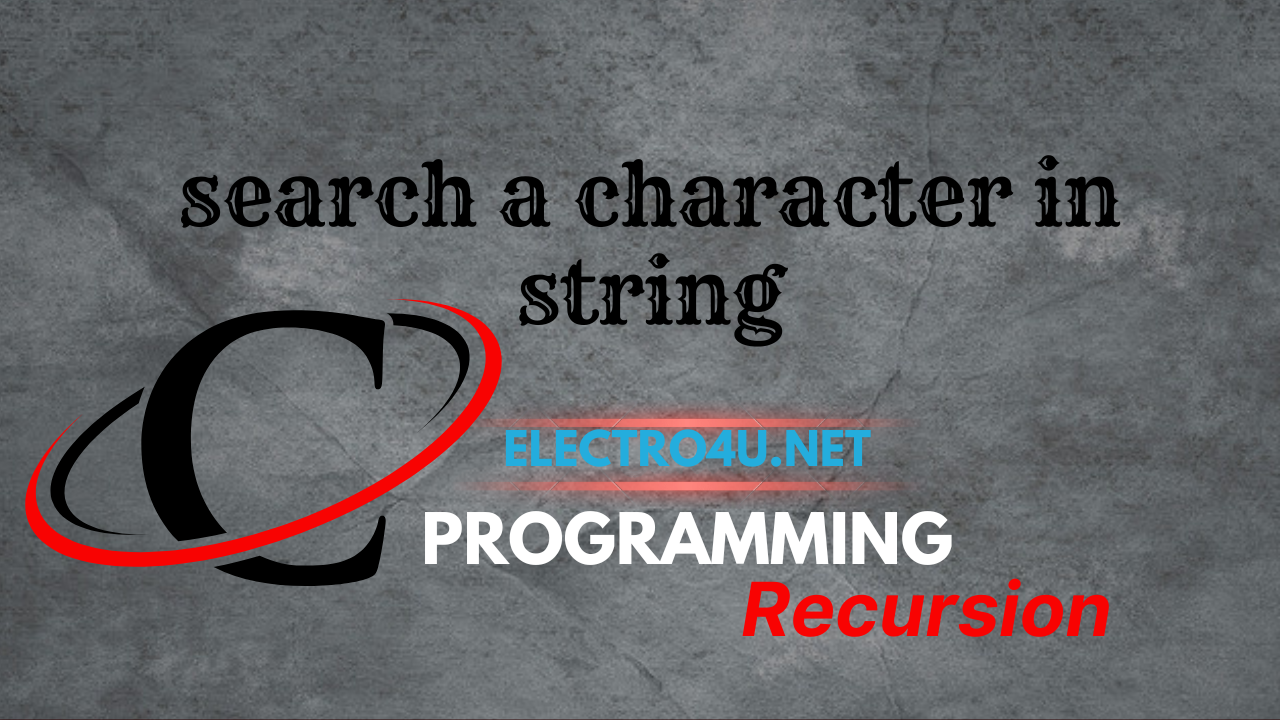
Design a recursive function to search a character in given string in c
what does mean to Design a recursive function to search a character in given string in c
Designing a recursive function to search for a character in a given string in C means creating a function that takes a character and a string as input and returns true if the character is present in the string and false otherwise, using recursion in the C programming language.
Here's an example 01 implementation in C:
#include<stdio.h>
char *strchr_recfun(const char *,char );
int main()
{
char s[10],ch;
printf("Enter the string:");
scanf("%[^\n]",s);
printf("Enter the character for searching:");
scanf(" %c",&ch);
if(strchr_recfun(s,ch))
printf("Character is there in this string:\n");
else
printf("Charcater is not There in this string:\n");
}
char *strchr_recfun(const char *s,char ch)
{
if(*s)
{
if(*s==ch)
return s;
else
return strchr_recfun(s+1,ch);
}
else
return 0;
}
Output:
Enter the string: Balmiki
Enter the character for searching: B
Character is there in this string
Enter the string: Balmiki
Enter the character for searching:b
Character is not There in this string:
Here's an example 02 implementation in C:
#include <stdio.h>
#include <string.h>
int search_character(char c, char *str);
int main() {
char str[100], c;
printf("Enter a string: ");
fgets(str, 100, stdin);
printf("Enter a character to search: ");
scanf("%c", &c);
if (search_character(c, str)) {
printf("Character found\n");
} else {
printf("Character not found\n");
}
return 0;
}
int search_character(char c, char *str) {
if (*str == '\0') {
return 0;
} else if (*str == c) {
return 1;
} else {
return search_character(c, str + 1);
}
}
In this implementation, we define a function search_character that takes a character c and a character pointer str as input. If the first character of str is the null character ('\0'), which signifies the end of the string, we return 0 to indicate that the character is not found. Otherwise, if the first character of str is the character we are searching for (c), we return 1 to indicate that the character is found. Otherwise, we recursively call the search_character function on the rest of the string (str + 1). This checks the rest of the string for the character recursively.
Note that in this implementation, we return an integer instead of a boolean value. 0 is used to represent false and 1 is used to represent true. This is because C does not have a boolean type. If you are using a C++ compiler, you can use the bool type and return true or false instead of 1 or 0.