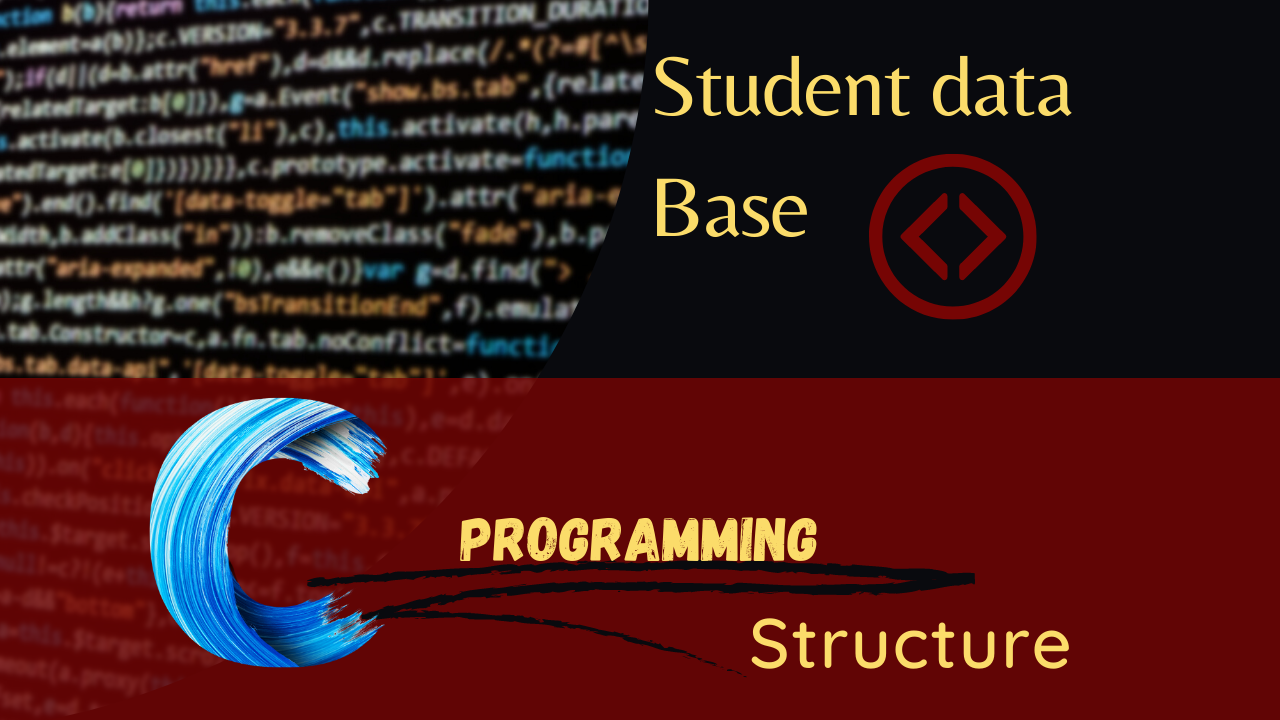
Write a c program to declare student data base structure which contains three members , roll number,name, total marks
"Write a C program to declare student database structure which contains three members - roll number, name, total marks" means that you need to write a C program that declares a structure called "student database" or "student record" with three members or fields:
- Roll number - an integer that uniquely identifies each student in the database.
- Name - a character array that stores the name of the student.
- Total marks - an integer that stores the total marks obtained by the student.
The program should declare this structure and also provide a way to store and retrieve values for each of the three members of the structure. This can be done using variables of the structure type, and accessing the members using dot notation. The program should also provide any necessary input/output statements to interact with the user or display the values of the members.
Program 01: for 1 student
#include
struct st
{
int roll_num;
char name[10];
float t_marks;
};
void main()
{
struct st s1;
printf("Enter the student roll_num:");
scanf("%d",&s1.roll_num);
printf("Enter the student name:");
scanf("%s",&s1.name);
printf("Enter the student total marks:");
scanf("%f",&s1.t_marks);
printf("%d %s %f\n",s1.roll_num,s1.name,s1.t_marks);
}
Output:
Enter the student roll_num: 101
Enter the student name: Balmiki
Enter the student's total marks: 95.3
101 Balmiki 95.300003
Program 02: for 5 Students
#include
struct st
{
int roll_num;
char name[10];
float t_marks;
};
void main()
{
struct st s[5];
int i,ele;
ele=sizeof(s)/sizeof(s[0]);
for(i=0;i<ele;i++){
printf("Enter the student roll_num:");
scanf("%d",&s[i].roll_num);
printf("Enter the student name:");
scanf("%s",&s[i].name);
printf("Enter the student total marks:");
scanf("%f",&s[i].t_marks);
}
for(i=0;i<ele;i++)
printf("%d %s %f\n",s[i].roll_num,s[i].name,s[i].t_marks);
}
Output:
Enter the student roll_num:90
Enter the student name: Balmiki
Enter the student's total marks:95.5
Enter the student roll_num:91
Enter the student name: Swathi
Enter the student's total marks:99.9
Enter the student roll_num:92
Enter the student name: sunethra
Enter the student's total marks:99.8
Enter the student roll_num:93
Enter the student name: Deepraj
Enter the student's total marks:100
Enter the student roll_num:94
Enter the student name: Manjula
Enter the student's total marks:98.8
90 Balmiki 95.500000
91 swathi 99.900002
92 sunethra 99.800003
93 Deepraj 100.000000
94 Manjula 98.800003