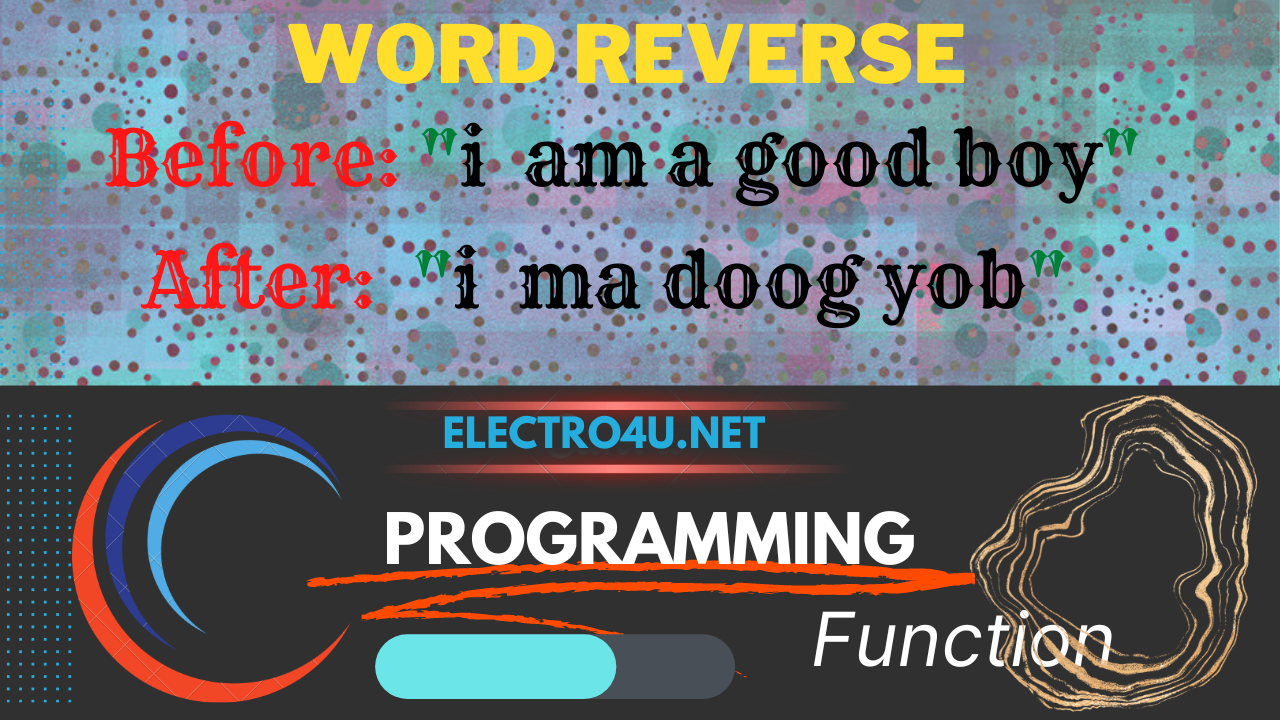
Design a function to reverse the word in given string in c
Reversing Words in a String using a C Function
"Design a function to reverse the word in given string"
means to create a function that takes a string as input, and then reverses the order of the words in the string. For example, if the input string is "hello world", the output should be "world hello".
To reverse the order of the words in a string, you can split the string into individual words (using a space character as the delimiter), reverse the order of the resulting array of words, and then join the words back into a single string (again using space as the delimiter).
It's important to note that "reverse the word" usually means to reverse the characters within each word, not to reverse the order of the characters within the word. So, in the example "hello world",
the words "hello"
and "world"
would be reversed to "olleh"
and "dlrow"
, respectively, but the order of the letters within each word would remain unchanged.
To design a function to reverse the word in a given string in C, we can use the following steps:
- Declare a function called reverse_word(), which takes a string as input and returns a reversed string as output.
- Inside the function, create two pointers, start and end. Initialize both pointers to the beginning of the string.
- Iterate over the string, swapping the characters at start and end until start is greater than or equal to end.
- Return the reversed string.
C code example of the reverse_word() function:
#include <stdio.h>
char *reverse_word(char *str) {
char *start = str;
char *end = str + strlen(str) - 1;
while (start < end) {
char temp = *start;
*start = *end;
*end = temp;
start++;
end--;
}
return str;
}
int main() {
char str[] = "Hello, World!";
char *reversed_word = reverse_word(str);
printf("The reversed word is: %s\n", reversed_word);
return 0;
}
Output:
The reversed word is: !dlroW ,olleH
Further Reading:
Concatenating Two Strings in C: A Step-by-Step Guide
Design a function to count given character in given string
Design a function to reverse the given string
Design a function to search given character in given string in c
Design a function to reverse the word in given string
Design a function replace the words in reverse order in a given string line
design a function to reverse data in between two location
Design a function to find string length in c
Design a Function to print the string character by character
Design a function to print the elements of a given integers array.
Design a function for bubble sort in c
Design a function to swapping of two number
Design a function to check the given number is Armstrong or not if Armstrong return 1 else 0
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [[email protected]].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!