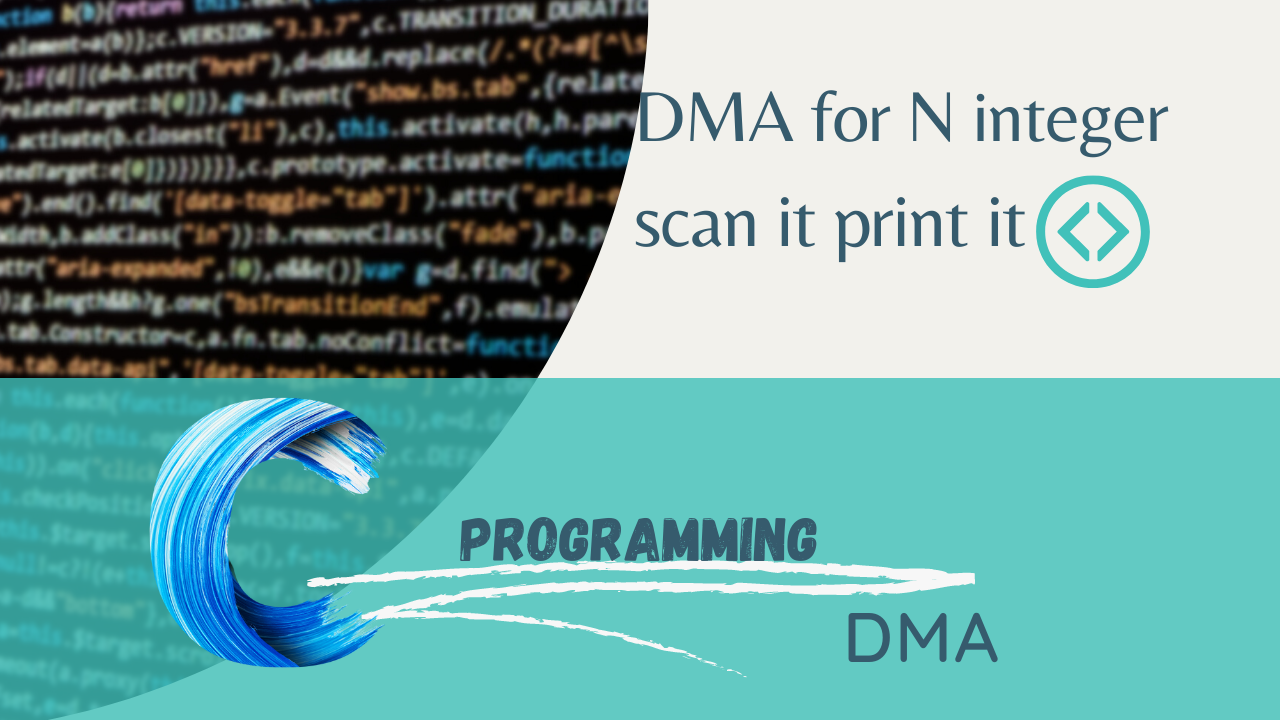
write a c program to allocate dynamic memory for n integers scan it and print n value takes it run times
Allocating Dynamic Memory for n Integers in C: A Practical Example
To write a C program to allocate dynamic memory for n integers, scan them, and print them, we can use the following steps:
- Declare two integer variables,
n
and*ptr
. - Prompt the user to enter the number of integers to allocate memory for.
- Allocate dynamic memory for n integers using the
malloc()
function. - Check the return value of
malloc()
to make sure that the memory was allocated successfully. - Prompt the user to enter the values of the integers.
- Scan the integer values into the dynamically allocated memory using the
scanf()
function. - Print the values of the integers.
Free
the dynamically allocated memory using thefree()
function.
C program that implements these steps:
C Programming
#include <stdio.h>
#include <stdlib.h>
int main() {
int n, *ptr;
// Prompt the user to enter the number of integers to allocate memory for.
printf("Enter the number of integers to allocate memory for: ");
scanf("%d", &n);
// Allocate dynamic memory for n integers using the malloc() function.
ptr = (int *)malloc(n * sizeof(int));
// Check the return value of malloc() to make sure that the memory was allocated successfully.
if (ptr == NULL) {
printf("Error: Could not allocate memory.\n");
return 1;
}
// Prompt the user to enter the values of the integers.
printf("Enter the values of the integers:\n");
for (int i = 0; i < n; i++) {
scanf("%d", ptr + i);
}
// Print the values of the integers.
printf("The values of the integers are:\n");
for (int i = 0; i < n; i++) {
printf("%d ", ptr[i]);
}
printf("\n");
// Free the dynamically allocated memory using the free() function.
free(ptr);
return 0;
}
Example output:
Enter the number of integers to allocate memory for: 5
Enter the values of the integers:
10
20
30
40
50
The values of the integers are:
10 20 30 40 50
Further Reading:
Dynamic Memory allocation or Run time memory allocation in c
What is static memory allocation and dynamic memory allocation?
Write a c program to allocate dynamic memory for 5 integers scan it and print it
write a c program to allocate dynamic memory for n integers scan it and print n value takes it run times
write a c program to allocate dynamic memory for one string scan it print it
write a c program to allocate dynamic memory for three string scan it and print it
Write a c program to allocate a dynamic memory for n string
c program to allocate dynamic memory for 2D array integers where a number of rows and numbers of columns scan from the user at run time,
Write a c program to allocate a dynamic memory for 1 student record it and scan it and print it
write a c program to allocate a dynamic memory for three student record scan it and print it using structure
write a c program Allocate a dynamic memory for n students record it scan it and print it using structure
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!