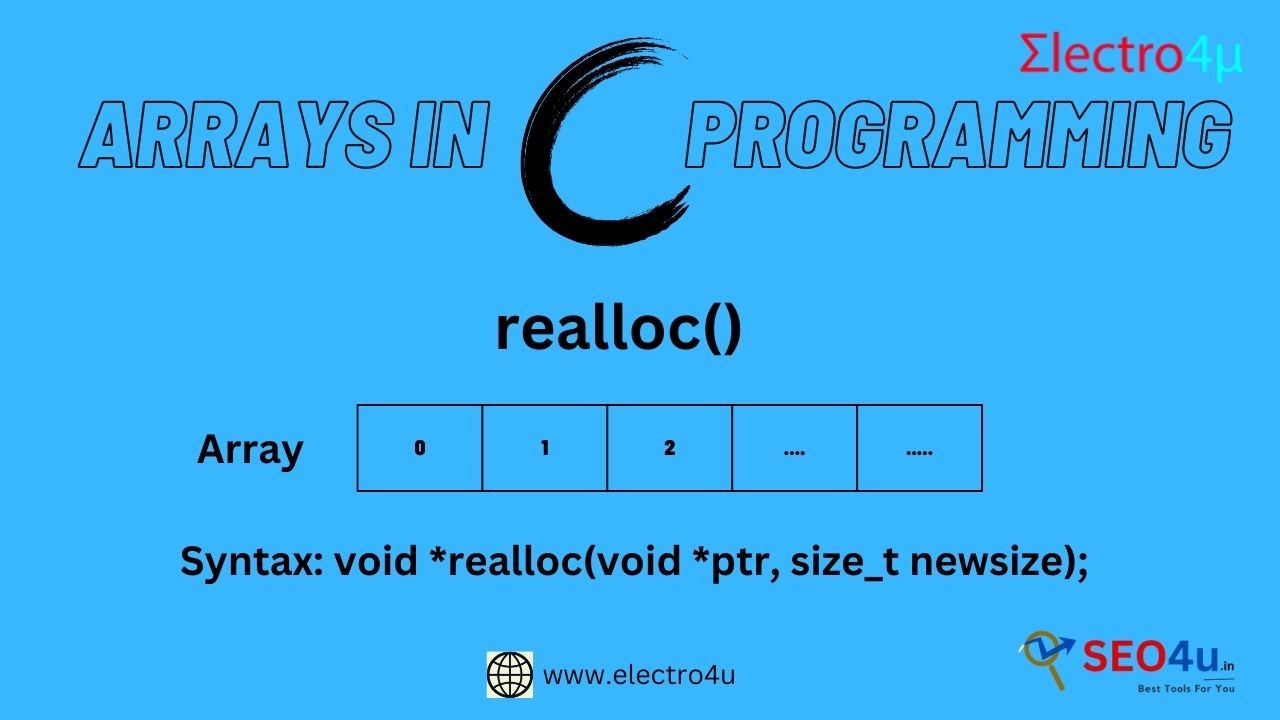
What is the use of realloc function in c programming.
Understanding the realloc() Function in C Programming
Introduction
The realloc() function is a powerful tool in the C programming language that allows for dynamic memory allocation. It enables you to resize previously allocated memory blocks, providing flexibility and efficiency in memory management. This function is particularly useful when you need to adjust the size of an array or structure to accommodate changing data requirements during program execution.
Key Benefits
1. Dynamic Memory Management
realloc() empowers developers to dynamically adjust the size of memory blocks at runtime. This means that you can allocate memory based on the specific needs of your program, rather than relying on fixed, static allocations.
2. Efficient Memory Utilization
By reallocating memory as needed, you can optimize memory usage and prevent unnecessary wastage. This is especially crucial when dealing with large datasets or when the size of data structures may vary over time.
3. Avoiding Data Loss
When resizing memory using realloc(), the function takes care of preserving existing data. It ensures that any information stored in the original memory block is retained, even after resizing. This is a critical feature for maintaining data integrity.
How to Use realloc()
Using realloc() involves a few key steps:
-
Include the <stdlib.h> Header File: To access the realloc() function, include the standard library header file.
#include <stdlib.h>
-
Allocate Initial Memory: Begin by allocating memory using functions like malloc() or calloc().
int *ptr = (int *)malloc(5 * sizeof(int));
-
Use realloc() to Resize: When the need arises, call realloc() to adjust the size of the allocated memory block.
ptr = (int *)realloc(ptr, 10 * sizeof(int));
-
Check for NULL: Always check the return value of realloc() to ensure that the memory allocation was successful.
if (ptr == NULL) { // Handle memory allocation failure }
Best Practices
-
Error Handling: Always check if realloc() returns NULL to handle potential memory allocation failures gracefully.
-
Avoid Memory Leaks: Keep track of allocated memory and free it when it is no longer needed to prevent memory leaks.
-
Avoid Excessive Calls: Frequent calls to realloc() can lead to inefficiencies. Consider allocating slightly more memory than initially needed to reduce the frequency of reallocation.
-
Reassign Pointers: After calling realloc(), remember to assign the returned pointer back to the original variable.
Conclusion
The realloc() function is a valuable tool for managing memory dynamically in C programming. By allowing you to resize memory blocks on-demand, it provides flexibility and efficiency in memory allocation. Understanding how to use realloc() and following best practices will help you leverage this function effectively in your programs.
For further information and examples, Please visit C-Programming From Scratch to Advanced 2023-2024