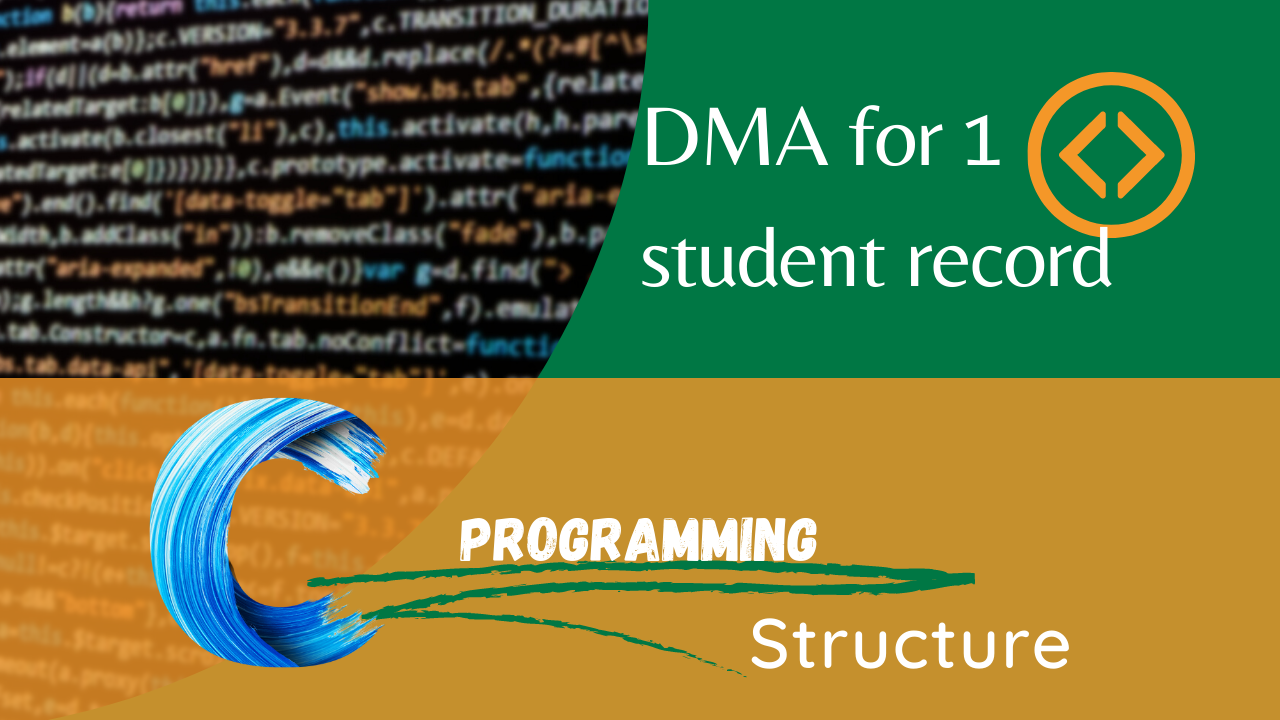
Write a c program to allocate a dynamic memory for 1 student record it and scan it and print it
C Program: Allocating Dynamic Memory for a 1 Student Record
To write a C program to allocate dynamic memory for 1 student record, scan it, and print it, we can use the following steps:
- Declare a struct to store the student record.
- Allocate dynamic memory for the student record using the malloc() function.
- Scan the student record from the user.
- Print the student record.
Here is a sample code:
C Programming
#include <stdio.h>
#include <stdlib.h>
struct Student {
char name[50];
int age;
int marks;
};
int main() {
// Declare a struct to store the student record
struct Student *student;
// Allocate dynamic memory for the student record
student = (struct Student *)malloc(sizeof(struct Student));
// Scan the student record from the user
printf("Enter the student's name: ");
scanf("%s", student->name);
printf("Enter the student's age: ");
scanf("%d", &student->age);
printf("Enter the student's marks: ");
scanf("%d", &student->marks);
// Print the student record
printf("\nStudent record:\n");
printf("Name: %s\n", student->name);
printf("Age: %d\n", student->age);
printf("Marks: %d\n", student->marks);
// Free the allocated memory
free(student);
return 0;
}
Output:
Enter the student's name: John Doe
Enter the student's age: 18
Enter the student's marks: 90
Student record:
Name: John Doe
Age: 18
Marks: 90
Further Reading:
Dynamic Memory allocation or Run time memory allocation in c
What is static memory allocation and dynamic memory allocation?
Write a c program to allocate a dynamic memory for 1 student record it and scan it and print it
Write a c program to allocate dynamic memory for 5 integers scan it and print it
write a c program Allocate a dynamic memory for n students record it scan it and print it using structure
write a c program to allocate dynamic memory for n integers scan it and print n value takes it run times
write a c program to allocate dynamic memory for one string scan it print it
write a c program to allocate dynamic memory for three string scan it and print it
Write a c program to allocate a dynamic memory for n string
c program to allocate dynamic memory for 2D array integers where a number of rows and numbers of columns scan from the user at run time,
write a c program to allocate a dynamic memory for three student record scan it and print it using structure
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!