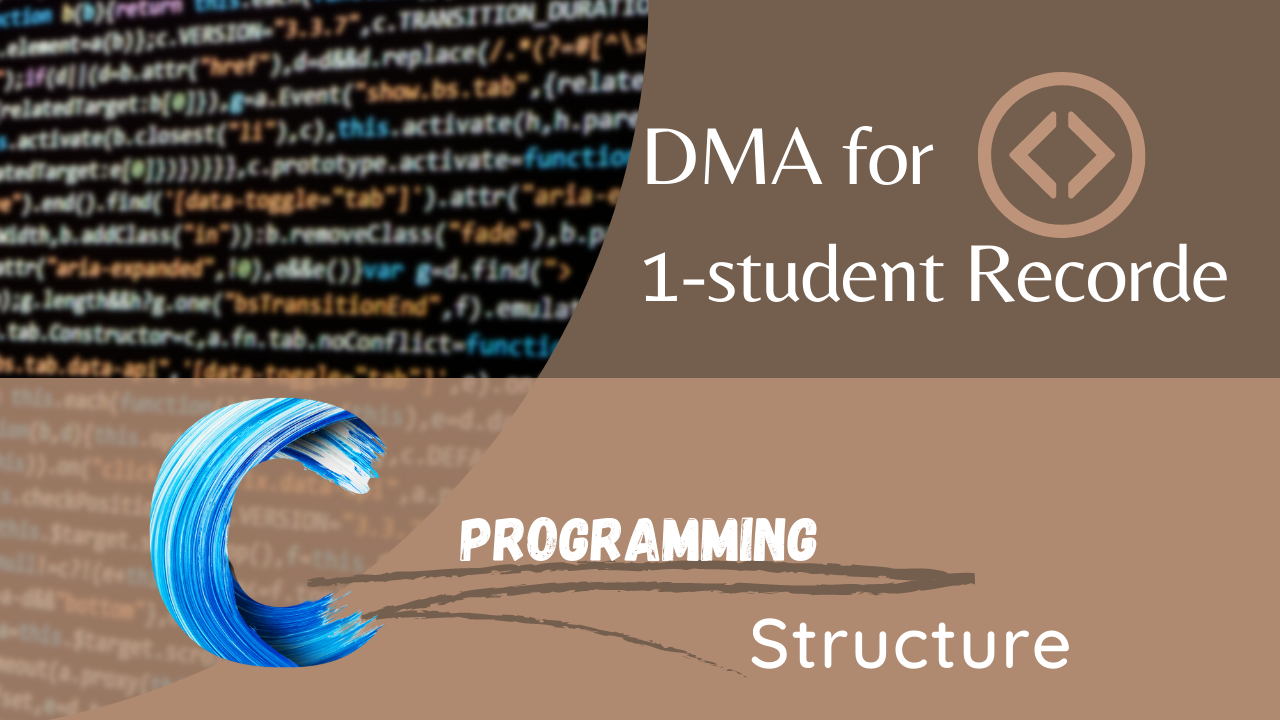
Clojure Programming Language: Understanding Syntax and Data Types
What is Clojure Programming language
Clojure is a dynamic, general-purpose programming language that builds on the Lisp tradition and runs on the Java Virtual Machine (JVM). It is a dialect of Lisp, so it shares many of its features, such as a powerful macro system and a focus on code as data. However, Clojure also has some unique features, such as its immutable data structures and its support for concurrent programming.
Basic Syntax
Clojure is a Lisp dialect, so it has a similar syntax to Lisp. Clojure code is written in parentheses, with expressions evaluated from left to right.
(+ 1 2 3) ; evaluates to 6
Clojure functions can be defined using the def keyword.
(def square (fn [x] (* x x)))
(square 3) ; evaluates to 9
Clojure also has a number of built-in functions, such as +, -, *, /, and =.
(= 1 1) ; evaluates to true
Data Types
Clojure has a number of different data types, including:
- Numbers: Clojure supports all of the primitive numeric types in Java, such as integers, floats, and doubles.
- Strings: Clojure strings are represented as sequences of characters.
- Symbols: Clojure symbols are unique identifiers that can be used to represent things like functions, variables, and classes.
- Lists: Clojure lists are ordered sequences of values.
- Vectors: Clojure vectors are immutable, fixed-size sequences of values.
- Maps: Clojure maps are associative collections of key-value pairs.
- Sets: Clojure sets are unordered collections of unique values.
Examples
Some examples of using Clojure data types:
;; Numbers
(1)
(1.23)
(100L)
;; Strings
"Hello, world!"
;; Symbols
'my-symbol
;; Lists
'(1 2 3 4)
;; Vectors
[1 2 3 4]
;; Maps
{:key "value"}
;; Sets
#{1 2 3 4}
Clojure is a powerful and expressive programming language that is well-suited for a variety of tasks. Its simple syntax and rich data types make it a good choice for beginners and experienced programmers alike.
Enroll Now:
[ Course in Production] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!