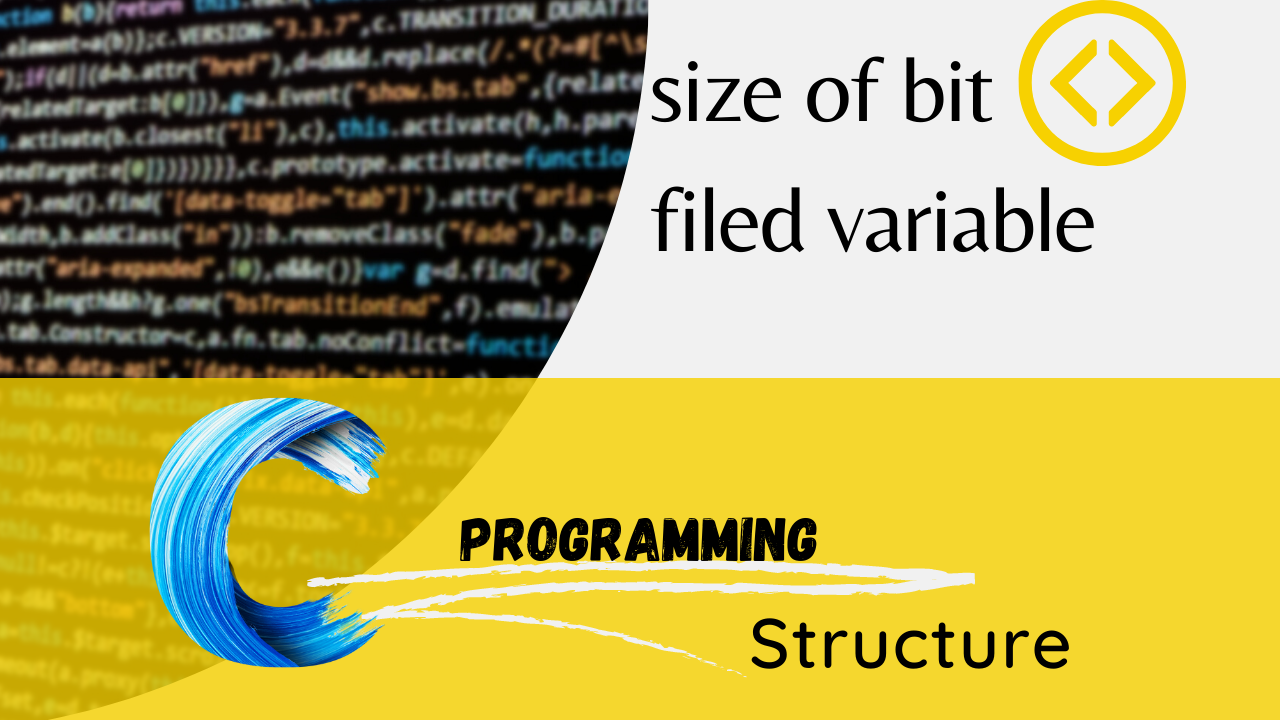
Write a c program for size of bit filed variable
C Program: Size of Bit Field Variables
To write a C program for the size of a bit-field variable, we can use the following steps:
- Include the necessary header files.
- Declare a bit-field variable.
- Use the sizeof() operator to get the size of the bit-field variable.
- Print the size of the bit-field variable to the console.
C program for the size of a bit-field variable:
C Programming
#include <stdio.h>
struct {
unsigned int a : 3;
unsigned int b : 5;
} bit_field;
int main() {
int size = sizeof(bit_field);
printf("The size of the bit-field variable is %d bytes.\n", size);
return 0;
}
Output:
The size of the bit-field variable is 1 byte.
As we can see from the output, the size of the bit-field variable is 1 byte, even though the bit-field variable has 8 bits. This is because the bit-field variable is packed into a single byte.
We can also use the sizeof() operator to get the size of a single bit-field member.
For example, the following code shows how to get the size of the a bit-field member:
C Programming
int size_a = sizeof(bit_field.a);
printf("The size of the bit-field member `a` is %d bits.\n", size_a);
Output:
The size of the bit-field member `a` is 3 bits.
We can use this information to optimize our code by using bit-field variables to store data that does not need to be stored in a full byte.
Top Resources
what is structure in C?
What is self-referential structure pointer in c?
What is structure padding? How to avoid structure padding?
differences between a structure and a union in c?
How can typedef be to define a type of structure in c?
Program to illustrate a function that assigns value to the structure
What is structure? What are differences between Arrays and Structures?
Write a program to find the sizeof structure without using sizeof operator?
Write a program that returns 3 numbers from a function using a structure in c.
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.