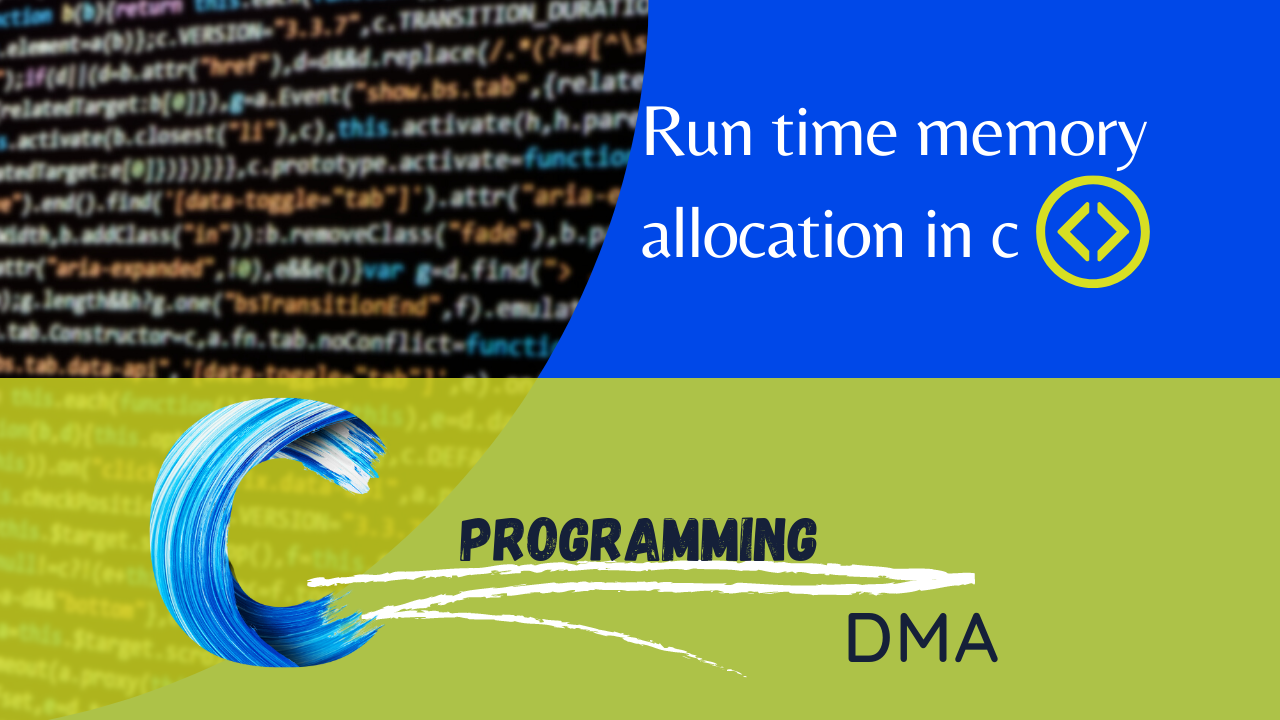
Dynamic Memory allocation or Run time memory allocation in c
Mastering Dynamic Memory Allocation in C Programming
Dynamic memory allocation, also known as run-time memory allocation, is a programming technique that allows programmers to allocate memory at run time. This is in contrast to static memory allocation, where memory is allocated at compile time.
Syntax of Dynamic memory allocation
Dynamic memory allocation is useful for a variety of purposes, such as:
- Allocating memory for data structures of unknown size, such as linked lists and trees.
- Allocating memory for temporary data structures, such as buffers and stacks.
- Reallocating memory for existing data structures to make them larger or smaller.
Dynamic memory allocation in C is performed using the following standard library functions:
- malloc(): Allocates a block of memory of the specified size in bytes.
- calloc(): Allocates a block of memory of the specified size in bytes and initializes all of the bytes to zero.
- free(): Frees the memory allocated by malloc() or calloc().
- realloc(): Reallocates a block of memory to a new size.
Example of how to use dynamic memory allocation in C to allocate memory for an array of integers:
#include <stdlib.h>
int main() {
// Allocate memory for an array of 10 integers
int* arr = (int*)malloc(10 * sizeof(int));
// Initialize the array elements
for (int i = 0; i < 10; i++) {
arr[i] = i;
}
// Print the array elements
for (int i = 0; i < 10; i++) {
printf("%d ", arr[i]);
}
printf("\n");
// Free the memory allocated by malloc()
free(arr);
return 0;
}
Output:
0 1 2 3 4 5 6 7 8 9
It is important to note that when using dynamic memory allocation, it is important to free the allocated memory when it is no longer needed. This is to prevent memory leaks, which can occur when allocated memory is not freed and is no longer accessible by the program.
Some tips for using dynamic memory allocation safely:
- Always free the memory allocated by malloc() or calloc() when it is no longer needed.
- Use realloc() to resize existing data structures instead of allocating new memory and freeing the old memory.
- Check the return value of malloc(), calloc(), and realloc() to make sure that the memory allocation was successful.
- Use Valgrind to detect memory leaks and other memory-related errors.
Further Reading:
Dynamic Memory allocation or Run time memory allocation in c
What is static memory allocation and dynamic memory allocation?
Write a c program to allocate dynamic memory for 5 integers scan it and print it
write a c program to allocate dynamic memory for n integers scan it and print n value takes it run times
write a c program to allocate dynamic memory for one string scan it print it
write a c program to allocate dynamic memory for three string scan it and print it
Write a c program to allocate a dynamic memory for n string
c program to allocate dynamic memory for 2D array integers where a number of rows and numbers of columns scan from the user at run time,
Write a c program to allocate a dynamic memory for 1 student record it and scan it and print it
write a c program to allocate a dynamic memory for three student record scan it and print it using structure
write a c program Allocate a dynamic memory for n students record it scan it and print it using structure
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!