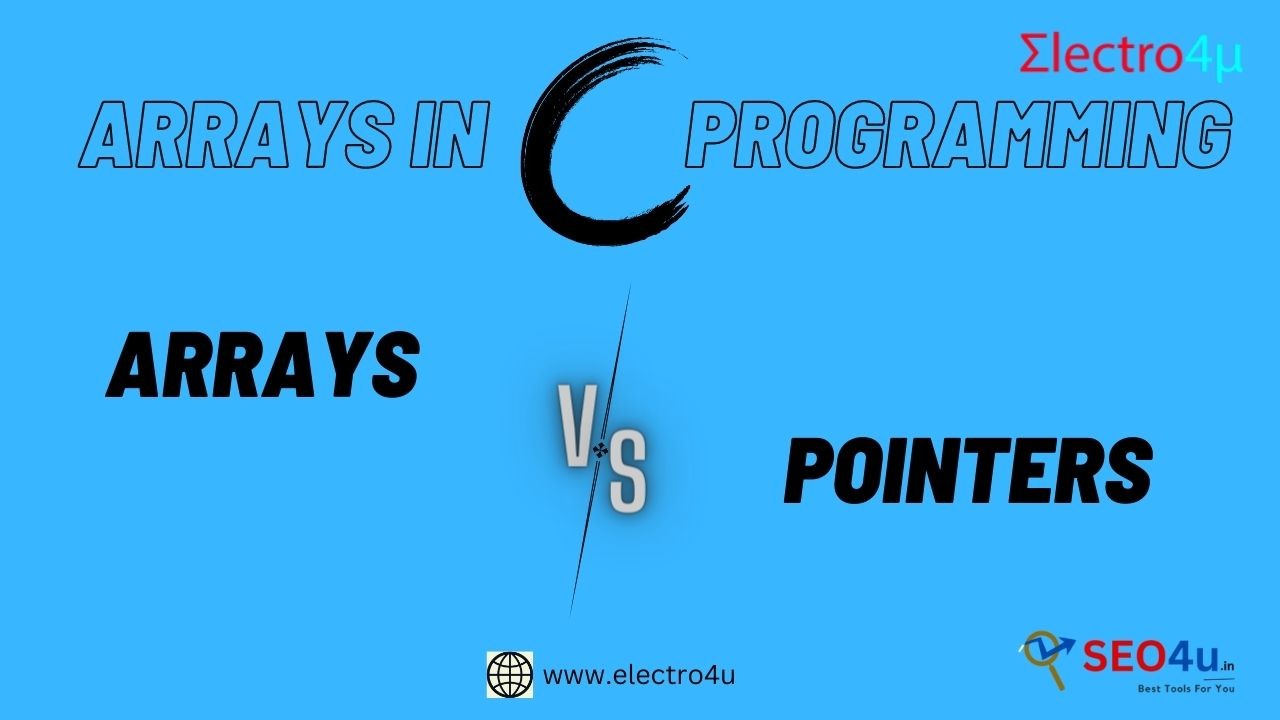
What are the differences between Arrays & Pointers?
Understanding the Differences Between Arrays and Pointers in Programming
Arrays and pointers are two closely related concepts in programming. However, there are some key differences between the two.
Array
- An array is a collection of elements of the same data type.
- Arrays are static in nature, meaning that their size cannot be changed once they are declared.
- Arrays are allocated at compile time.
- Arrays can be initialized at the time of declaration.
- Arrays can be accessed using their indices.
Pointer
- A pointer is a variable that stores the address of another variable.
- Pointers are dynamic in nature, meaning that their value can be changed at runtime.
- Pointers are allocated at runtime.
- Pointers cannot be initialized at the time of declaration.
- Pointers are accessed using the dereference operator (*).
Table summarizes the key differences between arrays and pointers:
Feature | Array | Pointer |
---|---|---|
Data type | Collection of elements of the same data type | Variable that stores the address of another variable |
Size | Static | Dynamic |
Allocation | Compile time | Runtime |
Initialization | Can be initialized at the time of declaration | Cannot be initialized at the time of declaration |
Access | Using indices | Using the dereference operator (*) |
Example an array declaration and initialization:
int arr[5] = {1, 2, 3, 4, 5};
This code declares an array of 5 integers and initializes it with the values 1, 2, 3, 4, and 5.
Example of pointer declaration and initialization:
int *ptr;
This code declares a pointer to an integer. The pointer is not initialized, so it does not point to any variable yet.
To use the pointer, we can assign it the address of a variable:
ptr = &arr[0];
This code assigns the address of the first element of the arr array to the ptr pointer.
We can now access the element of the arr array pointed to by ptr using the dereference operator:
int value = *ptr;
This code will store the value of the first element of the arr array in the variable value.
Conclusion
Arrays and pointers are two powerful tools in programming. However, it is important to understand the differences between the two in order to use them effectively.