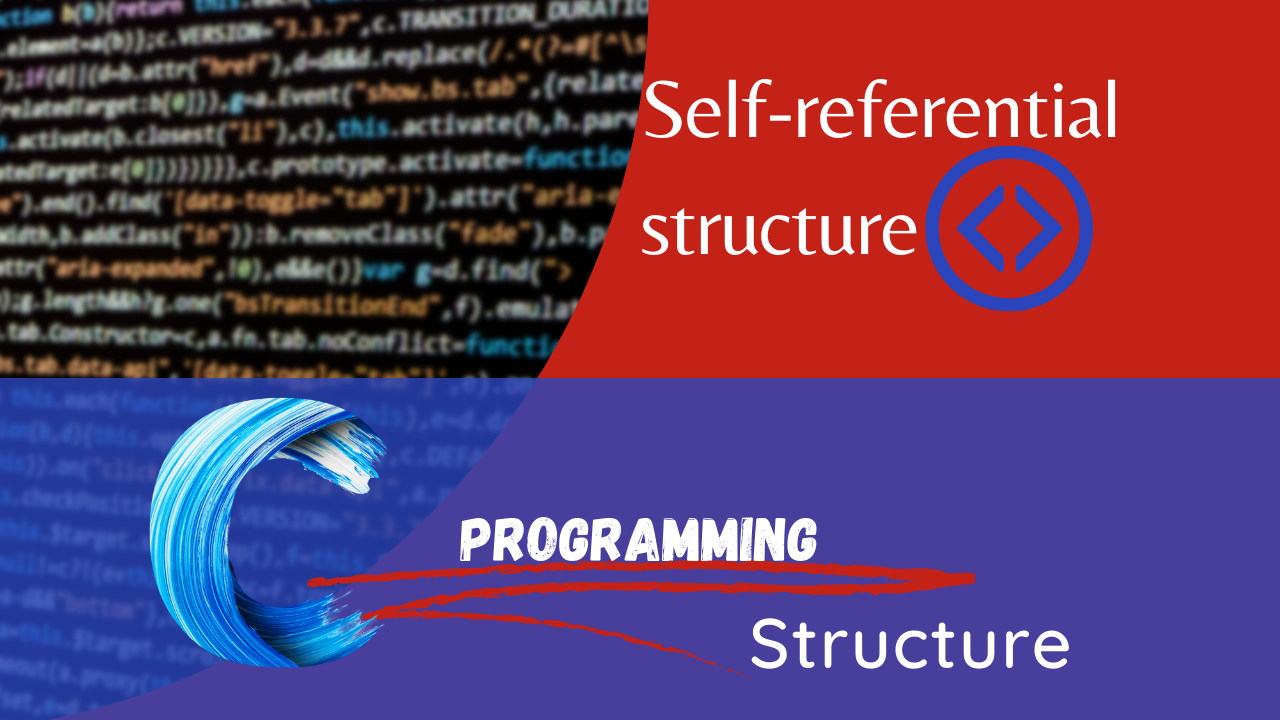
Self referential structure in c programming
Exploring Self-Referential Structures in C Programming
A self-referential structure in C is a structure that contains a pointer to itself. This allows the structure to refer to itself, creating a linked data structure. Self-referential structures are a powerful tool for creating complex data structures in C++ and are commonly used in algorithms such as trees, graphs, and linked lists.
Here is an example of a self-referential structure in C:
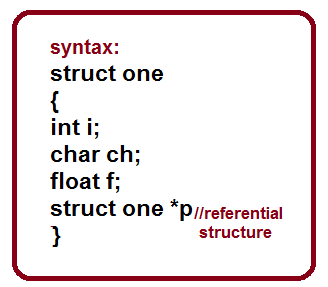
To create a linked list, we can use the following code:
Node *head = NULL;
// Create a new node
Node *newNode = malloc(sizeof(Node));
newNode->data = 10;
newNode->next = NULL;
// Add the new node to the linked list
head = newNode;
We can then add more nodes to the linked list by using the next pointer to connect them together. For example:
// Create another new node
Node *newNode2 = malloc(sizeof(Node));
newNode2->data = 20;
newNode2->next = NULL;
// Connect the first and second nodes
head->next = newNode2;
To print the linked list, we can use the following code:
Node *current = head;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
This code will print the following output:
10 20
Self-referential structures can be used to create a variety of complex data structures, such as trees, graphs, and stacks. They are a powerful tool for C programmers and are used in many popular algorithms.
Top Resources
what is structure in C?
What is self-referential structure pointer in c?
What is structure padding? How to avoid structure padding?
differences between a structure and a union in c?
How can typedef be to define a type of structure in c?
Program to illustrate a function that assigns value to the structure
What is structure? What are differences between Arrays and Structures?
Write a program to find the sizeof structure without using sizeof operator?
Write a program that returns 3 numbers from a function using a structure in c.
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!