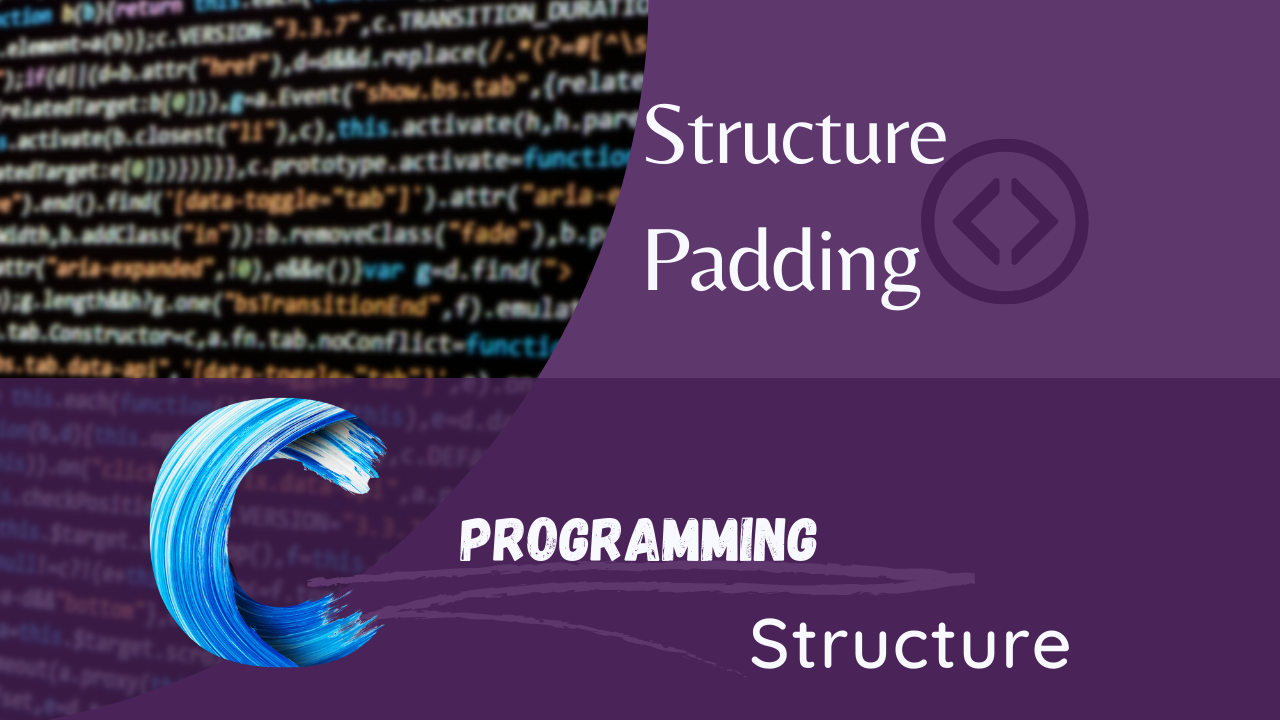
Structure Padding in c
What is Structure Padding?
structure padding is compiler dependent process, some times in structure variables more than the required memory allocated that extra memory allocated is called as structure padding. that additional unused byte that is allocated is called a hole in a structure.
In C programming, structure padding refers to the process of adding extra bytes of memory to a structure in order to align its elements to certain memory boundaries.
This is necessary because modern computer architectures are designed to be more efficient when accessing data that is aligned to specific byte boundaries, such as multiples of 2, 4, or 8
bytes. Without proper alignment, accessing data can become slower and less efficient.
When a structure is declared, the compiler will automatically insert padding bytes between its elements to ensure proper alignment. The amount of padding added depends on the size and alignment requirements of each element.
Note
- This structure padding is compiler dependent process
- the compiler will decide the size of a variable, os
- os will be reserved the memory for variables
- the process will process that variable
- When the compiler informing to the os to remove some memory for os it will remove
What are the points to take care of?
- The variables which required 1 byte of the memory address should be multiple of 1 byte
- The variables which required a 2-byte memory address should be multiple of 2-byte
- the variables which required 1-byte and more than 4-byte. the memory address should be a multiple of 4 bytes
- so that every time no need to calculate offset, os that processing the variable becomes faster
How to avoid structure padding in c?
if you want to avoid structure padding up to a certain we need to put a similar type of data type or use #pragma pack(1)
For example, 01, consider the following structure:
struct Example {
char c;
int i;
double d;
};
In this case, the char element has a size of 1 byte and requires no padding, the int element has a size of 4 bytes and should be aligned on a 4-byte boundary, and the double element has a size of 8 bytes and should be aligned on an 8-byte boundary.
To achieve proper alignment, the compiler may add 3 bytes of padding after the char element, and 4 bytes of padding after the int element, resulting in a total size of 24 bytes:
struct Example {
char c; // 1 byte
char padding[3]; // 3 bytes padding
int i; // 4 bytes
double d; // 8 bytes
};
It's important to note that structure padding can vary between different compilers and platforms, so it's generally not recommended to rely on the exact size and layout of a structure. Instead, it's best to use standard C techniques like sizeof and offsetof to work with structures in a portable and reliable way.
Program 01: C program to structure padding
#include<stdio.h>
#pragma pack(1)
struct one
{
int i;
char ch;
float f;
};
void main()
{
struct one s1;
printf("Total size of structure: %ld\n",sizeof(s1));
printf("%ld %ld %ld\n",sizeof(s1.i),sizeof(s1.ch),sizeof(s1.f));
// printf("%u %u %u\n",&i,&ch,&f);
}
Output:
The total size of the structure: 9
4 1 4
Advantages of structure padding in c
The primary advantage of structure padding in C is that it improves the performance of the program by ensuring that data elements are properly aligned in memory. This is because most computer architectures are designed to access data in aligned memory locations, which can result in faster and more efficient data access.
- Improved memory access performance
- Efficient use of memory
- Platform independence
- Better optimization
Disadvantages of structure padding in c
There are also some disadvantages to consider to structure
- Waste of memory
- Portability issues
- Difficulty in memory management
- Difficult to debug
- Potential security risks
Top Resources
what is structure in C?
What is self-referential structure pointer in c?
What is structure padding? How to avoid structure padding?
differences between a structure and a union in c?
How can typedef be to define a type of structure in c?
Program to illustrate a function that assigns value to the structure
What is structure? What are differences between Arrays and Structures?
Write a program to find the sizeof structure without using sizeof operator?
Write a program that returns 3 numbers from a function using a structure in c.
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!