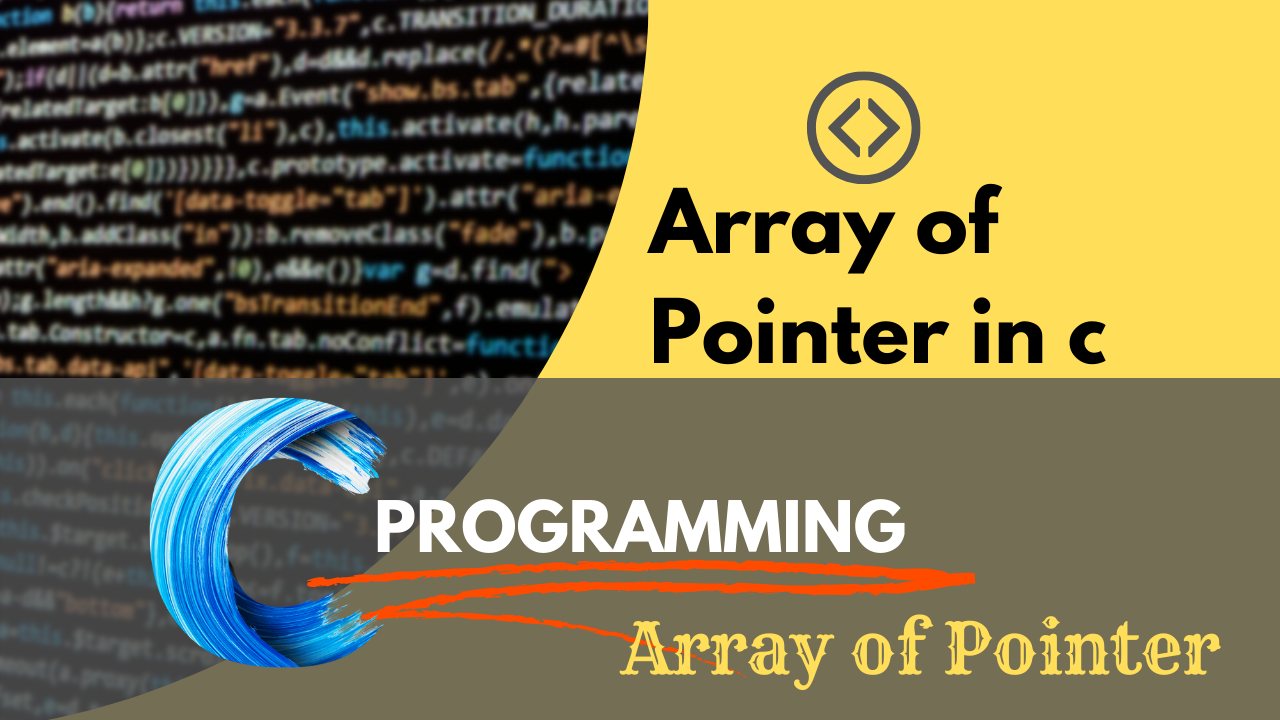
Array of Pointer in c programming language
What is an array of pointers in c
An array of pointers in C is an array that holds a sequence of memory addresses (pointers) pointing to other data types. Each element in the array holds the address of a specific memory location where the actual data is stored.
In C programming language, an array of pointers is a data structure that stores multiple pointers to data elements of the same type in contiguous memory locations.
array of pointers syntax:
where datatype is the type of data the pointers will point to, variable_name is the name of the array, and ele is the number of elements in the array.
For example 01, to declare an array of 5 pointers to integers, you would use:
C Programming
int *p[ 5 ]
p is an array of 5 Integers Pointers
You can then assign each pointer in the array to a specific memory location using the & operator or by allocating memory dynamically using the malloc() function.
For example, to assign the first pointer in the array to the address of an integer variable num, you would use:
C Programming
int num = 10; ptr_array[0] = #
You can access the value of the integer variable through the pointer in the array using the * operator.
For example, to print the value of the integer variable using the first pointer in the array, you would use:
C Programming
printf("%d\n", *ptr_array[0]);
This would output 10, the value of the integer variable num.
Example 02: write a c program to find the size of the array of pointer
C Programming
#include<stdio.h>
void main()
{
int *p[5];
printf("Sizeof Array of Pointer:%ld\n",sizeof(p));
}
Output: Size of Array of Pointer: 20
Example 03: write a c program to find the size of the array of 3 pointer variable
C programming
#include<stdio.h>
void main()
{
int i=10,j=20,k=30;
int *p[3];
p[0]=&i;
p[1]=&j;
p[2]=&k;
printf("Sizeof array of Pointer:%ld\n",sizeof(p));
printf(" %d %d %d\n",*p[0],*p[1],*p[2]);
}
Output: Sizeof Array of Pointer: 12
Further Reading:
What is Array of pointer? Give one example.
Array of Pointer in c programming language
How to Create An Array Of Pointers in C – A Step-by-Step Guide
What is the difference between Array of pointer and Pointer to Array?
array of pointers, string functions, data manipulation, efficient algorithms
Dynamically Allocating Memory for an Array of Pointers
Virtual Shorting Using an array of Pointer
Write a C function that takes an array of pointers to strings and sorts them in lexicographic (dictionary) order.
Assignment of Array of Pointers in C
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!