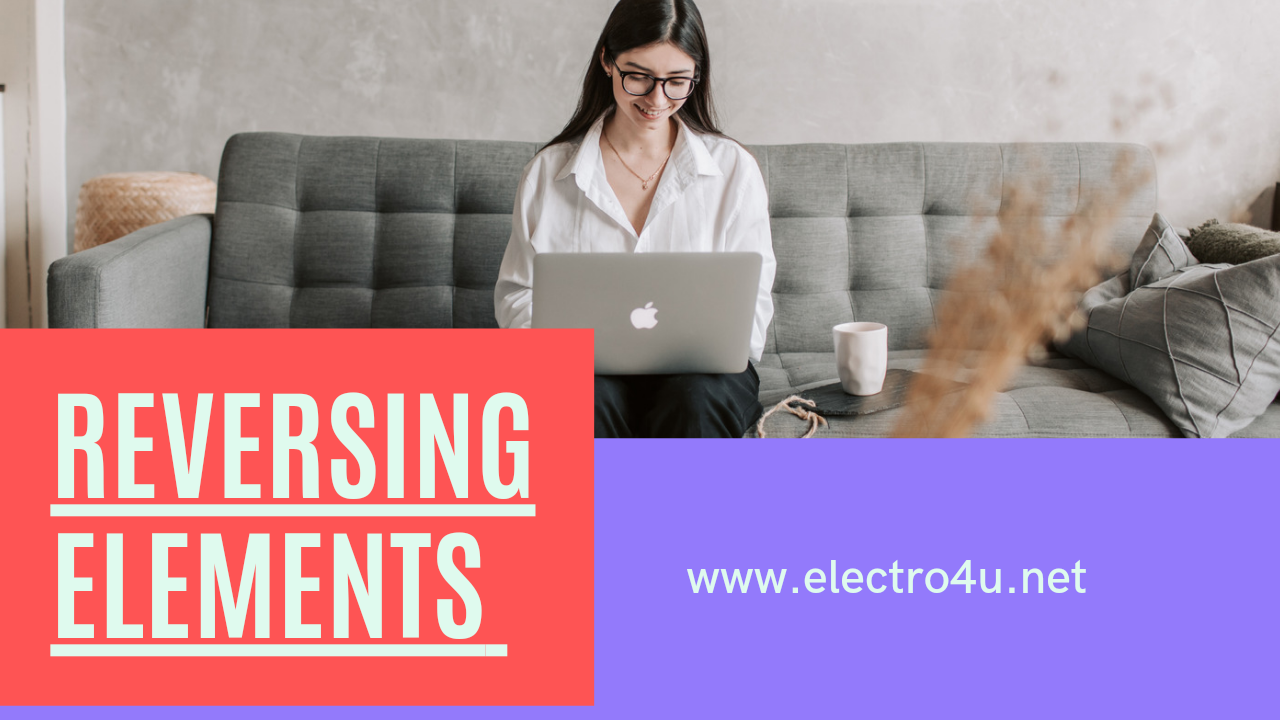
Write a c program for reversing of an array ( original array element reverse ).
Array Reversal Using C: A Practical Example
To write a C program for reversing the elements of an array, we can use the following steps:
- Declare an array and initialize it with the elements to be reversed.
- Declare two integer variables, i and j, to iterate over the array.
- Initialize i to 0 and j to the size of the array minus 1.
- While i is less than or equal to j:
- Swap the elements at indices i and j.
- Increment i by 1.
- Decrement j by 1.
- Print the reversed array.
Reverse Array in C: A Simple Program
C Programming
#include <stdio.h>
int main() {
// Declare an array and initialize it with the elements to be reversed.
int arr[] = {1, 2, 3, 4, 5};
// Declare two integer variables, i and j, to iterate over the array.
int i, j;
// Initialize i to 0 and j to the size of the array minus 1.
i = 0;
j = sizeof(arr) / sizeof(arr[0]) - 1;
// While i is less than or equal to j:
while (i <= j) {
// Swap the elements at indices i and j.
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
// Increment i by 1.
i++;
// Decrement j by 1.
j--;
}
// Print the reversed array.
printf("The reversed array is: ");
for (i = 0; i < sizeof(arr) / sizeof(arr[0]); i++) {
printf("%d ", arr[i]);
}
printf("\n");
return 0;
}
Output:
The reversed array is: 5 4 3 2 1
Top Resources
Copy in a reverse manner in c
Write a program to reverse printing not reversing of 10 elements of array in c
Write a program to reverse the bits of a given number using for loop
Design a function to reverse the given string
Design a function to reverse the word in given string
write a program to copy one array element to another array element in reverse manner.
write a program to reverse adjacent elements of an array.
write a program to reverse half of the elements of an array.
Further Reading:
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!