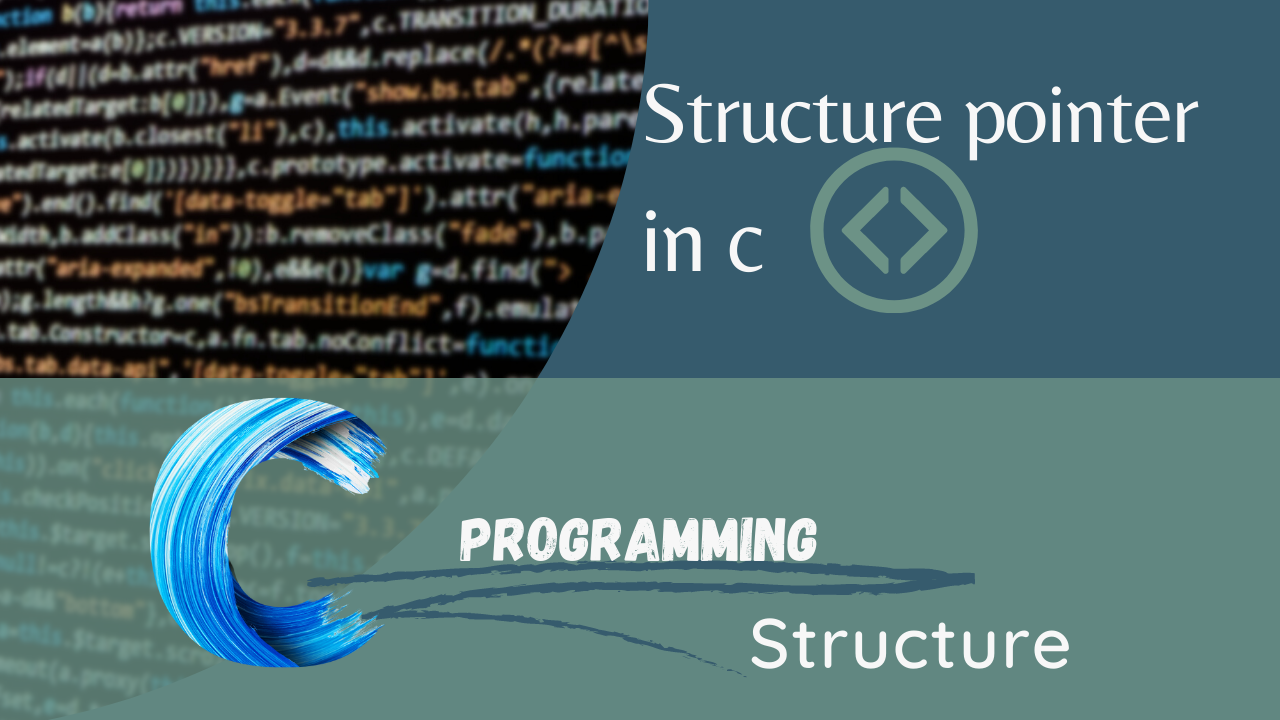
Structure pointer in c
Mastering Structure Pointers: A Comprehensive Guide in C
A structure pointer in C is a pointer that points to a structure variable. It is used to access and modify the members of the structure variable. Structure pointers are useful for passing structures to functions and for creating linked lists and other complex data structures.
To declare a structure pointer, you use the same syntax as for declaring any other pointer, but you prefix the pointer type with the struct keyword. For example, the following code declares a structure pointer to a structure named Person:
struct Person {
char name[20];
int age;
};
struct Person *personPtr;
To initialize a structure pointer, you can use the address-of operator (&) to get the address of a structure variable. For example, the following code initializes the personPtr pointer to point to the person1 structure variable:
struct Person person1 = {"John Doe", 30};
personPtr = &person1;
Once a structure pointer is initialized, you can use it to access and modify the members of the structure variable. To do this, you use the arrow operator (->). For example, the following code prints the name of the person pointed to by the personPtr pointer:
printf("%s\n", personPtr->name);
You can also use structure pointers to pass structures to functions. For example, the following code defines a function called printPerson() that takes a structure pointer to a Person structure as an argument:
void printPerson(struct Person *person) {
printf("%s, %d\n", person->name, person->age);
}
To call the printPerson() function, you pass it the address of a Person structure variable. For example, the following code calls the printPerson() function to print the information of the person1 structure variable:
printPerson(&person1);
Structure pointers are a powerful tool that can be used to create complex data structures and to write efficient and reusable code.
Examples of how to use structure pointers in C:
Create a linked list: To create a linked list, you can use a structure pointer to point to the next element in the list. For example, the following code defines a linked list node:
struct Node {
int data;
struct Node *next;
};
You can then use a structure pointer to create a linked list of nodes:
struct Node *head = NULL;
// Add the first node to the list
struct Node *node1 = malloc(sizeof(struct Node));
node1->data = 10;
node1->next = NULL;
head = node1;
// Add a second node to the list
struct Node *node2 = malloc(sizeof(struct Node));
node2->data = 20;
node2->next = NULL;
node1->next = node2;
// Print the linked list
struct Node *current = head;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
Pass a structure to a function: You can pass a structure to a function by passing the address of the structure variable to the function. For example, the following code defines a function called sum() that takes two structure pointers to Person structures as arguments and returns the sum of their ages:
int sum(struct Person *person1, struct Person *person2) {
return person1->age + person2->age;
}
To call the sum() function, you would pass it the addresses of two Person structure variables:
int sum = sum(&person1, &person2);
printf("The sum of the ages is %d\n", sum);
Structure pointers are a powerful tool that can be used to write efficient and reusable code in C.
Further Reading:
What is self-referential structure pointer in c?
Structure pointer in c
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!