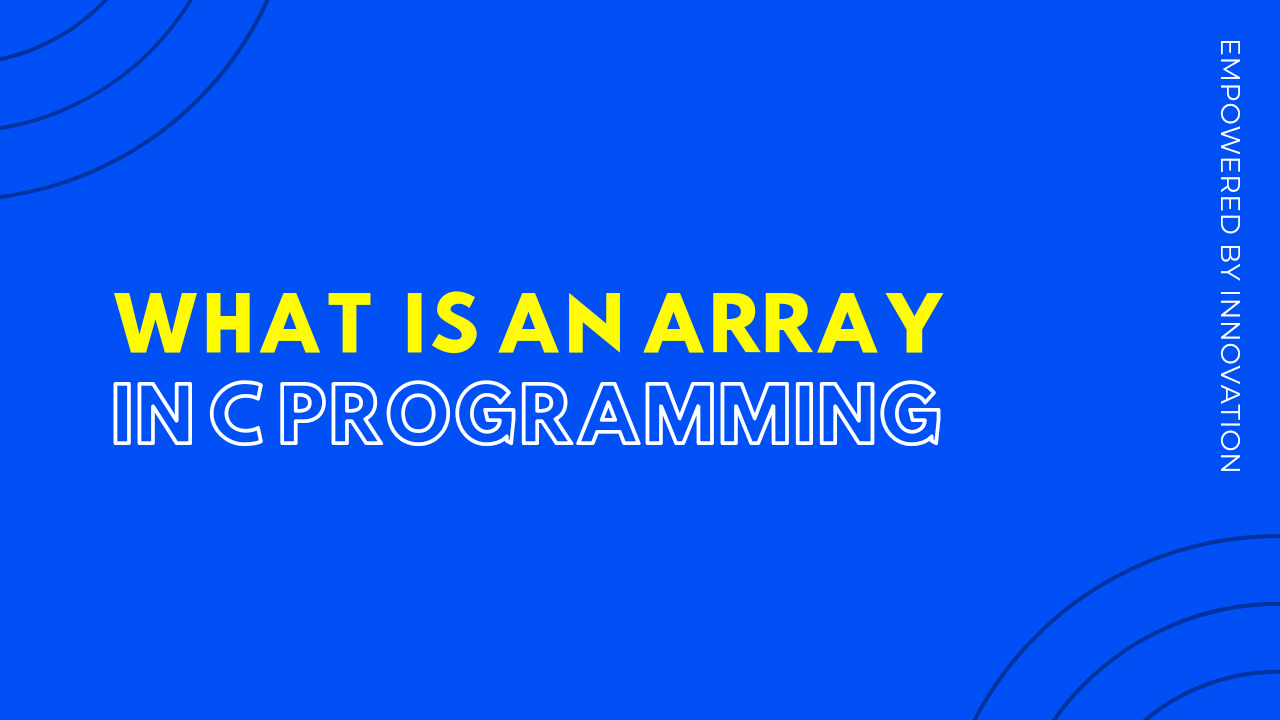
What is Array in c
What is an Array in C? A Comprehensive Guide
In C programming language, an array is a collection of elements of the same data type that are stored in contiguous memory locations. The elements in an array can be accessed using an index or a subscript, which starts from zero. Arrays in C are useful for storing and manipulating large sets of data efficiently.
In C, arrays can be declared statically or dynamically. Static arrays have a fixed size that is specified at compile time, while dynamic arrays can be resized during runtime using memory allocation functions such as malloc() and calloc().
syntax: Data_type variable name[ele];
Here's an example of a static array declaration in C:
int myArray[5]; // Declares an integer array with five elements
And here's an example of a dynamic array declaration in C:
int *myArray; // Declares a pointer to an integer array
myArray = (int *)malloc(5 * sizeof(int)); // Allocates memory for an integer array with five elements
In both cases, the array can be accessed using the index notation, like this:
myArray[0] = 10; // Assigns 10 to the first element of the array
int x = myArray[2]; // Retrieves the value of the third element of the array
Further Reading:
write a c program to reverse the elements of a given array.
write a c program to delete an element at desired from an array
write a c program to insert an element at a desired position in an array
write a c program that deletes the duplicate elements of an array.
write a c program to find the duplicate elements of a given array and find the count of duplicate elements.
write a c program to print non-repeated numbers of a given array.
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!