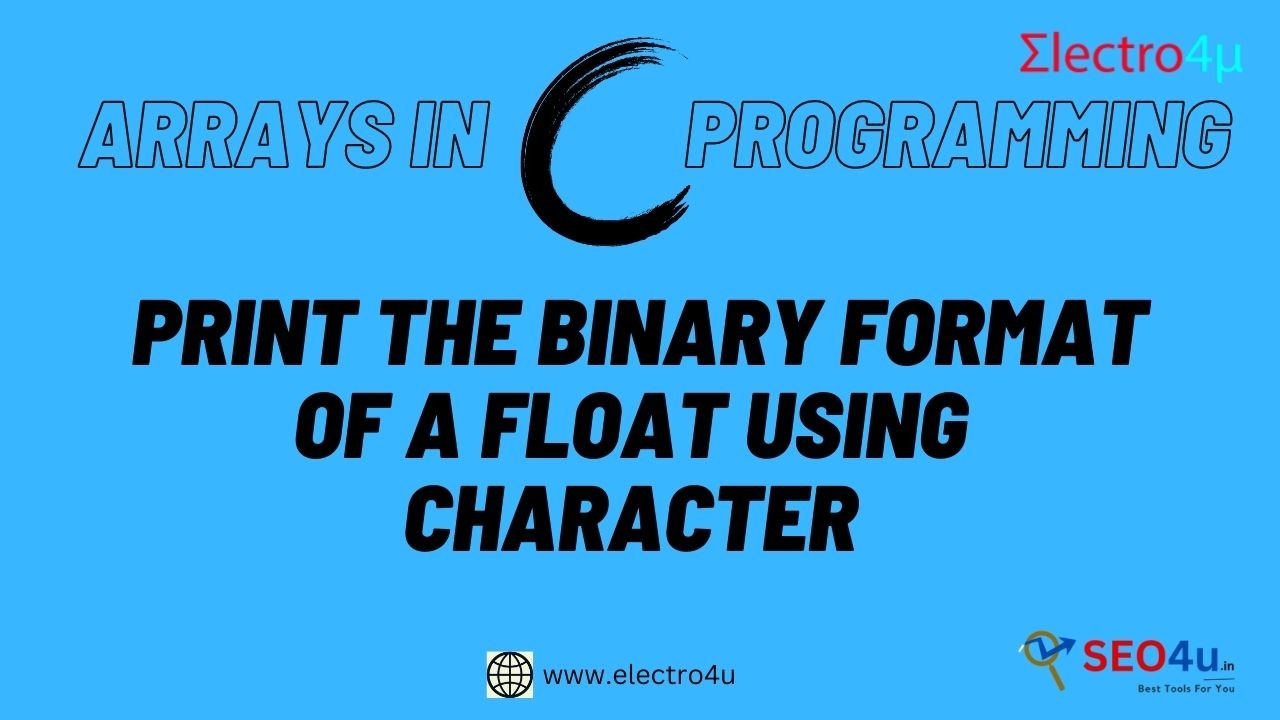
Writing a c program to print the binary format of float using character format
C program to print the binary format of a float using character
To write a C program to print the binary format of float using character format, we can use the following steps:
- Define a union to store the float and character representation of the float value.
- Assign the float value to the float member of the union.
- Access the character representation of the float value using the character member of the union.
- Print the character representation of the float value to the console.
Code shows how to implement the above steps:
C programming
#include<stdio.h>
typedef union {
float f;
char c[sizeof(float)];
} FloatCharUnion;
int main() {
float f = 1.23456789f;
FloatCharUnion u;
u.f = f;
for (int i = 0; i < sizeof(float); i++) {
printf("%c", u.c[i]);
}
printf("\n");
return 0;
}
Output:
3Fh9E983
The above output is the binary representation of the float value 1.23456789f.
It is important to note that the binary representation of a float value is not guaranteed to be the same on all platforms. This is because the IEEE floating-point standard allows for some flexibility in how float values are represented in memory.
Top Resources
Copy in a reverse manner in c
Write a program to reverse printing not reversing of 10 elements of array in c
Write a program to reverse the bits of a given number using for loop
Design a function to reverse the given string
Design a function to reverse the word in given string
write a program to copy one array element to another array element in reverse manner.
write a program to reverse adjacent elements of an array.
write a program to reverse half of the elements of an array.
Further Reading:
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!