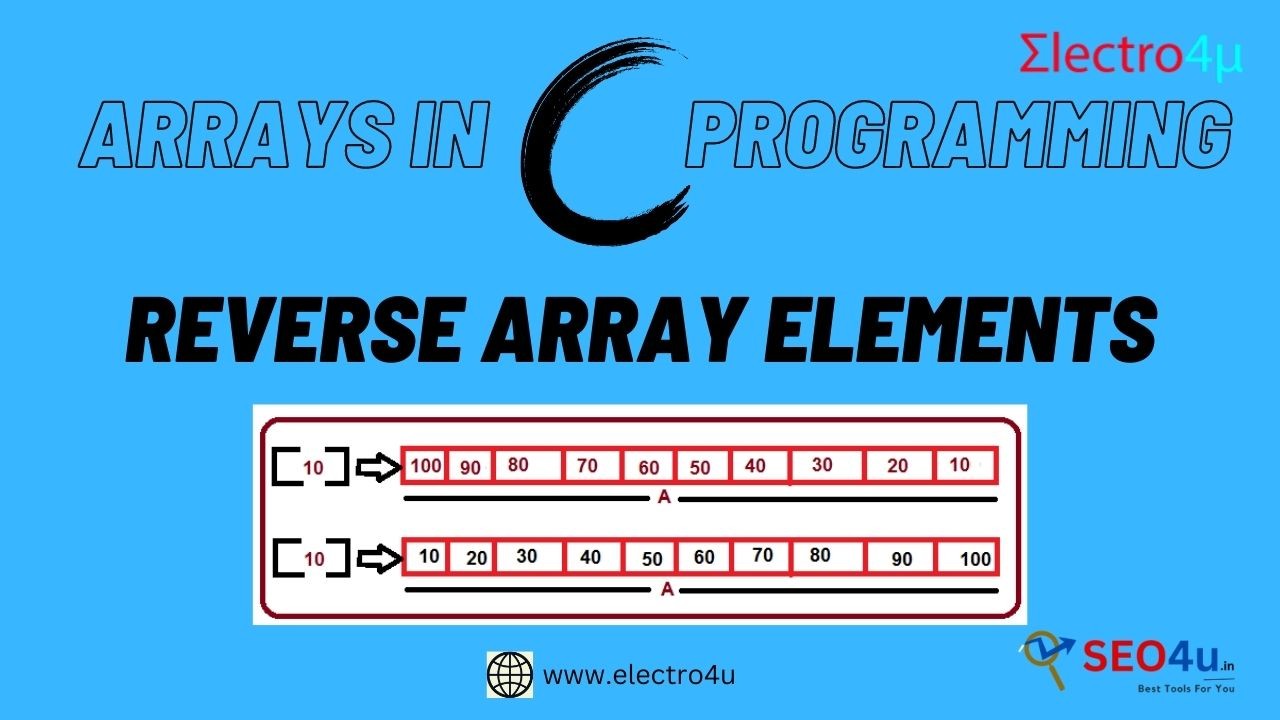
Write a program to reverse printing not reversing of 10 elements of array in c
Reverse Printing of 10 Elements of Array in C
The statement "Write a c program to reverse printing not reversing of 10 elements of array in C
" means that you should write a program that prints the elements of an array in reverse order, without actually reversing the order of the elements in the array.
For example, if the array contains the elements {1, 2, 3, 4, 5}
, the program should print the elements in the order {5, 4, 3, 2, 1}.
#include<stdio.h>
int main()
{
int a[10],i,j,t,ele;
ele=sizeof(a)/sizeof(a[0]);
printf("Enter Array:");
for(i=0;i<ele;i++)
scanf("%d",&a[i]);
printf("before:");
for(i=0;i<ele;i++)
printf(" %d",a[i]);
printf("\n");
printf("after:");
for(i=ele-1;i>=0;i--)
printf(" %d",a[i]);
printf("\n");
}
This program creates an integer array of size 10 with the values 1 to 10. It then prints the original array using a for loop that iterates through each element of the array and prints it out. After that, it prints the reversed array using another for loop that starts from the last element and goes to the first element, printing each element as it goes.
output
before: 1 2 3 4 5 6 7 8 9 10
after: 10 9 8 7 6 5 4 3 2 1
Top Resources
Copy in a reverse manner in c
Write a program to reverse printing not reversing of 10 elements of array in c
Write a program to reverse the bits of a given number using for loop
Design a function to reverse the given string
Design a function to reverse the word in given string
write a program to copy one array element to another array element in reverse manner.
write a program to reverse adjacent elements of an array.
write a program to reverse half of the elements of an array.
Further Reading:
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!