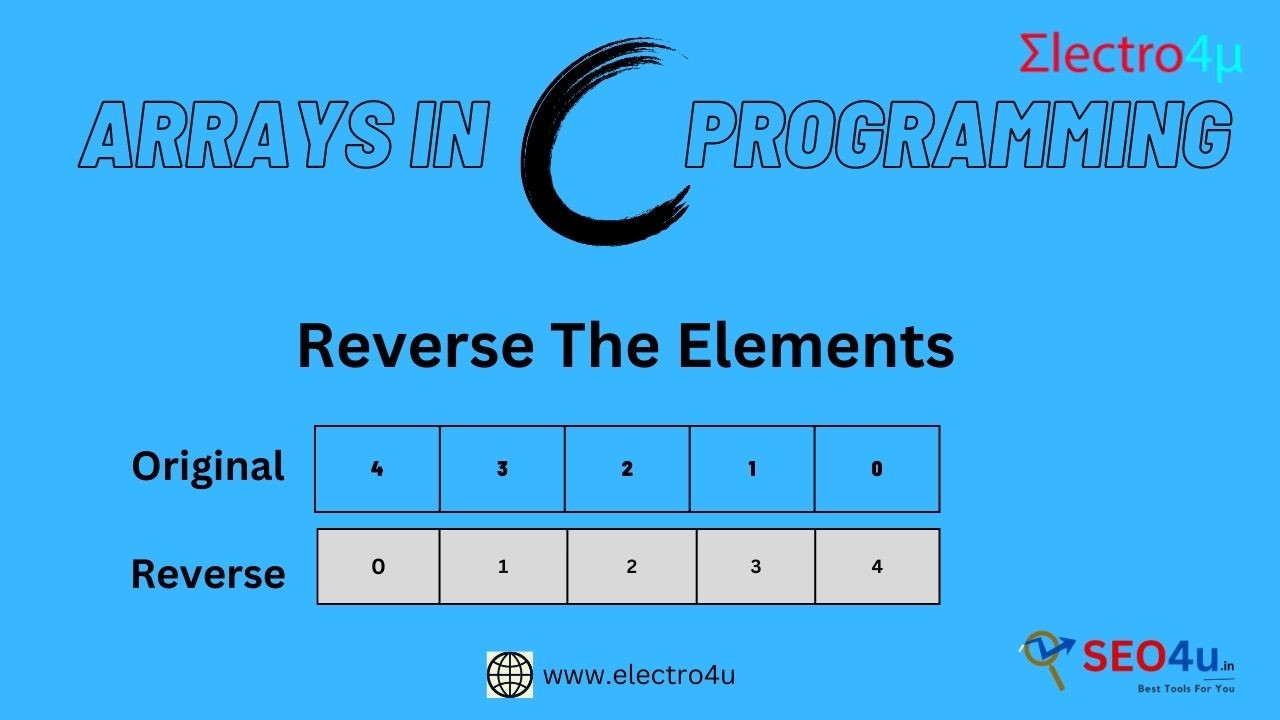
Write a c program to reverse the elements of a given array
Array Reversal in C: Step-by-Step Guide
To reverse the elements of a given array in C, we can use the following steps:
- Declare an integer variable i to iterate over the array.
- Initialize i to 0.
- While i is less than the length of the array:
- Swap the elements at indices i and length - i - 1.
- Increment i by 1.
- Return the array.
The following code shows how to implement these steps in C:
C programming
void reverseArray(int arr[], int length) {
int i;
for (i = 0; i < length / 2; i++) {
int temp = arr[i];
arr[i] = arr[length - i - 1];
arr[length - i - 1] = temp;
}
}
int main() {
int arr[] = {1, 2, 3, 4, 5};
int length = sizeof(arr) / sizeof(arr[0]);
reverseArray(arr, length);
for (int i = 0; i < length; i++) {
printf("%d ", arr[i]);
}
printf("\n");
return 0;
}
Output:
5 4 3 2 1
Top Resources
Copy in a reverse manner in c
Write a program to reverse printing not reversing of 10 elements of array in c
Write a program to reverse the bits of a given number using for loop
Design a function to reverse the given string
Design a function to reverse the word in given string
write a program to copy one array element to another array element in reverse manner.
write a program to reverse adjacent elements of an array.
write a program to reverse half of the elements of an array.
Further Reading:
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!