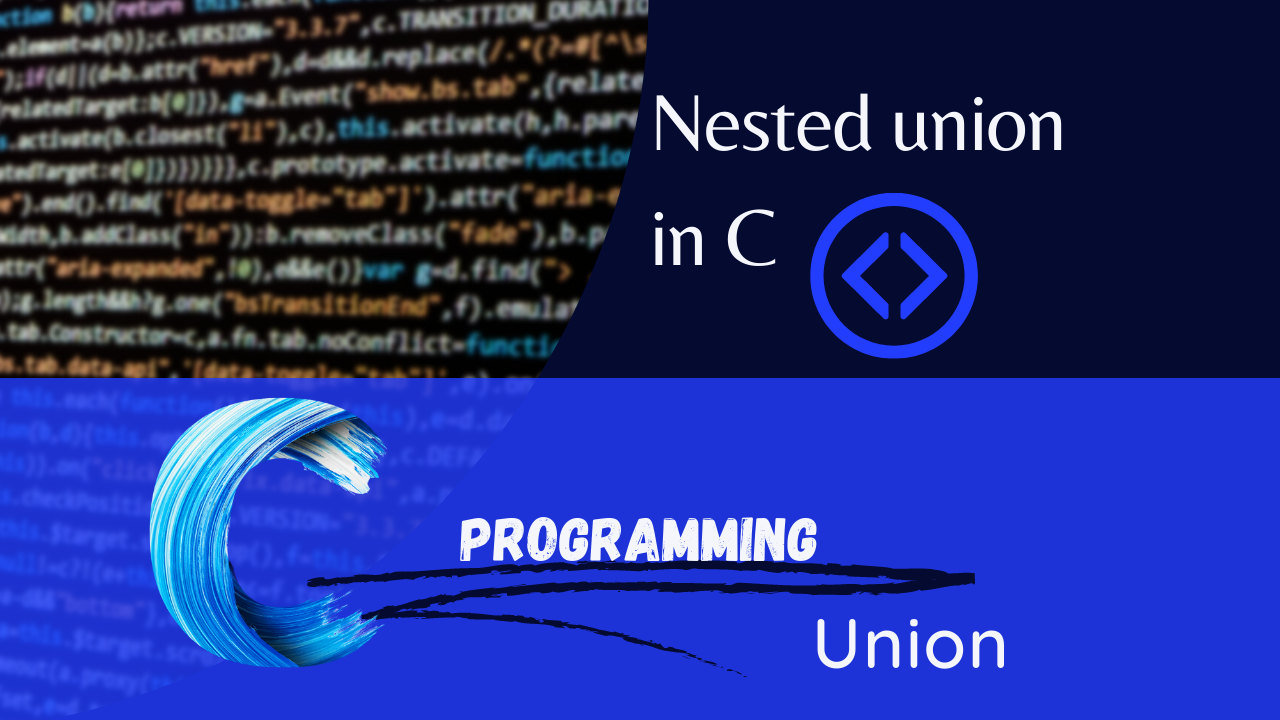
Nested union in C
Mastering Nested Union Declarations in C
A nested union in C is a union that contains another union as a member. This can be useful for grouping together related data types, or for creating complex data structures.
For example, the following code defines a nested union to represent an employee's contact information:
union contact_info {
char phone_number[10];
char email_address[255];
};
union employee {
int employee_id;
char name[255];
contact_info contact;
};
This nested union allows us to store an employee's phone number or email address, depending on which one is available. To access the contact information for a particular employee, we can use the following code:
union employee employee1;
employee1.employee_id = 12345;
strcpy(employee1.name, "John Doe");
strcpy(employee1.contact.phone_number, "+1 (555) 555-5555");
// Print the employee's phone number
printf("Employee %d's phone number is %s\n", employee1.employee_id, employee1.contact.phone_number);
Nested unions can also be used to create more complex data structures. For example, the following code defines a nested union to represent a linked list:
union node {
int data;
union node *next;
};
// Create a new node
union node *create_node(int data) {
union node *node = malloc(sizeof(union node));
node->data = data;
node->next = NULL;
return node;
}
// Add a new node to the beginning of a linked list
union node *add_node_to_front(union node *head, int data) {
union node *new_node = create_node(data);
new_node->next = head;
head = new_node;
return head;
}
// Print the elements of a linked list
void print_linked_list(union node *head) {
while (head != NULL) {
printf("%d ", head->data);
head = head->next;
}
printf("\n");
}
int main() {
union node *head = NULL;
// Add some nodes to the linked list
head = add_node_to_front(head, 10);
head = add_node_to_front(head, 20);
head = add_node_to_front(head, 30);
// Print the elements of the linked list
print_linked_list(head);
return 0;
}
This code shows how nested unions can be used to create complex data structures, such as linked lists, binary trees, and graphs.
Advantages of nested unions
Nested unions offer a number of advantages, including:
- Flexibility: Nested unions allow us to group together related data types, or to create complex data structures.
- Efficiency: Nested unions can be more efficient than using separate data structures for each member.
- Readability: Nested unions can make our code more readable and maintainable.
Disadvantages of nested unions
Nested unions also have some disadvantages, including:
- Complexity: Nested unions can be complex to understand and use.
- Error-prone: It is easy to make mistakes when using nested unions, which can lead to errors.
Overall, nested unions are a powerful tool that can be used to create complex and efficient data structures. However, it is important to use them carefully to avoid errors.
Top Resources
Union in c
Nested union in C
Advantages of union in c
Differences between a structure and a union in c?
What is a union? What are the advantages & Disadvantages in c?
What are differences between Structure and Union in c?
printing the binary of any integer number using union in c | electro4u
printing the Binary Format of any Floating number using union
write a program to prove that we are working on little Endian or big Endian using union
Design a function for shorting the integer of array elements using union - bubble sort using union in c
Write a Program to prove we are working on little Endian environment using a union
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!