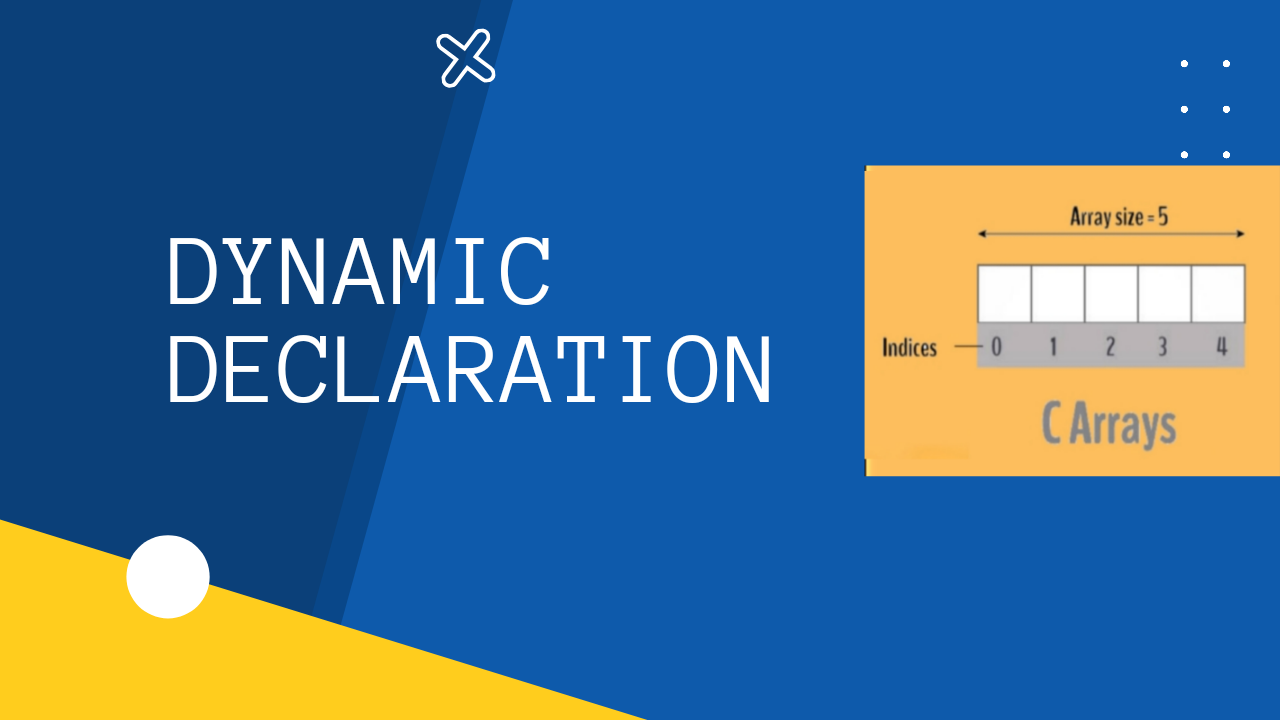
Dynamic Declaration of an array.
Dynamic Declaration of an Array in C: A Comprehensive Guide
Dynamic declaration of an array in C refers to creating an array whose size is determined during runtime, rather than at compile-time. This allows the program to allocate memory for the array based on the input or other dynamic conditions.
Dynamic declaration of an array in C is the process of allocating memory for an array at runtime, rather than at compile time. This is done using the malloc()
function, which returns a pointer to the allocated memory. The pointer can then be used to access the elements of the array.
Here is an example of how to dynamically declare an array in C:
int *array = malloc(sizeof(int) * 10); // Allocates memory for an array of 10 integers
The sizeof()
operator is used to determine the size of the array in bytes. In this case, the array is 10 integers long, so the size is 10 * sizeof(int). The malloc()
function then allocates memory for the array and returns a pointer to it.
The pointer can then be used to access the elements of the array. For example, the following code prints the value of the first element of the array:
printf("%d\n", array[0]);
It is important to free the memory allocated by malloc() when the array is no longer needed. This can be done using the free() function.
For example: free(array);
Advantages of Dynamic Arrays
- Dynamic arrays can be resized at runtime, which allows them to grow or shrink as needed.
- Dynamic arrays can be used to store any type of data.
- Dynamic arrays are not limited by the stack size, which allows them to store large amounts of data.
Disadvantages of Dynamic Arrays
- Dynamic arrays are more complex to use than fixed-size arrays.
- Dynamic arrays can waste memory if they are not resized properly.
- Dynamic arrays can be a security risk if they are not properly initialized.
Example 01: C program to dynamically declare an array
#include
void main()
{
int n;
printf("enter any number:\n");
scanf("%d",&n);
int a[n];
printf("%d\n",sizeof(a));
}
Example 02: C program to declare dynamically array using pointer
#include
#include
int main() {
int n, i;
printf("Enter the size of the array: ");
scanf("%d", &n);
int* arr = (int*) malloc(n * sizeof(int)); // dynamically declare the array
printf("Enter the elements of the array:\n");
for(i=0; i<n; i++){
scanf("%d", &arr[i]);
}
printf("The array elements are: ");
for(i=0; i<n; i++){
printf("%d ", arr[i]);
}
free(arr); // free the allocated memory
return 0;
}
In this code, the user is asked to enter the size of the array. Then, using the malloc() function, memory is allocated dynamically for the array based on the input size. The elements of the array are then scanned from the user, and finally, the elements are printed on the screen. At the end of the program, the memory allocated for the array is freed using the free() function.
Note that the size of the array can be changed dynamically based on any other condition as well.
Conclusion
Dynamic arrays are a powerful tool that can be used to store large amounts of data and to resize arrays at runtime. However, they are more complex to use than fixed-size arrays and can be a security risk if they are not properly initialized.
Further Reading:
write a c program to reverse the elements of a given array.
write a c program to delete an element at desired from an array
write a c program to insert an element at a desired position in an array
write a c program that deletes the duplicate elements of an array.
write a c program to find the duplicate elements of a given array and find the count of duplicate elements.
write a c program to print non-repeated numbers of a given array.
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!