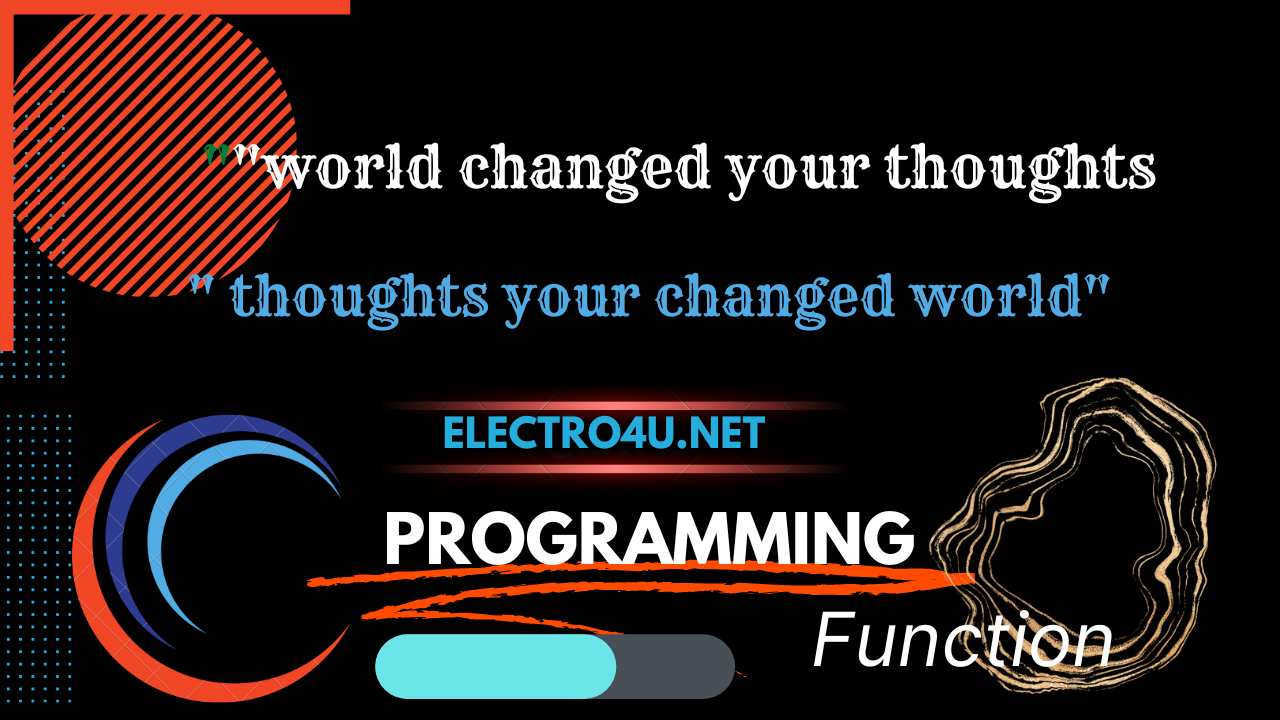
Design a function replace the words in reverse order in a given string line in c
What does mean Replace the words in reverse order in a given string line
"Replace the words in reverse order in a given string line"
means to take a given string of text and reverse the order of the words within the string.For example, if the original string is "The quick brown fox",
the function should replace it with "fox brown quick The"
by reversing the order of the words.
To do this,
we need to identify the individual words in the string and reverse their order. One approach is to reverse the entire string first, then iterate through the reversed string and reverse each individual word back to its original order.
Note that "word"
here refers to any sequence of characters separated by whitespaces, such as a space, tab, or newline. Punctuation marks and other special characters should be treated as part of the word they are attached to.
Reverse Word Replacement Function in a String
To design a function in C to replace the words in reverse order in a given string line, we can follow these steps:
- Create a function called
replace_words_in_reverse_order()
that takes a string as input and returns a string as output. - Split the input string into an array of words using the
strtok()
function. - Reverse the order of the words in the array.
- Join the reversed words in the array into a string using the
strcat()
function. - Return the reversed string.
C code implementation of the replace_words_in_reverse_order() function:
#include <stdio.h>
#include <string.h>
char *replace_words_in_reverse_order(char *line) {
// Split the input string into an array of words using the strtok() function.
char *words[100];
int i = 0;
char *word = strtok(line, " ");
while (word != NULL) {
words[i++] = word;
word = strtok(NULL, " ");
}
// Reverse the order of the words in the array.
for (int j = 0; j < i / 2; j++) {
char *temp = words[j];
words[j] = words[i - j - 1];
words[i - j - 1] = temp;
}
// Join the reversed words in the array into a string using the strcat() function.
char reversed_line[100] = "";
for (int j = 0; j < i; j++) {
strcat(reversed_line, words[j]);
if (j < i - 1) {
strcat(reversed_line, " ");
}
}
// Return the reversed string.
return reversed_line;
}
int main() {
char line[100] = "This is a test string.";
char *reversed_line = replace_words_in_reverse_order(line);
printf("The reversed line is: %s\n", reversed_line);
return 0;
}
Output:
The reversed line is: string. test a is This
Further Reading:
Concatenating Two Strings in C: A Step-by-Step Guide
Design a function to count given character in given string
Design a function to reverse the given string
Design a function to search given character in given string in c
Design a function to reverse the word in given string
Design a function replace the words in reverse order in a given string line
design a function to reverse data in between two location
Design a function to find string length in c
Design a Function to print the string character by character
Design a function to print the elements of a given integers array.
Design a function for bubble sort in c
Design a function to swapping of two number
Design a function to check the given number is Armstrong or not if Armstrong return 1 else 0
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!