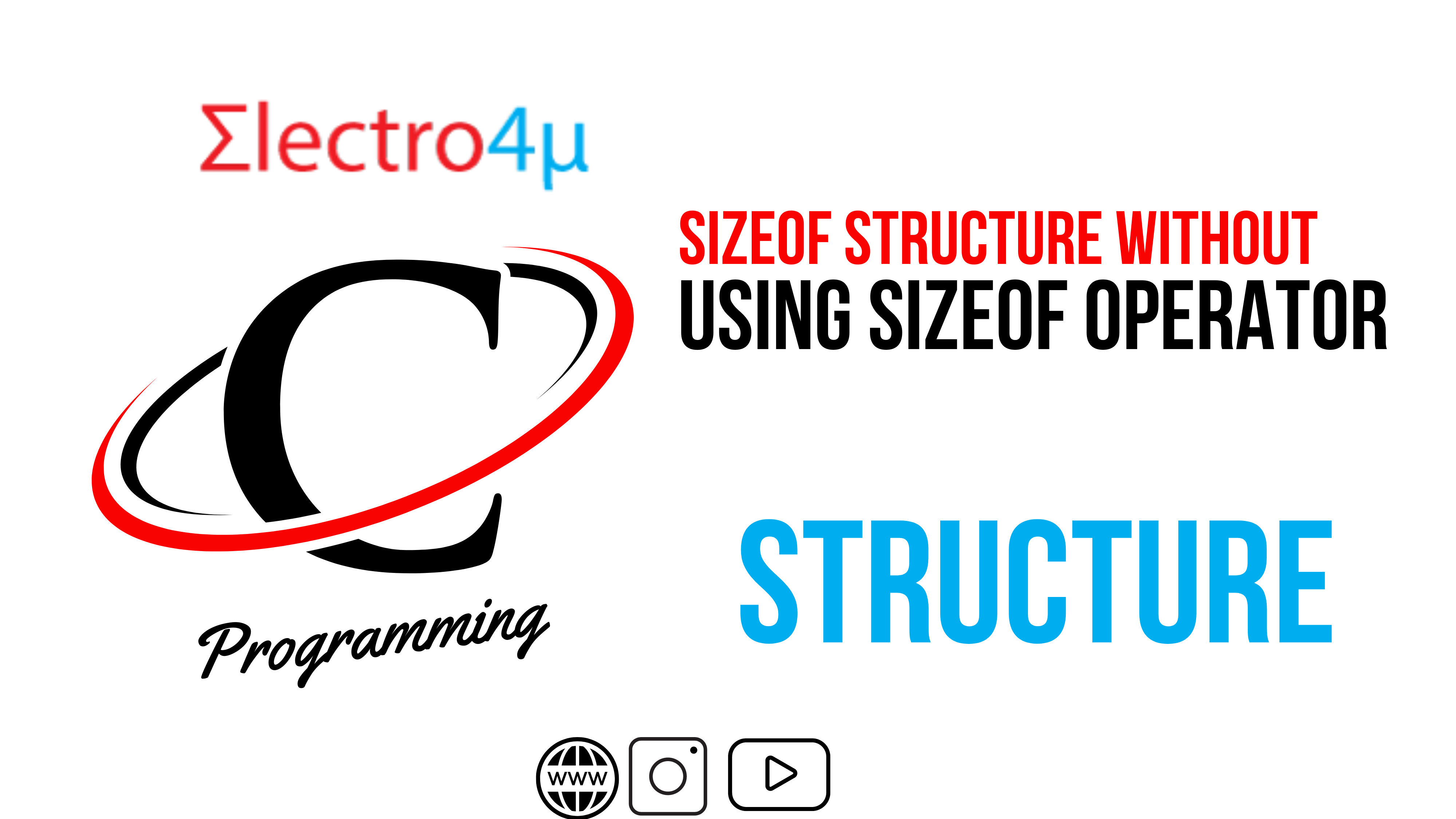
Write a program to find the sizeof structure without using sizeof operator?
How to Find the Size of a Structure in C Without Using the sizeof Operator
To find the size of a structure without using the sizeof operator in C, we can use a pointer arithmetic approach. The idea is to create a pointer to the structure and then calculate the difference between the address of the first member of the structure and the address of the next member.
Example 01: Program that demonstrates this approach sizeof Operator
#include<stdio.h>
struct sample {
int a;
char b;
float c;
};
int main() {
struct sample s;
struct sample *ptr = &s;
struct sample *ptr_next = ptr + 1;
int size = (char*)ptr_next - (char*)ptr;
printf("Size of structure is %d bytes\n", size);
return 0;
}
In this program, we define a structure named sample that has three members: an integer a, a character b, and a float c. In the main() function, we create an instance of the sample structure named s. We then create a pointer to s named ptr, and a pointer to the next member of s named ptr_next.
Next, we use pointer arithmetic to calculate the size of the structure. We cast the pointers to char* types and calculate the difference between their addresses. This gives us the size of the structure in bytes.
Finally, we print the size of the structure using printf() function.
Size of structure is 12 bytes
Note that this approach assumes that the members of the structure are packed tightly together, with no padding bytes between them. If the structure has padding bytes, this approach will not give the correct size.
Example 02: C program to find the size of a structure without using the sizeof operator:
#include<stdio.h>
struct student {
int roll;
char name[100];
float marks;
};
int main() {
struct student s;
struct student *ptr = &s;
// Calculate the size of the structure by subtracting the addresses of two consecutive elements of the array.
int size = (char *)ptr + sizeof(struct student) - (char *)ptr;
printf("The size of the structure is %d bytes.\n", size);
return 0;
}
Conclusion
This program first defines a structure called a student with three members: an integer roll, a character array name, and a float marks. It then creates a variable of type struct student called s and a pointer to struct student called ptr.
The main function then calculates the size of the structure by subtracting the addresses of two consecutive elements of the array. The first element is the address of ptr, and the second element is the address of ptr plus the size of the structure. The difference between these two addresses is the size of the structure.
Finally, the program prints the size of the structure to the console.
output of this program:
The size of the structure is 24 bytes.
The size of the structure in this example is 24 bytes because the integer roll is 4 bytes, the character array name is 100 bytes, and the float marks is 4 bytes. The padding between the members of the structure is not taken into account.
Top Recources
- Learn-C.org
- C Programming at LearnCpp.com
- GeeksforGeeks - C Programming Language
- C Programming on Tutorialspoint
- Codecademy - Learn C
- CProgramming.com