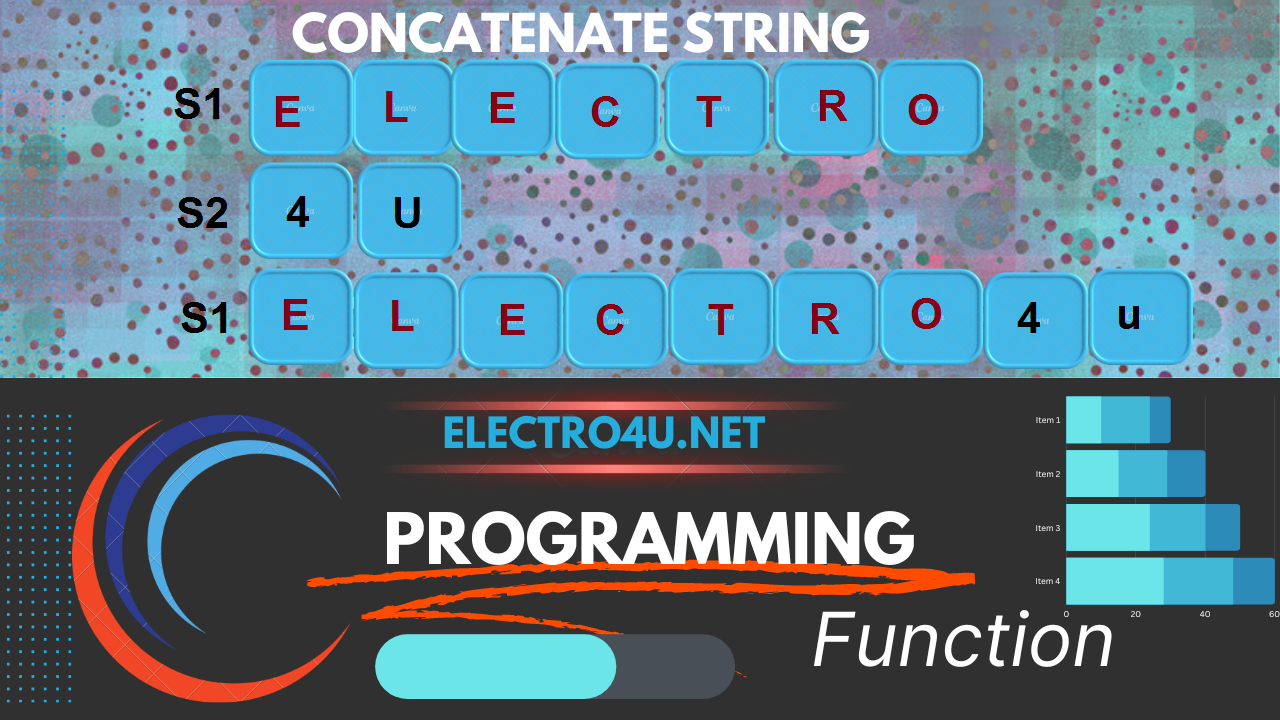
Design a function Concatenate two string in c
Concatenating Two Strings in C: A Step-by-Step Guide
To design a function to concatenate two strings in C, we can use the following steps:
- Declare the function prototype with two string parameters and a string return value.
- Within the function body, use the
strlen()
function to get the length of the first string. - Allocate a new string of sufficient size to hold the concatenated string.
- Copy the first string to the new string.
- Append the second string to the new string.
- Return the new string.
C function to concatenate two strings:
C Prpogramming
#include <string.h>
char* Concatenate(char* str1, char* str2) {
// Get the length of the first string.
size_t len1 = strlen(str1);
// Allocate a new string of sufficient size to hold the concatenated string.
char* str = malloc(len1 + strlen(str2) + 1);
if (str == NULL) {
return NULL;
}
// Copy the first string to the new string.
strcpy(str, str1);
// Append the second string to the new string.
strcat(str, str2);
// Return the new string.
return str;
}
This function can be used to concatenate two strings as follows:
C Programming
char* str1 = "Hello";
char* str2 = "World";
// Concatenate the two strings.
char* str = Concatenate(str1, str2);
// Print the concatenated string.
printf("%s\n", str);
Output:
HelloWorld
Further Reading:
Concatenating Two Strings in C: A Step-by-Step Guide
Design a function to count given character in given string
Design a function to reverse the given string
Design a function to search given character in given string in c
Design a function to reverse the word in given string
Design a function replace the words in reverse order in a given string line
design a function to reverse data in between two location
Design a function to find string length in c
Design a Function to print the string character by character
Design a function to print the elements of a given integers array.
Design a function for bubble sort in c
Design a function to swapping of two number
Design a function to check the given number is Armstrong or not if Armstrong return 1 else 0
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!