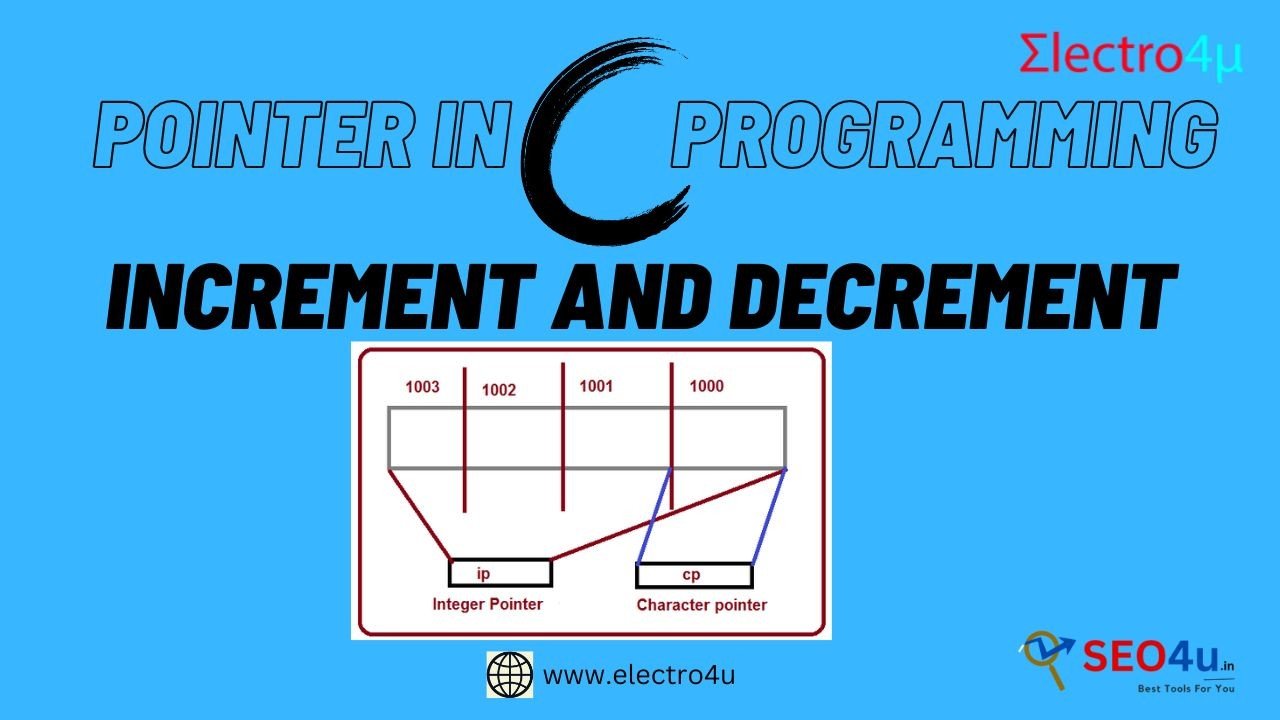
Increment and decrement on pointer variable in c
Pointer Variable Increment and Decrement in C: Explained
Incrementing and decrementing a pointer variable in C moves the pointer to the next or previous memory location, respectively. The amount by which the pointer is moved depends on the size of the data type that the pointer points to. For example, incrementing a pointer to an integer will move the pointer by 4 bytes, while incrementing a pointer to a float will move the pointer by 8 bytes.
To increment a pointer variable, we use the ++
operator. To decrement a pointer variable, we use the --
operator.
Code shows how to increment and decrement a pointer variable:
int main() {
int arr[10] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
int* ptr = arr;
printf("The value of the element at the pointer is %d\n", *ptr);
// Increment the pointer to the next element in the array.
ptr++;
printf("The value of the element at the pointer is %d\n", *ptr);
// Decrement the pointer to the previous element in the array.
ptr--;
printf("The value of the element at the pointer is %d\n", *ptr);
return 0;
}
Output:
The value of the element at the pointer is 1
The value of the element at the pointer is 2
The value of the element at the pointer is 1
Incrementing and decrementing pointer variables is a powerful technique that can be used to access and manipulate arrays and other data structures in C. However, it is important to use this technique carefully, as it can be easy to make mistakes that can lead to program crashes.
Some important things to keep in mind when incrementing and decrementing pointer variables:
- Make sure that the pointer is pointing to a valid memory location before incrementing or decrementing it.
- Do not increment or decrement a pointer beyond the bounds of the array or other data structure that it is pointing to.
- Be careful when using pointer arithmetic to calculate the address of an element in an array or other data structure.
If you are unsure whether it is safe to increment or decrement a pointer variable, it is always best to err on the side of caution and avoid doing so.