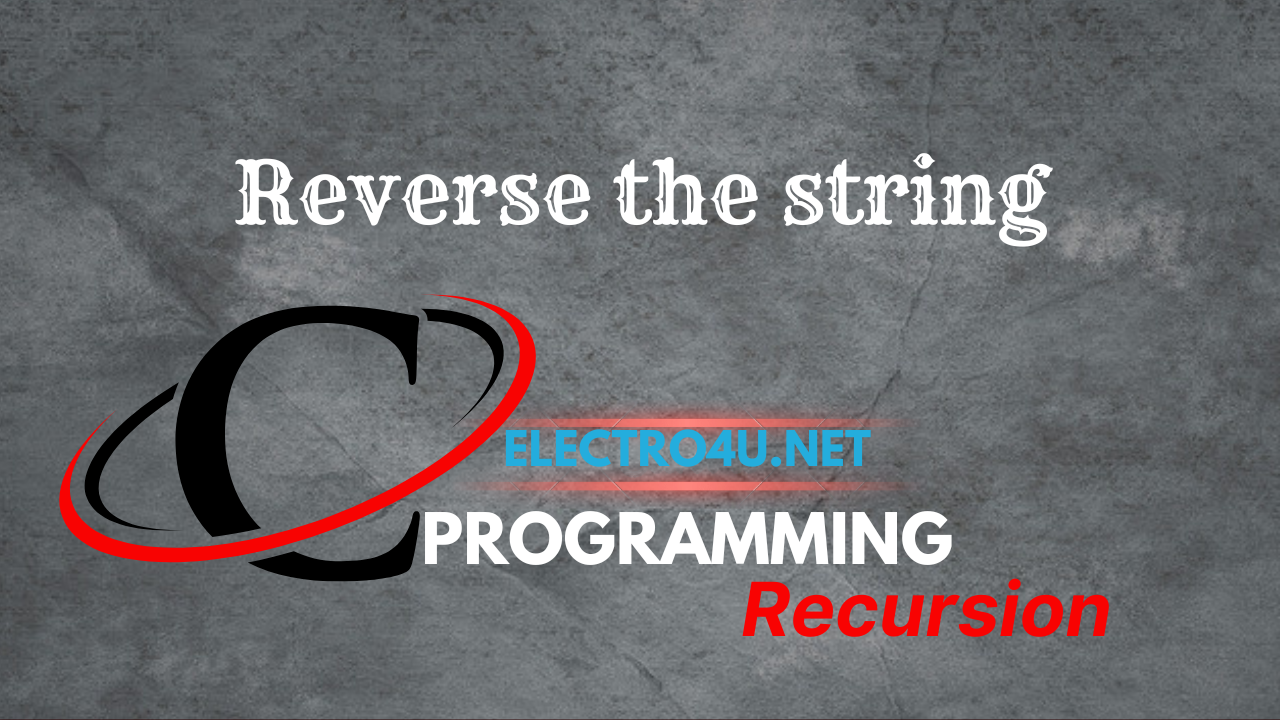
Design a recursive function reverse the string in c
what does mean to Design a recursive function to reverse the string in c
Designing a recursive function to reverse a string in C means creating a function that takes a string as input and returns the string in reverse order using recursion in the C programming language.
Example 01 implementation in C
#include<stdio.h>
void string_rev(char *,int);
int my_strlen(const char *);
int main()
{
char s[10];
int i;
printf("Enter the string:");
scanf("%[^\n]",s);
i=my_strlen(s);
printf("String lenth is:%d\n",i);
printf("Entered string is:%s\n",s);
string_rev(s,i-1);
printf("After:%s\n",s);
}
void string_rev(char *s,int i)
{
static char t,j=0;
if(j<i)
{
t=s[i];
s[i]=s[j];
s[j]=t;
j++;
i--;
string_rev(s,i);
}
}
int my_strlen(const char *p)
{
if(*p)
{
return 1+my_strlen(p+1);
}
else
return 0;
}
Output:
Enter the string: Balmiki
String length is:7
Entered string is: Balmiki
After: ikimlaB
Example 02 implementation in C
#include <stdio.h>
#include <string.h>
void reverse_string(char *str);
int main() {
char str[100];
printf("Enter a string: ");
fgets(str, 100, stdin);
reverse_string(str);
printf("Reversed string is: %s", str);
return 0;
}
void reverse_string(char *str) {
if (*str == '\0') {
return;
} else {
reverse_string(str + 1);
printf("%c", *str);
}
}
Attention: In this implementation, we define a function reverse_string that takes a character pointer str as input. If the first character of str is the null character ('\0'), which signifies the end of the string, we simply return. Otherwise, we recursively call the reverse_string function on the rest of the string (str + 1) and print the first character (*str) after the recursive call is complete. This effectively reverses the string recursively.
Note that this implementation prints the reversed string character by character as it is being reversed. To store the reversed string in a separate variable, you can modify the implementation to use a helper function that takes an additional argument to keep track of the reversed string.