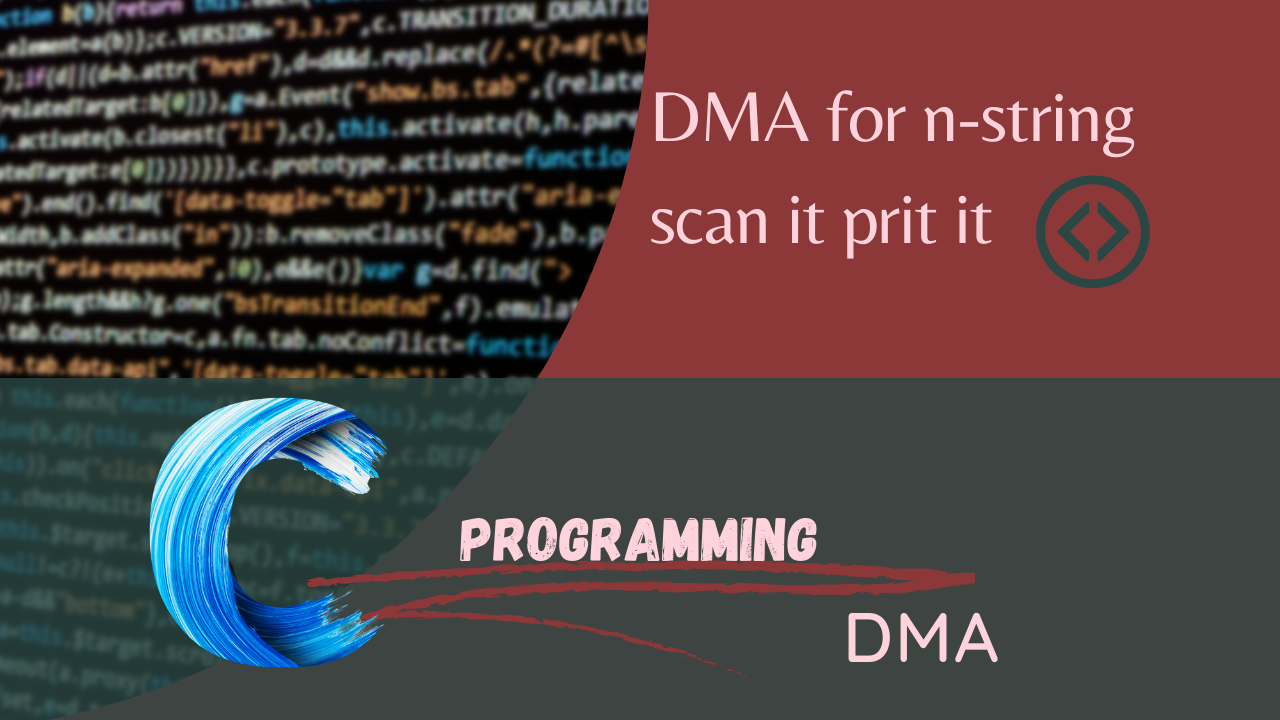
Write a c program to allocate a dynamic memory for n string
Allocating dynamic memory for n strings means that you are creating memory space in the computer's RAM for n number of strings at runtime. Dynamic memory allocation allows you to allocate memory for a variable number of strings or a variable amount of memory. This is in contrast to static memory allocation, where memory is allocated at compile-time and is fixed in size.
In a C program, you can allocate dynamic memory for n strings by using the malloc function, which allocates a block of memory of a specified size and returns a pointer to the first byte of the block. To allocate memory for n strings, you can create an array of pointers to strings (i.e., an array of char * pointers), and then use malloc to allocate memory for each individual string. The following code snippet demonstrates how to allocate dynamic memory for n strings:
int n;
char **strings; // declare a pointer to a pointer to char
// get the number of strings from the user
printf("Enter the number of strings: ");
scanf("%d", &n);
// allocate memory for the array of pointers
strings = (char **) malloc(n * sizeof(char *));
// allocate memory for each individual string
for (int i = 0; i < n; i++) {
strings[i] = (char *) malloc(MAX_STRING_LENGTH * sizeof(char));
}
In the code above, MAX_STRING_LENGTH is a constant that defines the maximum length of each string. You can adjust this value based on your needs. After allocating memory for the strings, you can use them as needed in your program. Don't forget to deallocate the memory using free when you're done with it to avoid memory leaks.
#include<stdio.h>
#include<stdlib.h>
int main()
{
char **p;
int i,n;
printf("Enter n String:");
scanf("%d",&n);
p=malloc(sizeof(char*)*n);
for(i=0;i<n;i++)
p[i]=malloc(sizeof(char)*10);
printf("Enter the string:");
for(i=0;i<n;i++)
scanf("%s",p[i]);
for(i=0;i<n;i++)
printf("%s\n",p[i]);
}
Output:
Enter n String: 2
Enter the string: balmiki kumar
balmiki kumar