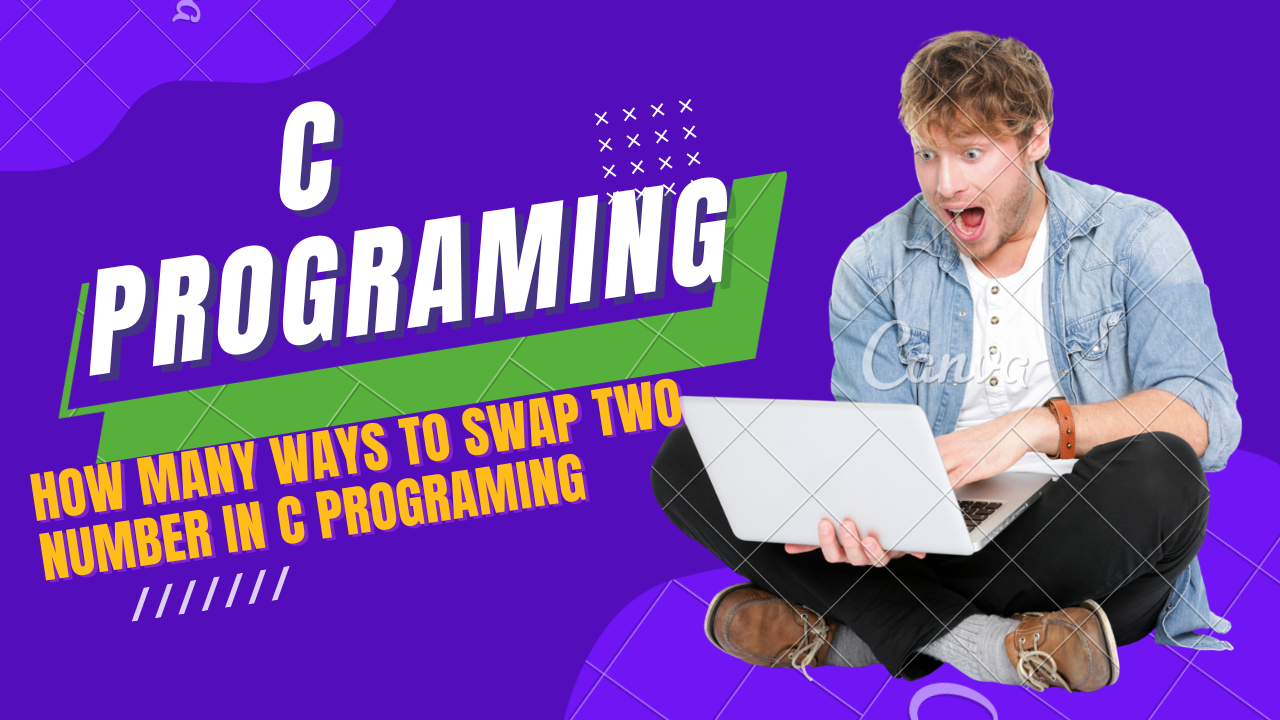
Ways to Swap Two Numbers in C: Methods and Examples
C Programming: Different Approaches for Swapping Numbers
Swapping of two numbers means exchanging the values of two variables. In other words, if there are two variables a and b with values x and y, swapping them means making a equal to y and b equal to x.
For example, if a is 3 and b is 5, after swapping a should become 5 and b should become 3. The main purpose of swapping two numbers is to rearrange the values of two variables in order to perform certain calculations or operations that require the values to be in a different order. Swapping is a fundamental operation in computer science and programming and is used in many algorithms and programs.
six-way to swap two numbers in c programming
- Using assignment operator
- Using arithmetic operator
- Using multiplication and division
- Using group operator
- Using multiplication and division in a single line
- using xor (bitwise operator)
Program 01: Swapping of two numbers using assignment Operator
#include
void main()
{
int n1,n2,temp;
printf("enter two number n1 and n2:");
scanf("%d%d",&n1,&n2);
printf("before swapping n1=%d and n2=%d\n",n1,n2);
temp=n1;
n1=n2;
n2=temp;
printf("after wapping the number n1=%d and n2=%d\n",n1,n2);
}
Enter two numbers n1 and n2:10 20
Before swapping n1=10 and n2=20
After swapping the number n1=20 and n2=10
Program 02: Swapping of two numbers using an arithmetic operator
#include
void main()
{
int n1,n2,temp;
printf("enter two number n1 and n2:");
scanf("%d%d",&n1,&n2);
printf("before swapping n1=%d and n2=%d\n",n1,n2);
n1=n1+n2;
n2=n1-n2;
n1=n1-n2;
printf("after swapping the number n1=%d and n2=%d\n",n1,n2);
}
Enter two numbers n1 and n2:20 30
Before swapping n1=20 and n2=30
After swapping the number n1=30 and n2=20
Program 03: Swapping of two numbers using multiplication and division in c,
#include
void main()
{
int n1,n2;
printf("enter two number n1 and n2:");
scanf("%d%d",&n1,&n2);
printf("before swapping n1=%d and n2=%d\n",n1,n2);
n1=n1*n2;
n2=n1/n2;
n1=n1/n2;
printf("after swapping the number n1=%d and n2=%d\n",n1,n2);
}
Enter two numbers n1 and n2:40 50
before swapping n1=40 and n2=50
after Swapping the number n1=50 and n2=40
Program 04: Swapping of two numbers Using group operator in c priogramming
#include
void main()
{
int n1,n2;
printf("enter two number n1 and n2:");
scanf("%d%d",&n1,&n2);
printf("before swapping n1=%d and n2=%d\n",n1,n2);
n2=n1+n2-(n1=n2);
printf("after wapping the number n1=%d and n2=%d\n",n1,n2);
}
Enter two numbers n1 and n2:50 60
before swapping n1=50 and n2=60
after swapping the number n1=60 and n2=50
Program 05: Swapping of two numbers Using multiplication and division in a single line in c
#include
void main()
{
int n1,n2;
printf("enter two number n1 and n2:");
scanf("%d%d",&n1,&n2);
printf("before swapping n1=%d and n2=%d\n",n1,n2);
n2=n1*n2/(n1=n2);
printf("after swapping the number n1=%d and n2=%d\n",n1,n2);
}
Enter two numbers n1 and n2:100 200
before swapping n1=100 and n2=200
after swapping the numbers n1=200 and n2=100
Program 06: Swapping of two numbers using xor (bitwise operator) in c programming
#include
void main()
{
int n1,n2;
printf("Enter two number n1 and n2:");
scanf("%d%d",&n1,&n2);
printf("before swapping n1=%d and n2=%d\n",n1,n2);
n2=n1^n2;
n1=n1^n2;
n2=n1^n2;
printf("after swapping the number n1=%d and n2=%d\n",n1,n2);
}
Enter two numbers n1 and n2:500 600
before swapping n1=500 and n2=600
after swapping the numbers n1=600 and n2=500
For further information and examples, Please visit C-Programming From Scratch to Advanced 2023-2024
Conclusion
Ultimately, the best method to use to swap two numbers in C programming language will depend on the specific needs of the program. If simplicity is important, then using a temporary variable is the best option. If performance is important, then using a function or bit manipulation may be the best option.
Awesome it's gets help