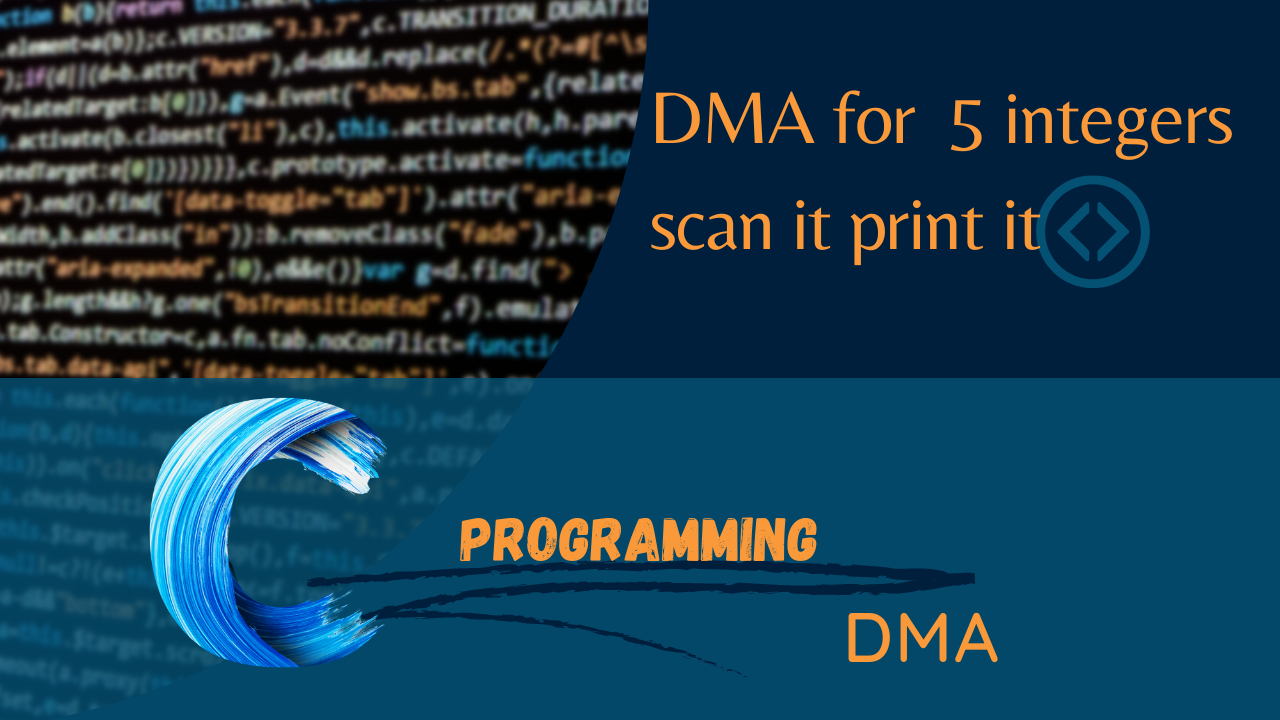
Write a c program to allocate dynamic memory for 5 integers scan it and print it
C Program: Allocating Memory for 5 Integers Dynamically
To allocate dynamic memory for 5
integers in C, you can use the malloc()
function. The malloc()
function takes the size of the memory block to be allocated in bytes as an argument and returns a pointer to the allocated memory block.
C program allocates dynamic memory for 5 integers, scans them from the user, and prints them:
C Programming
#include <stdio.h>
#include <stdlib.h>
int main() {
// Allocate dynamic memory for 5 integers
int *ptr = malloc(5 * sizeof(int));
// Check if memory allocation was successful
if (ptr == NULL) {
printf("Unable to allocate memory\n");
exit(1);
}
// Scan the integers from the user
printf("Enter 5 integers: ");
for (int i = 0; i < 5; i++) {
scanf("%d", &ptr[i]);
}
// Print the integers
printf("The integers are: ");
for (int i = 0; i < 5; i++) {
printf("%d ", ptr[i]);
}
printf("\n");
// Free the allocated memory
free(ptr);
return 0;
}
Output:
Enter 5 integers: 1 2 3 4 5
The integers are: 1 2 3 4 5
Further Reading:
Dynamic Memory allocation or Run time memory allocation in c
What is static memory allocation and dynamic memory allocation?
Write a c program to allocate dynamic memory for 5 integers scan it and print it
write a c program to allocate dynamic memory for n integers scan it and print n value takes it run times
write a c program to allocate dynamic memory for one string scan it print it
write a c program to allocate dynamic memory for three string scan it and print it
Write a c program to allocate a dynamic memory for n string
c program to allocate dynamic memory for 2D array integers where a number of rows and numbers of columns scan from the user at run time,
Write a c program to allocate a dynamic memory for 1 student record it and scan it and print it
write a c program to allocate a dynamic memory for three student record scan it and print it using structure
write a c program Allocate a dynamic memory for n students record it scan it and print it using structure
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!