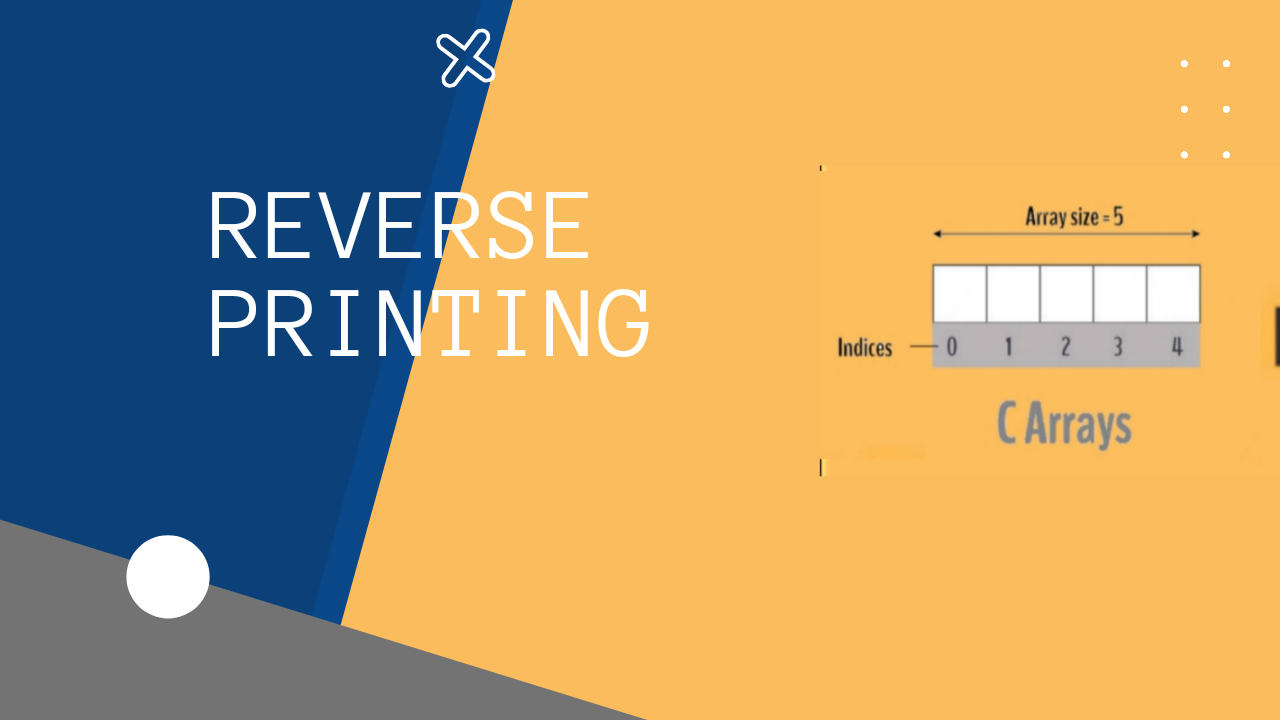
write a c program to reverse printing of an array | electro4u
Reverse Printing an Array in C ( only reverse printing not disturb the array original elements).
To reverse print an array in C without disturbing the original elements, we can use the following steps:
- Create a temporary array of the same size as the original array.
- Copy the elements of the original array to the temporary array in reverse order.
- Print the elements of the temporary array.
Input:
10 | 20 | 30 | 40 | 50 |
Output:
50 | 40 | 30 | 20 | 10 |
The following code shows how to implement these steps:
C programming
#include <stdio.h>
void reverse_print_array(int arr[], int size) {
int temp[size];
for (int i = size - 1; i >= 0; i--) {
temp[size - i - 1] = arr[i];
}
for (int i = 0; i < size; i++) {
printf("%d ", temp[i]);
}
printf("\n");
}
int main() {
int arr[] = {1, 2, 3, 4, 5};
int size = sizeof(arr) / sizeof(arr[0]);
reverse_print_array(arr, size);
return 0;
}
Output:
5 4 3 2 1
This code works by first creating a temporary array of the same size as the original array. Then, it copies the elements of the original array to the temporary array in reverse order. Finally, it prints the elements of the temporary array.
The temporary array is used to avoid disturbing the original elements of the array.
Top Resources
Copy in a reverse manner in c
Write a program to reverse printing not reversing of 10 elements of array in c
Write a program to reverse the bits of a given number using for loop
Design a function to reverse the given string
Design a function to reverse the word in given string
write a program to copy one array element to another array element in reverse manner.
write a program to reverse adjacent elements of an array.
write a program to reverse half of the elements of an array.
Further Reading:
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!