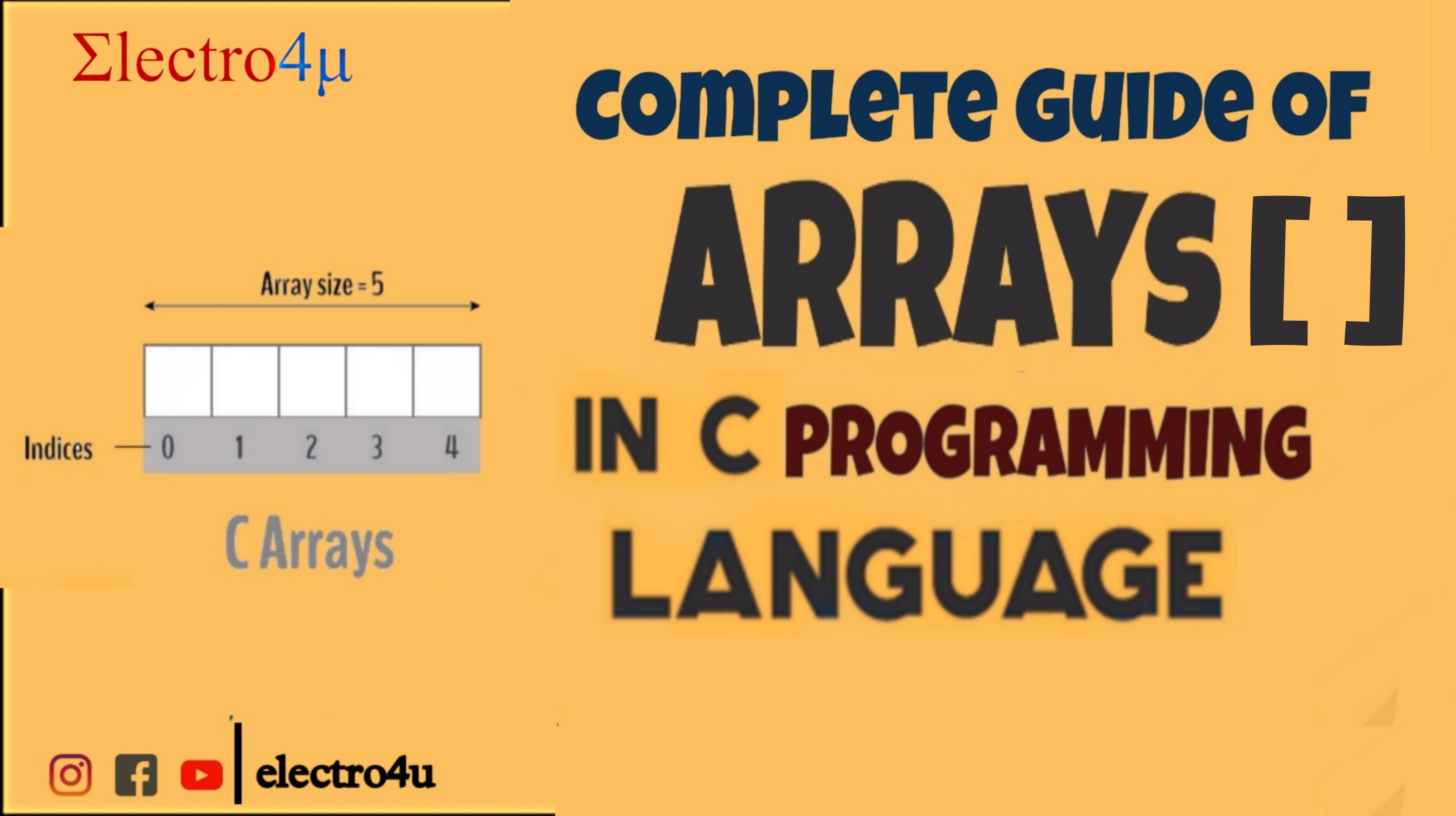
Array in c programming
What is array
An array in C programming is a data structure that stores a collection of elements of the same type. Arrays are contiguous memory locations, which means that the elements of an array are stored next to each other in memory.
To declare an array in C, you need to specify the type of the elements and the size of the array.
You can access the elements of an array using the array index operator ([]). The array index starts from 0, so the first element of the array is arr[0], the second element is arr[1], and so on.
You can initialize an array when you declare it, or you can initialize it later. To initialize an array when you declare it, you can use a comma-separated list of values. For example, the following code declares and initializes an array of 10 integers:
int arr[10] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
To initialize an array later, you can use a loop to iterate over the elements of the array and assign values to them.
For example, the following code initializes the arr array to all 0s:
int arr[10];
for (int i = 0; i < 10; i++) {
arr[i] = 0;
}
Arrays are a powerful data structure that can be used to store and manipulate data in a variety of ways. They are often used to implement other data structures, such as lists, stacks, and queues.
Here are some examples of how to use arrays in C programming:
// Declare an array of 10 floating-point numbers
float arr[10];
// Initialize the array to all 0s
for (int i = 0; i < 10; i++) {
arr[i] = 0.0f;
}
// Read 10 floating-point numbers from the user and store them in the array
for (int i = 0; i < 10; i++) {
scanf("%f", &arr[i]);
}
// Print the sum of the elements of the array
float sum = 0.0f;
for (int i = 0; i < 10; i++) {
sum += arr[i];
}
printf("The sum of the elements of the array is %f\n", sum);
This code will read 10 floating-point numbers from the user and store them in the arr array. It will then print the sum of the elements of the array.
Arrays are a versatile and powerful data structure that is used in many C programs. By understanding how to use arrays, you can write more efficient and concise code
Top Resources
how to declare and initialization an array
Dynamic Declaration of an array
How to scan an array and how to print it on screen.
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!