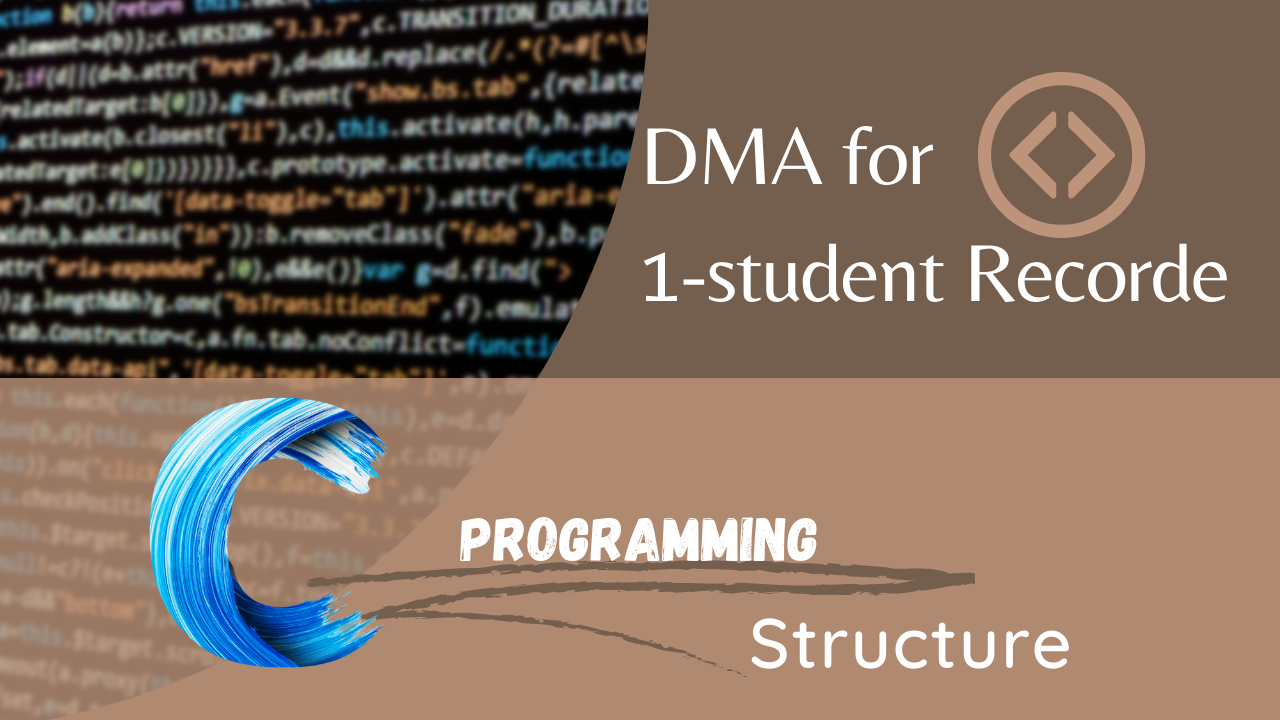
Design a function to allocate dynmaic memory for 1 student record in c
Dynamic Memory Allocation for Student Record in C
To design a function to allocate dynamic memory for 1 student record, we can use the following steps:
- Define a struct to store the student record. The struct should contain all of the necessary information for the student, such as their name, age, and grade.
- Allocate memory for the student record. We can use the malloc() function to allocate memory for the struct.
- Initialize the student record. We can set the values of the struct members to the student's information.
- Return the pointer to the student record. We can return the pointer to the struct that was allocated in step 2.
Example of a function to allocate dynamic memory for 1 student record in C:
#include <stdlib.h>
typedef struct student {
char name[100];
int age;
int grade;
} Student;
Student* allocate_student_record() {
// Allocate memory for the student record.
Student* student = (Student*)malloc(sizeof(Student));
if (student == NULL) {
// If memory could not be allocated, return NULL.
return NULL;
}
// Initialize the student record.
student->name[0] = '\0';
student->age = 0;
student->grade = 0;
// Return the pointer to the student record.
return student;
}
To use the allocate_student_record()
function, we can simply call it and store the result in a pointer variable. For example:
Student* student = allocate_student_record();
This will allocate dynamic memory for a new student record and return a pointer to the record. We can then use the pointer to access the student's information.
Once we are finished with the student record, we should free the memory that was allocated for it. We can do this using the free()
function. For example:
free(student);
This will free the memory that was used to store the student record.
It is important to note that we should always free memory that was allocated using malloc()
. If we do not free the memory, it will be leaked and will not be available for other programs to use.
Top Resources
what is structure in C?
What is self-referential structure pointer in c?
What is structure padding? How to avoid structure padding?
differences between a structure and a union in c?
How can typedef be to define a type of structure in c?
Program to illustrate a function that assigns value to the structure
What is structure? What are differences between Arrays and Structures?
Write a program to find the sizeof structure without using sizeof operator?
Write a program that returns 3 numbers from a function using a structure in c.
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [[email protected]].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!