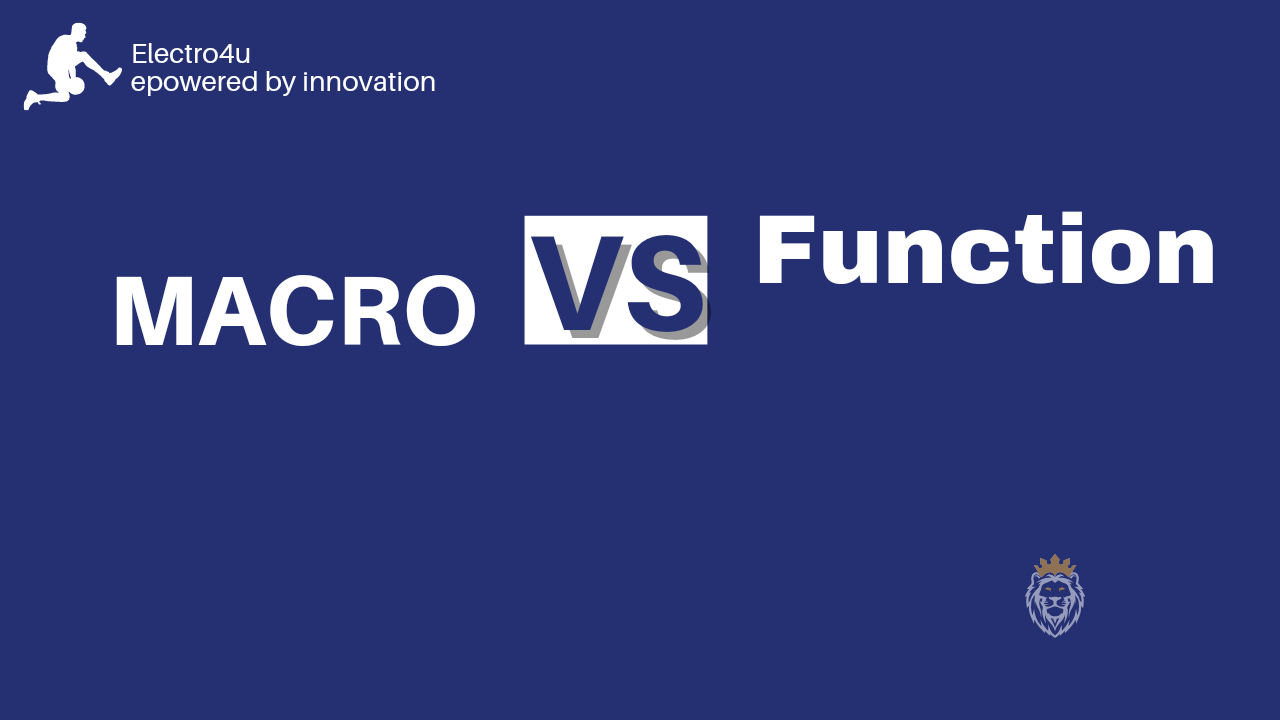
What are the differences between macros and functions in c?
Differences Between Macros and Functions
Introduction: When it comes to programming, understanding the differences between macros and functions is essential. Both macros and functions serve as powerful tools for code reuse and abstraction, but they have distinct characteristics and use cases. In this article, we'll explore the key differences between macros and functions to help you make informed decisions in your programming endeavors.
1. Definition:
- Macros: Macros are preprocessor directives that perform text replacement before the code is compiled. They are typically defined using #define and operate on the source code directly.
- Functions: Functions are blocks of code that encapsulate a set of instructions and can be invoked by calling their name. They are defined using a formal declaration and can accept parameters and return values.
2. Evaluation Time:
- Macros: Macros are evaluated at compile-time. The preprocessor replaces macro calls with their corresponding code before the compiler processes the code.
- Functions: Functions are evaluated at runtime. They execute when called during the program's execution.
3. Type Safety:
- Macros: Macros lack type safety since they work with raw text substitution. This can lead to unexpected behavior if not used carefully.
- Functions: Functions provide strong type safety, as they are explicitly defined with data types for parameters and return values, helping to catch type-related errors during compilation.
4. Debugging:
- Macros: Debugging macros can be challenging because the code generated by macros is not directly visible in the source code.
- Functions: Debugging functions is more straightforward since you can set breakpoints and inspect the code during runtime.
5. Code Readability:
- Macros: Macros can make the code harder to read and understand due to the text replacement nature, which may obscure the original intent.
- Functions: Functions promote code readability by encapsulating logic into named blocks, making the code more self-explanatory.
6. Functionality:
- Macros: Macros can perform complex code manipulations and generate repetitive code patterns, but they lack the ability to return values or execute conditional logic.
- Functions: Functions can perform complex operations, return values, and incorporate conditional statements, offering more flexibility and control.
7. Usage Scenarios:
- Macros: Macros are often used for simple code generation tasks, defining constants, and creating inline short functions, such as max and min.
- Functions: Functions are suitable for organizing code, promoting reusability, and encapsulating complex algorithms.
8. Scope:
- Macros: Macros have a global scope and can be used throughout the entire program.
- Functions: Functions have a limited scope defined by where they are declared, making them more modular and maintainable.
Key differences between macros and functions:
Characteristic | Macro | Function |
---|---|---|
Expansion | Preprocessed before compilation | Compiled as part of the program |
Execution | At compile time | At runtime |
Arguments | Can take arguments, but are not required | Can take arguments, and can return values |
Side effects | Can have side effects, such as changing global variables | Should not have side effects |
Type checking | No type checking | Type checking is performed |
Debugging | Can be difficult to debug | Easier to debug |
Here are some examples of how to use macros and functions:
Macro example:
#define MAX_SIZE 100
int main() {
int array[MAX_SIZE];
// ...
}
In this example, the macro MAX_SIZE is used to define the maximum size of the array. This makes the code more readable and maintainable, because the maximum size of the array is only defined in one place.
Function example:
int add_two_numbers(int a, int b) {
return a + b;
}
int main() {
int sum = add_two_numbers(1, 2);
// ...
}
In this example, the function add_two_numbers() is used to add two numbers together. This function can be reused throughout the program, without having to write the same code multiple times.
Which one to use?
In general, it is best to use functions instead of macros. Functions are more powerful and flexible than macros, and they are easier to debug. However, there are some cases where macros can be useful, such as when you need to define a constant or abbreviation.
Here are some tips for choosing between macros and functions:
- Use a function if you need to reuse a block of code multiple times.
- Use a function if you need to pass arguments to the code or return a value.
- Use a function if you need to avoid side effects.
- Use a macro if you need to define a constant or abbreviation.
If you are not sure whether to use a macro or a function, it is always best to err on the side of caution and use a function.
Conclusion:
In summary, macros and functions are distinct programming constructs, each with its own set of advantages and limitations. Choosing between macros and functions depends on the specific requirements of your project and the trade-offs between code readability, maintainability, and performance. Understanding when and how to use macros and functions effectively is a valuable skill for any programmer.
For further information and examples, Please visit C-Programming From Scratch to Advanced 2023-2024