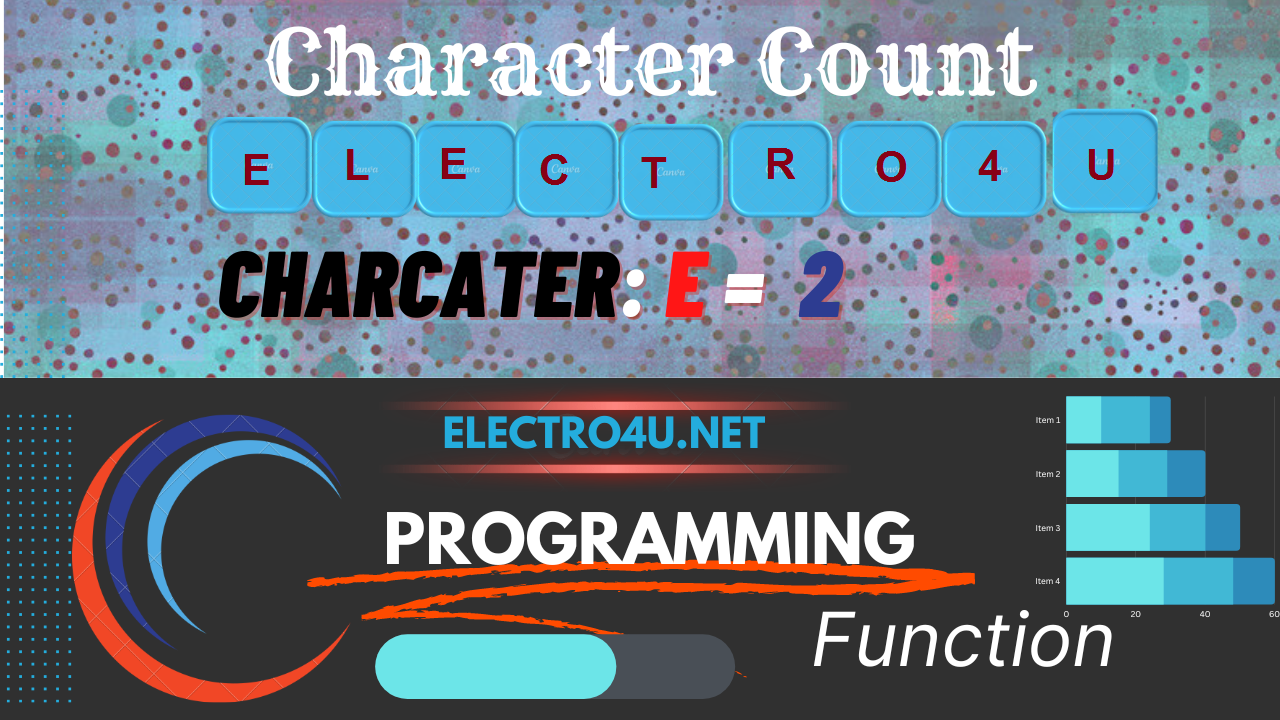
Design a function to count given character in given string
Count Occurrences of a Character in a String using a C Function
To design a function to count a given character in a given string in C, we can follow these steps:
Declare a function prototype with the following parameters:
- string: The pointer to the string to be searched.
- character: The character to be searched for.
- count: The pointer to the variable where the count of the occurrences of the character in the string will be stored.
Implement the function body as follows:
C Programming
int count_character(char *string, char character, int *count) {
// Initialize the count variable.
*count = 0;
// Iterate over the string.
while (*string != '\0') {
// If the current character matches the given character, increment the count.
if (*string == character) {
(*count)++;
}
// Move to the next character in the string.
string++;
}
// Return the count.
return *count;
}
To use the function,
we can pass the pointer to the string and the character to be searched for as arguments to the function. The function will return the count of the occurrences of the character in the string.
How to use the count_character() function:
C Programming
#include <stdio.h>
int count_character(char *string, char character, int *count);
int main() {
// Declare a string.
char string[] = "Hello, world!";
// Declare a character to be searched for.
char character = 'l';
// Declare a variable to store the count of the occurrences of the character in the string.
int count;
// Call the count_character() function.
count = count_character(string, character, &count);
// Print the count.
printf("The number of occurrences of the character '%c' in the string '%s' is %d.\n", character, string, count);
return 0;
}
Output:
The number of occurrences of the character 'l' in the string 'Hello, world!' is 3.
Further Reading:
Concatenating Two Strings in C: A Step-by-Step Guide
Design a function to count given character in given string
Design a function to reverse the given string
Design a function to search given character in given string in c
Design a function to reverse the word in given string
Design a function replace the words in reverse order in a given string line
design a function to reverse data in between two location
Design a function to find string length in c
Design a Function to print the string character by character
Design a function to print the elements of a given integers array.
Design a function for bubble sort in c
Design a function to swapping of two number
Design a function to check the given number is Armstrong or not if Armstrong return 1 else 0
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!