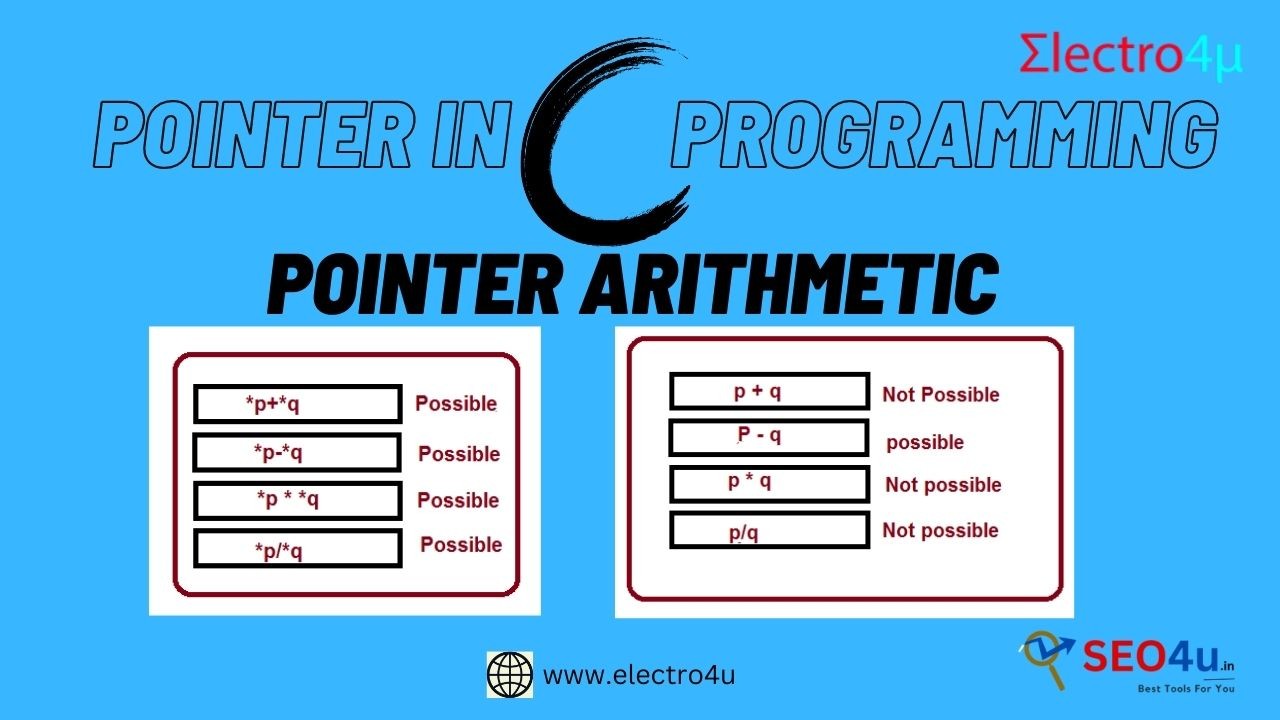
Pointer Arithmetic in c
Understanding Pointer Arithmetic in C Programming
Pointer arithmetic in C is the process of performing arithmetic operations on pointers. This can be done to move a pointer to a different memory location, or to calculate the address of a specific element in an array.
The following arithmetic operations can be performed on pointers in C:
- Increment (++)
- Decrement (--)
- Addition (+)
- Subtraction (-)
The increment and decrement operators can be used to move a pointer to the next or previous element in an array. The addition and subtraction operators can be used to calculate the address of a specific element in an array.
How to use pointer arithmetic to move a pointer to the next element in an array:
C programming
int arr[10] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
int* ptr = arr;
// Move the pointer to the next element in the array.
ptr++;
// Print the value of the element at the current pointer location.
printf("%d\n", *ptr);
output:
2
How to use pointer arithmetic to calculate the address of a specific element in an array:
C programming
int arr[10] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
int* ptr = arr + 5;
// Print the address of the element at the current pointer location.
printf("%p\n", ptr);
Output
0x7ffe7004
Pointer arithmetic can be a powerful tool for manipulating arrays in C. However, it is important to use it carefully, as it can lead to undefined behavior if it is not used correctly.
Note: please not that the possible condition in diagram
Some important things to keep in mind when using pointer arithmetic in C:
- Always make sure that the pointer is within the bounds of the array before performing any arithmetic operations.
- Avoid performing pointer arithmetic on a null pointer.
- Avoid casting pointers to different data types.
If you are unsure whether it is safe to perform a pointer arithmetic operation, it is always best to err on the side of caution and avoid doing so.