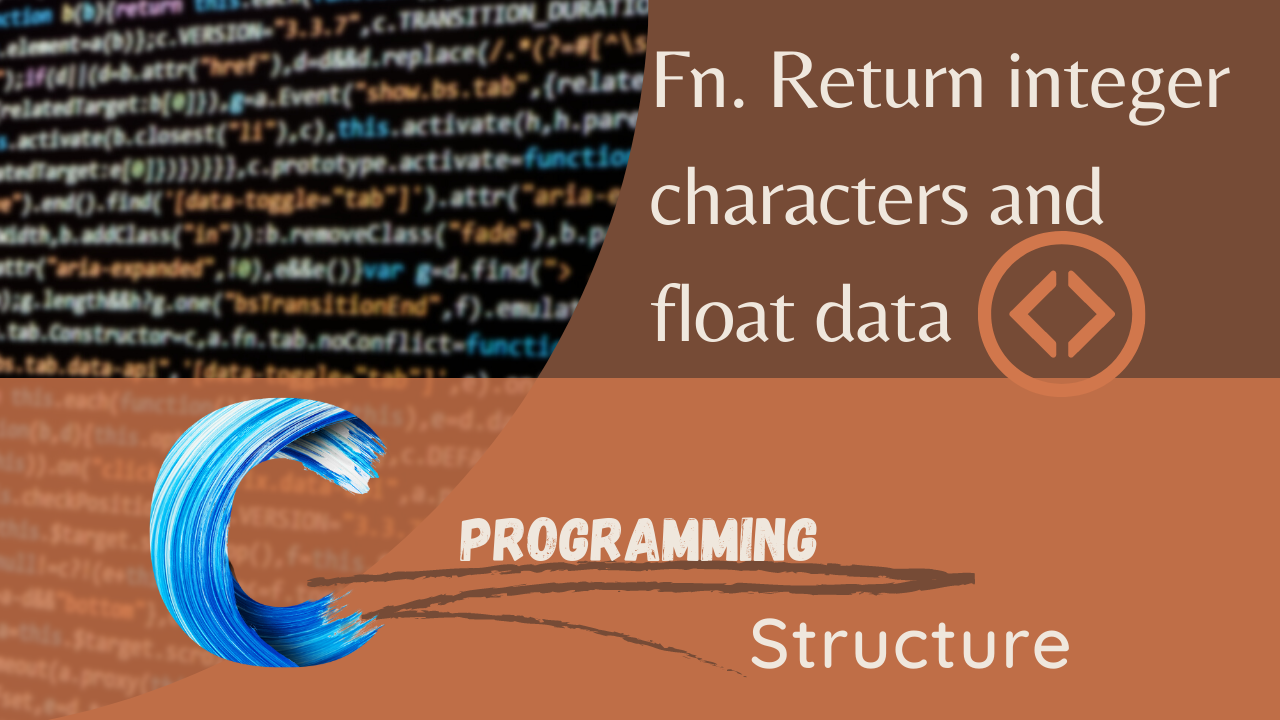
Design a function that should return integer characters and float data
C Programming: Creating a Function for Integer and Float Character Retrieval Using Structures
To design a function that should return integer characters and float data in C using structure, we can use the following steps:
- Define a structure to store the integer characters and float data.
- Declare a function that takes the structure as a parameter and returns it.
- Inside the function, assign the integer characters and float data to the corresponding fields in the structure.
- Return the structure from the function.
Function that returns integer characters and float data in C using structure:
C Programming
#include <stdio.h>
typedef struct {
int integer_characters;
float float_data;
} Data;
Data get_data() {
Data data;
// Assign the integer characters and float data to the corresponding fields in the structure.
data.integer_characters = 'A';
data.float_data = 3.14159;
// Return the structure from the function.
return data;
}
int main() {
// Call the function to get the data.
Data data = get_data();
// Print the integer characters and float data.
printf("Integer characters: %d\n", data.integer_characters);
printf("Float data: %f\n", data.float_data);
return 0;
}
Output:
Integer characters: 65
Float data: 3.141590
Top Resources
what is structure in C?
What is self-referential structure pointer in c?
What is structure padding? How to avoid structure padding?
differences between a structure and a union in c?
How can typedef be to define a type of structure in c?
Program to illustrate a function that assigns value to the structure
What is structure? What are differences between Arrays and Structures?
Write a program to find the sizeof structure without using sizeof operator?
Write a program that returns 3 numbers from a function using a structure in c.
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [[email protected]].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!