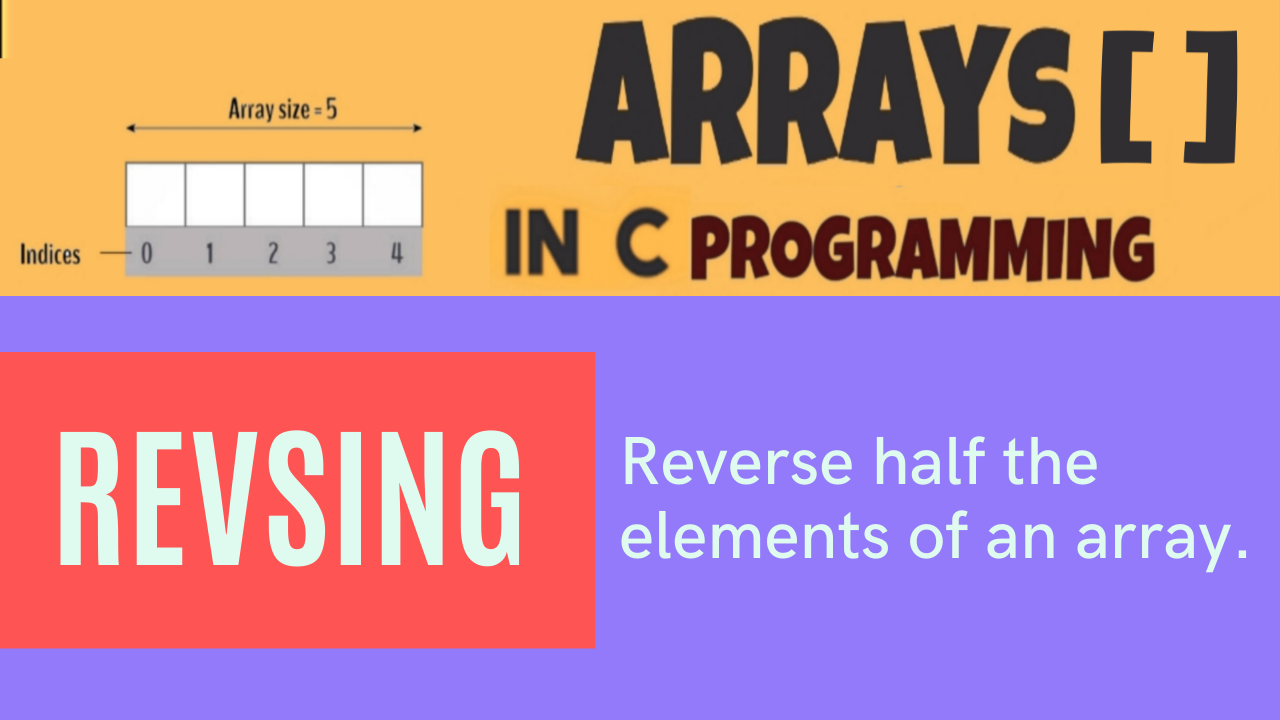
write a program to reverse half of the elements of an array.
Reverse Half of the Elements of an Array using C Programming
Write a C program to reverse half of the elements of an array" This means that you need to write a program in the C language that takes an input array and reverses half of its elements. For example, if the input array contains [1, 2, 3, 4, 5, 6]
, the program should reverse the second half of the array, resulting in [1, 2, 6, 5, 4, 3].
Input:
60 | 70 | 80 | 90 | 100 | 10 | 20 | 30 | 40 | 50 |
output:
10 | 20 | 30 | 40 | 50 | 60 | 70 | 80 | 90 | 100 |
Program 01: C language that reverses the first half of the elements of an array
#include<stdio.h>
void main()
{
int a[10],ele,i,j,t;
ele=sizeof(a)/sizeof(a[0]);
printf("enter the lelements of an array:\n");
for(i=0;i<ele;i++)//for scanning
scanf("%d",&a[i]);
printf("array elelments are:");
for(i=0;i<ele;i++)//for printing
printf(" %d",a[i]);
printf("\n");
//for(i=0,j=5;j<ele;i++,j++)//for half element reverse first logic
for(i=0,j=5;i<ele/2;i++,j++)//for half element reverse second logic
{
t=a[i];
a[i]=a[j];
a[j]=t;
}
printf("half reverse array:")
for(i=0;i<ele;i++)//half element reverse printing
printf(" %d",a[i]);
printf("\n");
}
Output
will depend on the input values of the array a. If the input values are [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
, then the output will be:
Enter the lelements of an array:
1 2 3 4 5 6 7 8 9 10
array elelments are:
1 2 3 4 5 6 7 8 9 10
half reverse array:
6 5 4 3 2 1 7 8 9 10
The code iterates over the first half of the array and swaps the elements at each index with the corresponding element in the second half of the array. This effectively reverses the order of the elements in the first half of the array. The code then prints the entire array, which results in the output above.
In general, the code will reverse the order of the elements in the first half of the array, regardless of the input values.
Program 02: C language that reverses the first half of the elements of an array Method 02
#include<stdio.h>
int main() {
// Initialize the input array
int input_arr[] = {1, 2, 3, 4, 5};
int length = sizeof(input_arr) / sizeof(int);
// Reverse half of the array
for (int i = 0; i < length / 2; i++) {
int temp = input_arr[i];
input_arr[i] = input_arr[length - i - 1];
input_arr[length - i - 1] = temp;
}
// Print the reversed array
printf("Reversed Array: ");
for (int i = 0; i < length; i++) {
printf("%d ", input_arr[i]);
}
printf("\n");
return 0;
}
Concusion
This program initializes an input array with some values and then uses a for loop to iterate over the first half of the array and reverse its elements. It does this by swapping the ith element with the (length-i-1)th
element, where i
is the loop variable. The program then prints the reversed array to the console to show the result.
Output
Reversed Array: 5 4 3 1 2
Note that in this program, we're assuming that the length of the input array is even. If the length of the input array is odd, the middle element will be left unchanged.
Top Resources
Copy in a reverse manner in c
Write a program to reverse printing not reversing of 10 elements of array in c
Write a program to reverse the bits of a given number using for loop
Design a function to reverse the given string
Design a function to reverse the word in given string
write a program to copy one array element to another array element in reverse manner.
write a program to reverse adjacent elements of an array.
write a program to reverse half of the elements of an array.
Further Reading:
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!