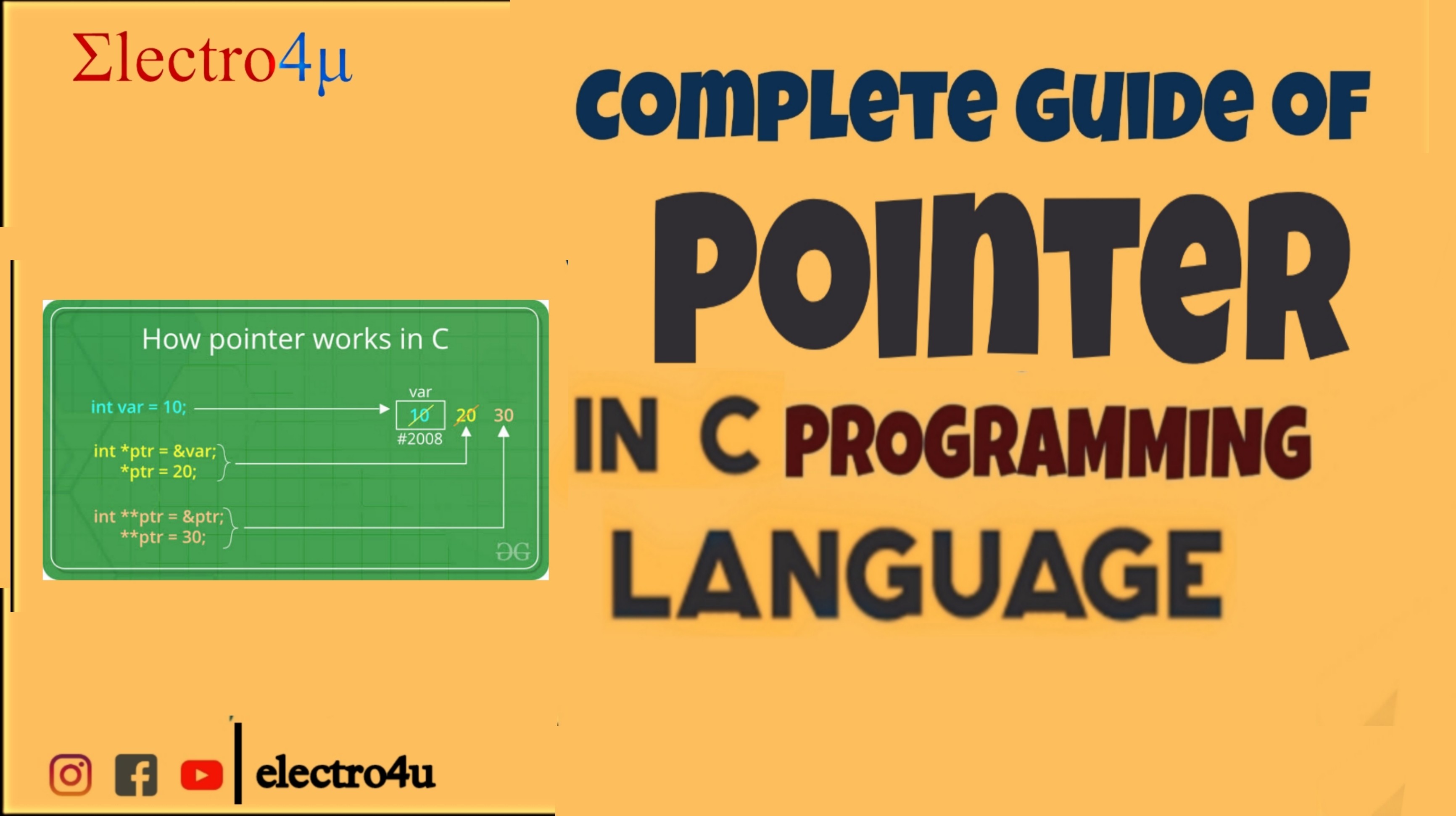
Pointer in c programming
Pointer in c programming
In C programming, a pointer is a variable that stores the memory address of another variable. Pointers allow you to access, manipulate, and manage memory dynamically, making them a powerful tool for creating efficient and complex programs.
pointer is one of the Secondary Derived Data Types which is used to hold the address of another variable through which we can excess the data in Directly
syntax for declaring a pointer in C:
data_type *pointer_variable;
Where data_type is the type of data that the pointer will point to, and pointer_variable is the name of the pointer. For example:
int *p;
This declares a pointer p that points to an int type.
You can get the memory address of a variable using the & operator and you can access the value stored at a memory address using the * operator.
For example:
int x = 10;
int *p;
p = &x;
printf("Value of x: %d\n", x);
printf("Address of x: %p\n", &x);
printf("Value stored at address p: %d\n", *p);
printf("Address stored in p: %p\n", p);
Output
Value of x: 10
Address of x: 0x7ffc5813b51c
Value stored at address p: 10
Address stored in p: 0x7ffc5813b51c
Pointers can also be used to dynamically allocate memory using the malloc function, and they are often used in combination with arrays and structures.
It's important to understand the basics of pointers before using them in your programs, as they can be both powerful and dangerous. Incorrect use of pointers can lead to memory leaks, crashes, and security vulnerabilities.
Note: If we do not Assign The value of pointer .ponter by default its's hold Garbage address is also known as wild pointer
Pointer initialization in c
In C, pointer initialization is the process of assigning a memory address to a pointer variable. This is done using the address-of operator & in combination with a variable name. For example:
int x = 5;
int *ptr = &x;
In this example, the integer variable x is assigned the value 5, and a pointer ptr is initialized to point to x by using the address-of operator &x.
It is important to initialize pointers correctly, as uninitialized pointers can lead to undefined behavior and potential security vulnerabilities in your program. Additionally, in C, it's generally recommended to set pointers to NULL after they are declared, to indicate that they don't currently point to any valid memory location.
For example:
int *ptr = NULL;
This way, if you attempt to dereference the pointer before initializing it to a valid memory address, your program will crash with a null pointer dereference error, alerting you to the problem.
The program to understand the size of a pointer is fixed
The size of a pointer is fixed on a given system and does not change dynamically. The size of a pointer is determined by the architecture of the computer and the operating system. For example, on a 32-bit system, a pointer is typically 4 bytes in size, while on a 64-bit system, a pointer is typically 8 bytes in size.
Here's an example in the C programming language that demonstrates this concept:
#include
int main()
{
int a = 10;
int *ptr_a = &a;
printf("Size of int: %ld bytes\n", sizeof(int));
printf("Size of int pointer: %ld bytes\n", sizeof(ptr_a));
return 0;
}
The output of this program on a 32-bit system would be:
Size of int: 4 bytes
Size of int pointer: 4 bytes
And on a 64-bit system, the output would be:
Size of int: 4 bytes
Size of int pointer: 8 bytes
As you can see, the size of the int variable is 4 bytes on both 32-bit and 64-bit systems, but the size of the pointer to an int variable is different, depending on the system
Top Resources
Constant Pointer in programing language
Null Pointer in c programming language
Increment and Decrement on Pointer Variable in c
Pointer Arithmetic in c
Difference Between Integers pointer and Character pointer
Prove That We are working on Little Endian and Big Endian Using Character Pointer
C Program to Apply Subtraction on Pointer
C program to appling subtraction on pointer
C program to To print the Binary format of float using a Character Pointer
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!