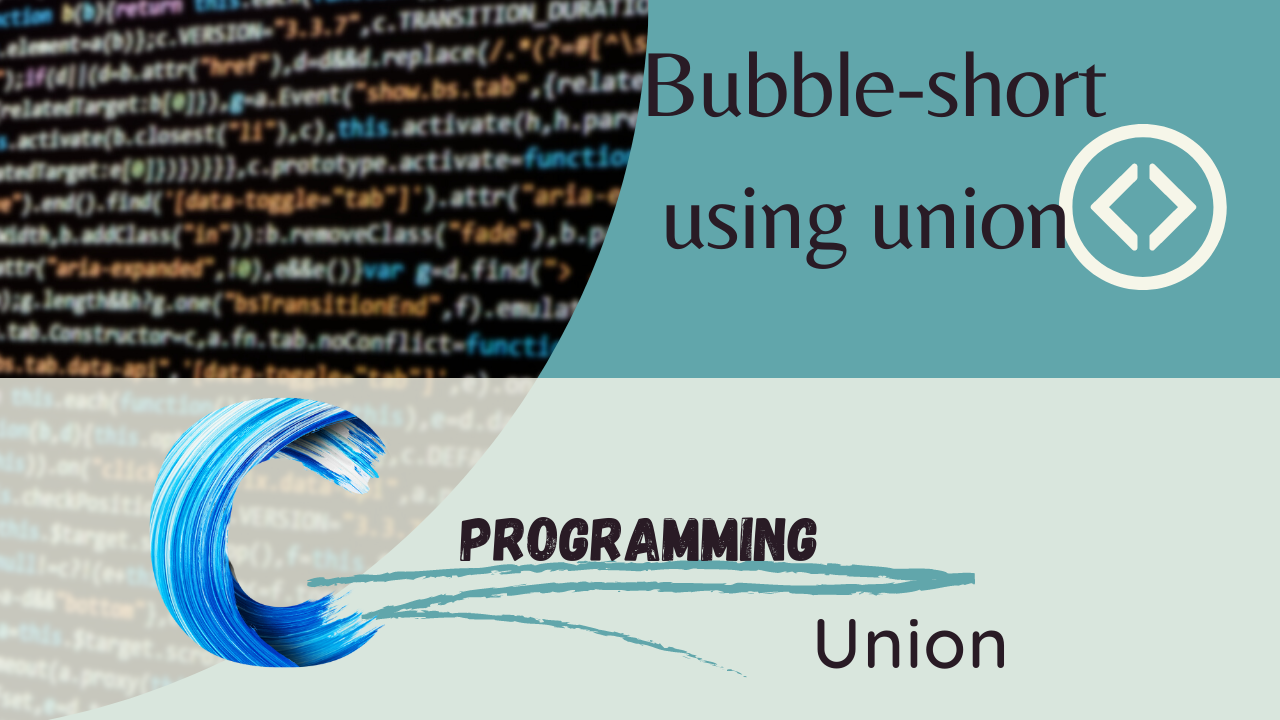
Design a function for shorting the integer of array elements using union - bubble sort using union in c
C Programming: Sorting Integers with Union and Bubble Sort
"Bubble sort using union in C"
means creating a function in the C programming language that uses the bubble sort algorithm to sort an array of integer elements. In this implementation, we will use a union data type to store the integers to be sorted. A union is a special type of C structure that can hold different data types in the same memory location.
The bubble sort algorithm works by repeatedly swapping adjacent elements in the array if they are in the wrong order until the array is sorted in ascending order. The union data type will allow us to swap elements in the array without needing to create a temporary variable to hold the value during the swap.
Overall, the task is to implement a function that takes an array of integer elements, sorts them using the bubble sort algorithm, and returns the sorted array using a union in C.
Implementation of bubble sort using a union in C
#include <stdio.h>
#include <stdbool.h>
union Number {
int i;
float f;
};
void bubble_sort(union Number arr[], int n) {
for (int i = 0; i < n - 1; i++) {
bool swapped = false;
for (int j = 0; j < n - i - 1; j++) {
if (arr[j].i > arr[j + 1].i) {
union Number temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
swapped = true;
}
}
if (!swapped) {
// If no swap occurred, the array is already sorted
break;
}
}
}
int main() {
union Number arr[] = {{.i = 10}, {.i = 5}, {.i = 7}, {.i = 3}, {.i = 2}, {.i = 8}, {.i = 6}, {.i = 1}, {.i = 4}, {.i = 9}};
int n = sizeof(arr) / sizeof(arr[0]);
bubble_sort(arr, n);
for (int i = 0; i < n; i++) {
printf("%d ", arr[i].i);
}
printf("\n");
return 0;
}
output
1 2 3 4 5 6 7 8 9 10
In this implementation, we use a union called Number to hold either an integer
or a float. Since we only need to sort integers in this case, we can use the integer field of the union. The bubble_sort the
function takes an array of Number
objects and an integer n
representing the size of the array.
The function uses the bubble sort algorithm to sort the array in ascending order. The swapped
variable is used to keep track of whether a swap occurred during each iteration of the outer loop. If no swaps occur during an iteration, the array is already sorted, and the function breaks out of the outer loop.
The sorted array is then printed using a loop in the main
function.
Top Resources
Design a function for bubble sort in c
bubble sort using union in c
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!