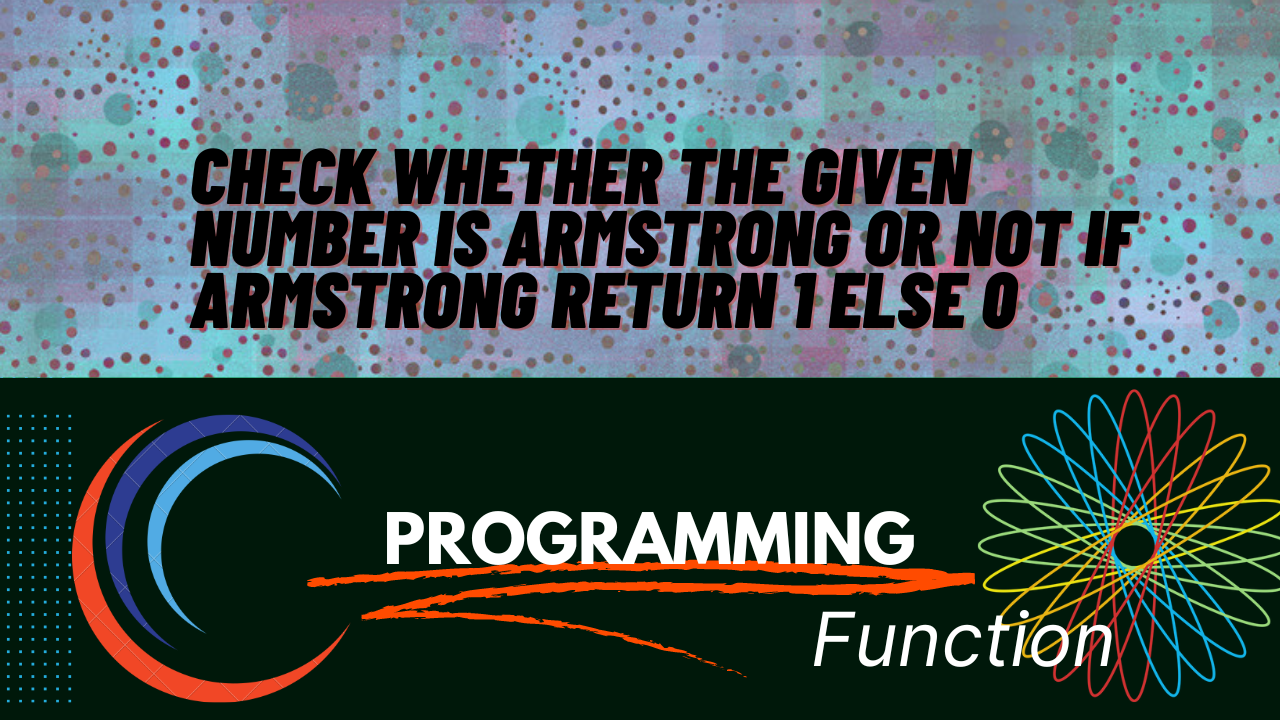
Design a function to check the given number is Armstrong or not if Armstrong return 1 else 0
Design a C Function to Check if a Number is Armstrong or Not
"Design a function to check the given number is Armstrong or not if Armstrong return 1 else 0"
means that you are asked to write a function that takes a number as an argument and checks whether it is an Armstrong number or not. If the number is an Armstrong number, the function should return 1
(which indicates true). Otherwise, the function should return 0
(which indicates false).
An Armstrong number is a number where the sum of each digit raised to the power of the number of digits in the number is equal to the original number. For example, 153
is an Armstrong number because 1^3 + 5^3 + 3^3 = 153.
So, the function should check whether the input number meets this condition and return 1
if it is an Armstrong number or 0
if it is not.
To design a function to check if a given number is Armstrong in C, we can use the following steps:
Declare the function prototype. This tells the compiler the name of the function, its return type, and the type of its arguments.
int isArmstrong(int n);
Implement the function body. This is where the code to check if the number is Armstrong goes.
int isArmstrong(int n) {
// Calculate the number of digits in the number.
int digits = 0;
while (n > 0) {
digits++;
n /= 10;
}
// Calculate the sum of the cubes of the digits.
int sum = 0;
for (int i = 0; i < digits; i++) {
int digit = n % 10;
sum += digit * digit * digit;
n /= 10;
}
// Return 1 if the sum is equal to the number, else return 0.
return sum == n;
}
Use the function in your main program.
int main() {
int n;
printf("Enter a number: ");
scanf("%d", &n);
if (isArmstrong(n)) {
printf("%d is an Armstrong number.\n", n);
} else {
printf("%d is not an Armstrong number.\n", n);
}
return 0;
}
Enter a number: 153
153 is an Armstrong number.
Further Reading:
Concatenating Two Strings in C: A Step-by-Step Guide
Design a function to count given character in given string
Design a function to reverse the given string
Design a function to search given character in given string in c
Design a function to reverse the word in given string
Design a function replace the words in reverse order in a given string line
design a function to reverse data in between two location
Design a function to find string length in c
Design a Function to print the string character by character
Design a function to print the elements of a given integers array.
Design a function for bubble sort in c
Design a function to swapping of two number
Design a function to check the given number is Armstrong or not if Armstrong return 1 else 0
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!