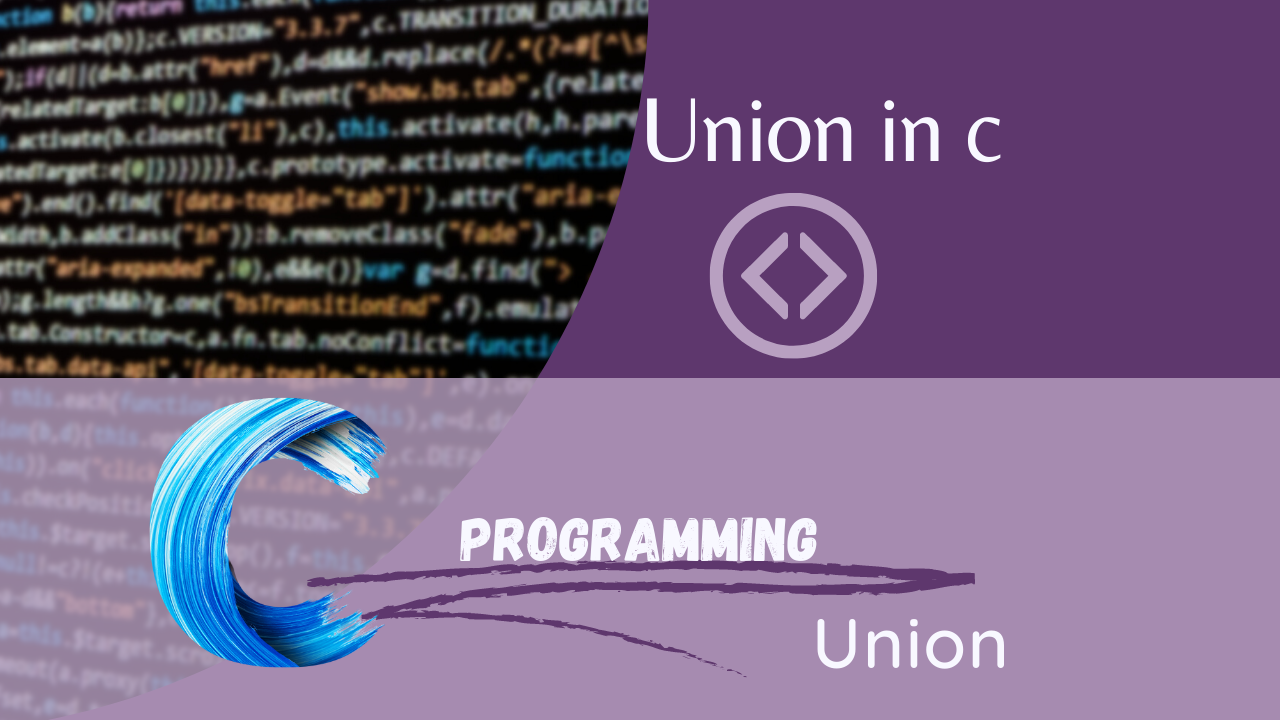
Union in c programming
What is Union
A union in C is a user-defined
data type that allows you to store different data types in the same memory location. Unions are useful for storing data that is used in different ways, such as a variable that is used as a flag in one part of the program and as a counter in another part of the program.
To define a union, you use the union keyword followed by the name of the union and a list of data types that the union can store. For example, the following code defines a union called Number that can store either an integer or a float value:
union Number {
int i;
float f;
};
To declare a variable of the Number union type, you use the following syntax:
union Number num;
You can then assign a value to the num variable using the .i or .f member selector, depending on the type of data you want to store.
For example, the following code assigns the integer value 10 to the num variable:
num.i = 10;
You can then access the value of the num variable using the .i or .f member selector, depending on the type of data you want to retrieve. For example, the following code prints the integer value 10 to the console:
printf("%d\n", num.i);
Unions are a powerful feature of C that can be used to save memory and write more efficient code. However, it is important to use unions carefully, as they can be confusing if used incorrectly.
Some examples of how unions can be used in C:
- To store a flag and a counter in the same memory location.
- To store a pointer to different types of data.
- To store a data structure that is used in different ways.
- To save memory by storing different types of data in the same memory location.
Some tips for using unions in C:
- Be careful not to overwrite the data in a union.
- Make sure to use the correct member selector to access the data in a union.
- Initialize a union before using it.
- Use unions sparingly, as they can be confusing if used incorrectly.
Example 01 of a union in C:
#include<stdio.h>
union u
{
int i;
char ch;
float f;
};
void main()
{
union u u1;
printf("%u %u %u \n",&u1.i,&u1.ch,&u1.f);
}
Output: 2293468 2293468 2293468
Conclusion:
In Diagram
will see the representation as you when we print the address of integer, character, and float all three data have the same address which means the union has the same memory location.
Top Resources
Union in c
Printing The Binary formate of float using union
Nested union in C
Assignment in union in c programming
What is a union? What are the advantages & Disadvantages in c?
What are differences between Structure and Union in c?
advantages of union in c
bubble sort using union in c
Write a C Program to prove we are working on little Endian environment using a union
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!