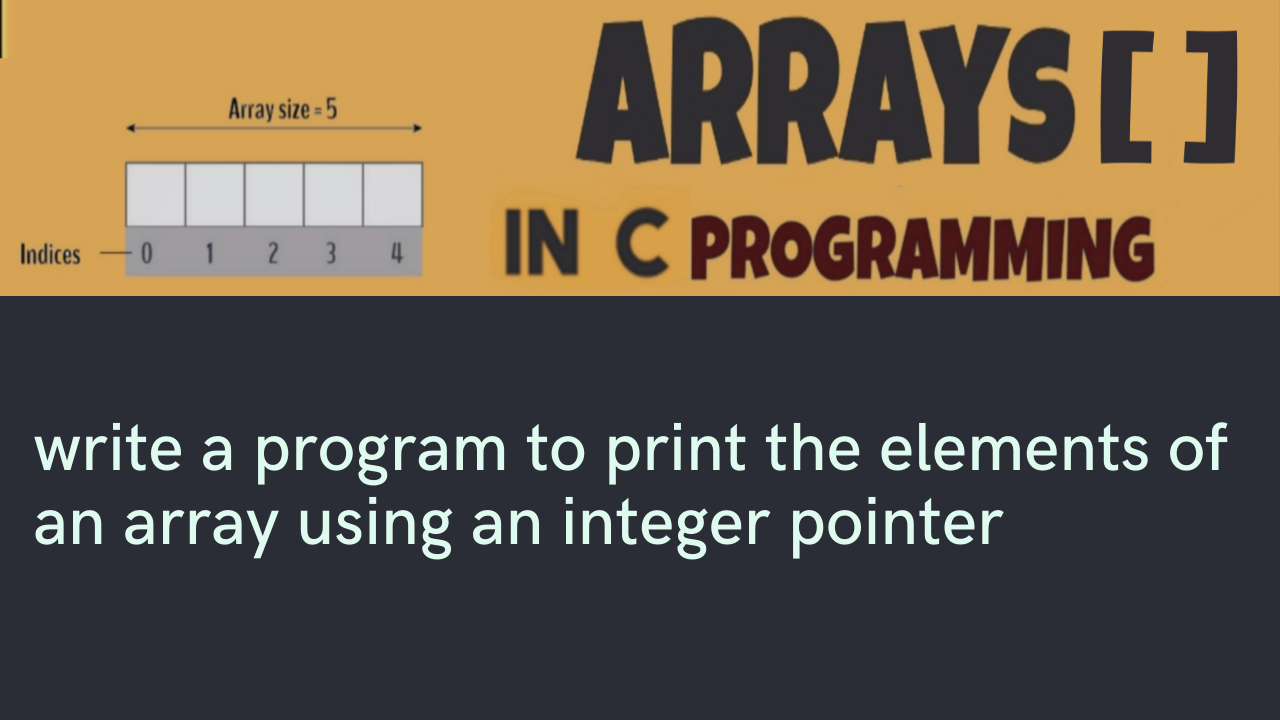
write a program to print the elements of an array using an integer pointer.
Print the Elements of an Array Using an Integer Pointer in C
"Write a C program to print the elements of an array using an integer pointer"
means that you need to write a program in the C programming language that prints out the elements of an integer array using a pointer.
To do this, you need to declare an integer array and its size. Then, create a pointer that points to the first element of the array. You can then use a for loop to iterate through the array and print out its elements using pointer notation. The pointer notation dereferences the pointer to get the value at the current index, and the + i part increments the pointer to point to the next element in the array.
Printing out the elements of an array using a pointer is a useful technique in C programming, as it allows you to access the elements of an array without using array indexing notation.
C to print the elements of an array using an integer pointer:
#include
int main() {
int arr[] = {1, 2, 3, 4, 5};
int n = sizeof(arr)/sizeof(arr[0]);
int *ptr = arr;
for (int i = 0; i < n; i++) {
printf("%d ", *(ptr + i));
}
return 0;
}
In this program, we declare an integer array arr and its size n. We then create a pointer ptr that points to the first element of arr. Finally, we use a for loop to iterate through the array and print out its elements using the pointer notation *(ptr + i). This notation dereferences the pointer to get the value at the current index, and the + i part increments the pointer to point to the next element in the array.
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [[email protected]].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!